Generating a Random Number
Author |
Message |
dymd3z
|
Posted: Thu May 17, 2007 8:01 am Post subject: Generating a Random Number |
|
|
I'm trying to make a coin toss game where the user is to guess which side will land. It has to be random, so I'm using if statements and whatnot. I just need to make a certain variable a random number each time, but I don't know how. For example, I need:
var randnum : int
I need randnum to be a random number each time the program is ran, how do i do this? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Clayton

|
Posted: Thu May 17, 2007 8:12 am Post subject: RE:Generating a Random Number |
|
|
Take a look at Rand.Int(). |
|
|
|
|
 |
dymd3z
|
Posted: Thu May 17, 2007 8:31 am Post subject: RE:Generating a Random Number |
|
|
How would I integrate Rant.Int with my variable? |
|
|
|
|
 |
Clayton

|
Posted: Thu May 17, 2007 9:06 am Post subject: RE:Generating a Random Number |
|
|
Have you looked at the Turing Documentation yet? Just giving you the answer doesn't help you. If you have, forgive me. |
|
|
|
|
 |
dymd3z
|
Posted: Thu May 17, 2007 9:23 am Post subject: RE:Generating a Random Number |
|
|
I have, I'm just not really made out for this stuff  |
|
|
|
|
 |
lilmizeminem

|
Posted: Thu May 17, 2007 10:37 am Post subject: RE:Generating a Random Number |
|
|
your need a variable for num and then declare it as a randant number so that it will be any number .. i think , in turing search randint in the help window. |
|
|
|
|
 |
chrisbrown

|
Posted: Thu May 17, 2007 8:46 pm Post subject: RE:Generating a Random Number |
|
|
on a bit of a tangent here, but does anyone else think that randint instead of Rand.Int (and all the other dumbed-down functions (drawfillbox??)) completely destroy the concept of classes, making learning real languages more difficult to understand? Just one of my annoyances with Turing. |
|
|
|
|
 |
Clayton

|
Posted: Thu May 17, 2007 8:58 pm Post subject: RE:Generating a Random Number |
|
|
In this case, it's not so much classes that it's affecting, but modules and compartmentalizing of code. When you call a pre-defined function or procedure, do you know where the code for it is? Calling drawfillbox() gives you no indication of where the code for that might be. With Draw.FillBox() however, you know exactly where that code is. It's in the Draw module in the folder you installed Turing to. Now, randint() vs. Rand.Int() is a completely different beast that I don't really feel like getting into right now, but just check [Turing Tutorials] for the tutorial on Functions vs. Procedures. I wrote one, as did Cervantes. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Dan
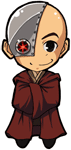
|
Posted: Fri May 18, 2007 12:09 am Post subject: RE:Generating a Random Number |
|
|
If i remember corectly, the randint, drawfillbox, ect methods are for legicy suport of old dos turing. The 1st versions of turing did not have any OOP or class suport so all of the comands where in the fourm of methods.
Once OOP was interdounce to turing, they started coverting all of the comands to moduels and alot of the time they impored the comands in the modules and not the method fourms.
I sugest ignoring the legecly suport comands unless you are progmaing in DOS turing, they shouldbe consdired to be depresatead. |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
rollerdude

|
Posted: Fri May 18, 2007 9:27 am Post subject: Re: Generating a Random Number |
|
|
sounds like you need somethijng as simple as
code: |
var side:int:=Rand.Int(1,2) %such as which side it lands on
% if it = 1 then heads, 2 then tails...
|
|
|
|
|
|
 |
dymd3z
|
Posted: Wed May 23, 2007 8:55 am Post subject: RE:Generating a Random Number |
|
|
I tried what you said, rollerdude, but I'm still not getting it to stop. Is there anythnig wrong with what I have?
var x1 : int := 0
var x2 : int := 0
var side : int := Rand.Int (1, 2)
var guess : int
var heads : int := Pic.FileNew ("heads.jpg")
var tails : int := Pic.FileNew ("tails.jpg")
put "Guess heads or tails. 1 = heads, 2 = tails; only enter 1 or 2"
get guess
loop
cls
x1 := heads + side
Pic.Draw (heads, 250, x1, picCopy)
delay (200)
cls
x2 := tails + side
Pic.Draw (tails, 250, x2, picCopy)
delay (200)
exit when x1 = guess or x2 = guess
if x1 = guess then
put "You guessed right, it landed on heads!"
elsif x2 = guess then
put "You guessed right, it landed on tails!"
else
put "You guessed wrong"
end if
end loop |
|
|
|
|
 |
lilmizeminem

|
Posted: Wed May 23, 2007 11:14 am Post subject: RE:Generating a Random Number |
|
|
yeah methodoxx
turing should be a alittle bit smarter
and have easier codes for the programmers to use
so that its easier for us to make our programs lol
before we even started turing in class my teacher told us that turing was relly dumb as in it doesnt know what our talkin about unless you do the exact codes it need
iunno just my opinion ahaahha! |
|
|
|
|
 |
Albrecd
|
Posted: Wed May 23, 2007 12:53 pm Post subject: Re: Generating a Random Number |
|
|
The reason it won't end is that you are making x1 and x2 equal to pictures as well as the random number, so they won't be 1 or 2, which is what the user is guessing.
instead of x1 := heads + side it should be x1 := side because side will equal either 1 or 2 (randomly decided) and the user will guess 1 or two. And you shouldn't need x2. |
|
|
|
|
 |
|
|