T.T Aggignment due... help!
Author |
Message |
kay188
|
Posted: Tue Dec 05, 2006 6:19 pm Post subject: T.T Aggignment due... help! |
|
|
Yah, i know, i know, I'm a n00b at programming. Espically turing. It's soo hard! please help!
code: |
Calculating the day of the week
The following algorithm is valid for the Gregorian calendar, which was adopted at different times in different parts of the world.
Algorithm:
Add the following numbers produced by consideration of the century, year, month and day of the given date.
Century
Divide centuries-figure by 4, take the remainder from 3, then multiply the result by 2.
Example
Years beginning with 18 (1800 - 1899):
18 / 4 gives remainder 2, 3 - 2 = 1, then 2*1 = 2
Year
Every fourth year is a leap year. Hence, divide the two year-digits by 4 and add the remainder (i.e. the number of leap years since the beginning of the century).
Example:
1978:
78 / 4 = 19
78 + 19 = 97
Month
Refer to the month table below:
Month Associated value
January 0 (in leap year 6)
February 3 (in leap year 2)
March 3
April 6
May 1
June 4
July 6
August 2
September 5
October 0
November 3
December 5
Day
The day represents the actual value.
Example:
January 22, add 22.
Once you add all the values up, divide the sum by 7 and the remainder will tell you the day of the week according to the following table:
Remainder Day of the week
0 Sunday
1 Monday
2 Tuesday
3 Wednesday
4 Thursday
5 Friday
6 Saturday
Example:
December 10th, 1978
Century: 0
Year: 78 + 19
Month: 5
Day: 10
0 + 78 + 19 + 5 + 10 = 112
112 / 7 = 16 remainder 0
Hence, December 10th, 1978 was a Sunday.
The Program
Create a Turing program that implements the above algorithm. Prompt the user to enter a date and then inform the user which day of the week the date represents.
Refer to the programming guidelines presented in class to ensure the elegance and efficiency of your code.
|
Thanks... |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Dan
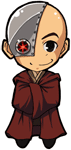
|
Posted: Tue Dec 05, 2006 6:46 pm Post subject: (No subject) |
|
|
As i am shure many will post and tell you, we do not do people's assignments for them here. That dose not help us and it will not help you in the long run (noramly you can't ask us questions during the exam). We are here to share knowagle about programing, ideas and just generally be a community for programmers and students of computer science.
If you take the time to try and solve this assigment and get stuck, then come to us with the code you tryed and a real question, "like how do find how many days in a month?, this is what i tryed so far.....". Then peoleop will be more then happy to help you.
Noramly you will not get just get some to do your coding for you but we will show you where you can find tutorals that will teach you how, tips and pointers as you learn and if you get stuck we will give examples on where to go next.
You most rember that most peoleop here are students like your self, or other peoleop with a background in computer science that take time out of there lives to help you. No one is geting payed for this, not even the peoleop who own the site.
So in summery please read the rules (in the genernal disction forum) and the stickys in this forum and try again. |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
kay188
|
Posted: Wed Dec 06, 2006 10:42 pm Post subject: (No subject) |
|
|
okay then, i wasnt asking someone to do it for me, i was asking for help on how to start it. because i have no idea how to.
here's what i got so far.
code: | var century : int
var year : int
var month : int
var day : int
var total : int
var dayword : string
put "Enter year"
get year
put "Enter Month"
get month
put"Enter Day"
get day
century := year div 100
century := (3 - (century mod 4)) * 2
|
|
|
|
|
|
 |
TokenHerbz

|
Posted: Thu Dec 07, 2006 2:26 am Post subject: (No subject) |
|
|
i see vars: dayword and total, declared and not used.... You where going to use them but didn't? why?
/me thinks your not tring very hard.... |
|
|
|
|
 |
kay188
|
Posted: Sat Dec 09, 2006 2:00 am Post subject: (No subject) |
|
|
man, that's the hardest i can go, i thought all night, but i still cant think of anytheing else. that part was only the beginning though |
|
|
|
|
 |
Hackmaster
|
Posted: Sat Dec 09, 2006 6:50 pm Post subject: (No subject) |
|
|
I have to say.. for someone who wants help, you aren't being very helpful...
Is that first thing you put up the assignment sheet?
What is it you are trying to solve? a key part, or the whole thing?
You can't really get help if you aren't helpful to the people trying to help you.
do you need to use that particular algorythem? there are probably easier, less confusing ways to do it.
Sadly, I can't really come forward and help without knowing the whole problem, but maybe it would be simpler for you this way:
there are 7 named days in a week. there are 4 weeks in a month, plus a few days. (depending on the month, and you can make this a constant)
all you would do is have some kind of a counter that has an if statement condition:
code: |
if counter > 7 then
counter =counter+ 1
end if
|
each number correlates to a day. you don't even need to deal with weeks. a very, very simple way to complete this problem involves counting in the highly obscure "heptal" (yes, it exists!) counting system, but there is a good chance you don't know what that is.
