Impossible Program
Author |
Message |
Fozmik
|
Posted: Sat Nov 11, 2006 8:22 pm Post subject: Impossible Program |
|
|
This program determines change for a twenty dollar bill. The cost is determined by the user. At the end of the program the user has a choice to either end the program or to start over. I decided to try something different and use Input.Keydown rather than a get. The program wasn't waiting for input so i put the Input.Keydown into a loop, which is nested in several other loops that are needed by the whole program. When the user presses the 'n' key, all the loops are ended and the window is closed. The program is supposed to loop from the beginning when the 'y' key is pressed, but so far this seem impossible because no matter what the loop that is covering the entire program is being ended.
Basically, I think a solution to this would be to skip the line that is ending the loop when the user presses 'y'. Ive checked the Tutorials and read through Turing's help and so far all I've found is that Turing does not have a Goto function to do this.
Maybe I could make the program restart when the 'y' key is pressed?
code: | var price : real
var change : real
var pennies : real
var answer : array char of boolean
var window : int
window := Window.Open ("position:center;center,graphics:250;260")
put "This program calculates the "
put "most ideal change for a twenty"
put "dollar bill."
put " "
loop
loop
loop
put "The total cost of the item(s)"
put "purchased is $" ..
get price
if price > 20
then
cls
put "The amount must be equal to or smaller than $20."
put " "
elsif price <= 20
then
exit
end if
end loop
cls
if price = 20
then
put "No change."
exit
else
put "The cost is $", price : 0 : 2
put "Your change is:"
put " "
end if
change := 20 - price
if change >= 10
then
if change div 10 > 1
then
put change div 10 ..
put " ten dollar bills.", "$", change div 10, ".00"
else
put "1 ten dollar bill."
end if
change := change mod 10
end if
if change >= 5
then
if change div 5 > 1
then
put change div 5 ..
put " five dollar bills."
else
put "1 five dollar bill."
end if
change := change mod 5
end if
if change >= 2
then
if change div 2 > 1
then
put change div 2 ..
put " toonies."
else
put "1 toonie."
end if
change := change mod 2
end if
if change >= 1
then
if change div 1 > 1
then
put change div 1 ..
put " loonies."
else
put "1 loonie."
end if
change := change mod 1
end if
if change >= 0.25
then
if change div 0.25 > 1
then
put change div 0.25 ..
put " quarters."
else
put "1 quarter."
end if
change := change mod 0.25
end if
if change >= 0.1
then
if change div 0.1 > 1
then
put change div 0.1 ..
put " dimes."
else
put "1 dime."
end if
change := change mod 0.1
end if
if change >= 0.05
then
if change div 0.05 > 1
then
put change div 0.05 ..
put " nickels."
else
put "1 nickel."
end if
change := change mod 0.05
end if
pennies := change * 100
if change < 0.015 and change > 0.001
then
put "1 penny."
elsif change > 0.015
then
put pennies ..
put " pennies."
end if
locate (maxrow - 3, 1)
put "Press 'n' to exit the program."
put "Press 'y' to receive change "
put "again."
exit
end loop
loop
Input.KeyDown (answer)
if answer ('y')
then
cls
exit
elsif answer ('n')
then
exit
end if
end loop
exit
end loop
Window.Close (window) |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
shoobyman

|
Posted: Sat Nov 11, 2006 8:55 pm Post subject: (No subject) |
|
|
code: |
var price : real
var change : real
var pennies : real
var answer : array char of boolean
var window : int
window := Window.Open ("position:center;center,graphics:250;260")
put "This program calculates the "
put "most ideal change for a twenty"
put "dollar bill."
put " "
loop
loop
loop
put "The total cost of the item(s)"
put "purchased is $" ..
get price
if price > 20
then
cls
put "The amount must be equal to or smaller than $20."
put " "
elsif price <= 20
then
exit
end if
end loop
cls
if price = 20
then
put "No change."
exit
else
put "The cost is $", price : 0 : 2
put "Your change is:"
put " "
end if
change := 20 - price
if change >= 10
then
if change div 10 > 1
then
put change div 10 ..
put " ten dollar bills.", "$", change div 10, ".00"
else
put "1 ten dollar bill."
end if
change := change mod 10
end if
if change >= 5
then
if change div 5 > 1
then
put change div 5 ..
put " five dollar bills."
else
put "1 five dollar bill."
end if
change := change mod 5
end if
if change >= 2
then
if change div 2 > 1
then
put change div 2 ..
put " toonies."
else
put "1 toonie."
end if
change := change mod 2
end if
if change >= 1
then
if change div 1 > 1
then
put change div 1 ..
put " loonies."
else
put "1 loonie."
end if
change := change mod 1
end if
if change >= 0.25
then
if change div 0.25 > 1
then
put change div 0.25 ..
put " quarters."
else
put "1 quarter."
end if
change := change mod 0.25
end if
if change >= 0.1
then
if change div 0.1 > 1
then
put change div 0.1 ..
put " dimes."
else
put "1 dime."
end if
change := change mod 0.1
end if
if change >= 0.05