Anyways, what is your programming Experience? I truly wish that people would tell me so I could answer thier questions more acuratly to their level of knowledge. Whatever.
I hope this helps, and if it doesn't, I don't mind explaning it better, but tell me your programming Experience first. |
|
|
|
|
 |
kay188
|
Posted: Sat Dec 09, 2006 8:08 pm Post subject: (No subject) |
|
|
sorry, lol. well, i'm not very good at progrmsamming because personally i don't like it. I like more of the hardware better.
The first post is the assignment. I have to create a calculator that calculates the days of the week. Like you enter the year, day, and month, and it tells you what day of the week it was like if you enter today's year, month and day, it would tell you it is a saturday. (for me) |
|
|
|
|
 |
Hackmaster
|
Posted: Sat Dec 09, 2006 11:05 pm Post subject: (No subject) |
|
|
It's alright... it's your first offense... now no more!
Alrighty. so, the best way to approach this in my opininon is the same as before. count by seven. simply assign each day of the week to an number, by using constants. in case you don't know what they are, they are values that don't change throughout the program. they are mainly used so you can change one value, and it will do lots of things in your program. they are declared thusly:
code: |
constant MONDAY : int := 1
|
it is usually good practice to make the name of a constant in all caps.
essentially, it has the same declaration assembly as a var statement. you say the keyword, in this case constant, and what it is named, following directly afterwards. then, you declare the type, and what it's value is. you can't assign values to constants after they are declared, so do it then.
after you assign all the days of the week to constants, you should also make constants for the months. except, instead of assigning them a 1-12
value, you should assign them 30, 31, or 28 (or 29). then, figure out which years are leap years. you can also do this on the fly, as opposed to using constants.
now... for the logic... this is the hardest part. I'm going to leave most of this to you, so I don't give you the whole thing, but I will give some hints.
you need to count the days manually. there are better ways to do this, but because of your realative inexperince, I will use an easy verison. count the days manually, and every time your count would go to 8, set it back to 1, and keep going. that way, the number will be one - 7, which correlates to a constant, and voila! you are done!
programming is simple when you get the hang of it. unfourtunatly, there are a huge number of people who don't stick with it long enough to make it easier. Programming is a bell curve. it starts easy. then, it gets hard fast. lots of logic, new commands... it all flys at you soooo fast..... and then, you come to a point when it gets easier. you begin to see problems, and know just how to fix them. things become obvious, and then it gets to a point where gigantic things, like full sized RPGs, become more obvious. you break it down, and things just fit. you are probably getting to the steep part of the curve, and I really hope you stick with it. it gets so much better at the top! hope to see you there!
I hope this post helps... and I hope I didn't kill you with philosophy... I can be known to do that. thanks, and good luck! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
TokenHerbz

|
Posted: Sun Dec 10, 2006 11:12 pm Post subject: (No subject) |
|
|
not all months have a monday starting at day 1. |
|
|
|
|
 |
Hackmaster
|
Posted: Mon Dec 11, 2006 12:40 pm Post subject: (No subject) |
|
|
Token, I understand that not every month begins with monday the first, but what i'm saying is that you should iterate through each day, reseting the day count to 1 every time it hits 8. that way, as you go through the months, you will keep that number between 1- 7, and then you can correlate that number to a day, monday through Sunday. do you understand, token?  |
|
|
|
|
 |
kay188
|
Posted: Tue Dec 12, 2006 11:48 pm Post subject: (No subject) |
|
|
i'm REALLY confused...
and now the class is on to drawing objects in turing.... |
|
|
|
|
 |
Hackmaster
|
Posted: Wed Dec 13, 2006 8:26 am Post subject: (No subject) |
|
|
hold up... you mean doing objects and classes? or using commands like drawfillbox and such like that? |
|
|
|
|
 |
kay188
|
Posted: Mon Dec 18, 2006 1:20 pm Post subject: (No subject) |
|
|
no, we have to create a calculator that you enter the year, and the day, and the month, and it tells you hwat day of the week it was. and i tried my hardest.. i still coundn't get it |
|
|
|
|
 |
ericfourfour
|
Posted: Mon Dec 18, 2006 4:05 pm Post subject: (No subject) |
|
|
key188, this is more of a math problem, than programming. Write it out on a piece of paper. Write a few test cases. Once you've figured it out, convert it to code. It should not be difficult. |
|
|
|
|
 |
Hackmaster
|
Posted: Wed Dec 20, 2006 8:22 pm Post subject: (No subject) |
|
|
you know, kay, what eric said is right. I fully agree, and not just for this problem. Almost all programming problems can be avoided with planning. also, even if you manage to code the stuff , it's usually messy as hell. I confess to several times approching HUGE projects (5000+ lines) and getting tottaly wrecked, becuase My variable names suck, or it's not orginized, or whatever. The golden rule (learn from experience) Plan your Work! |
|
|
|
|
 |
|
|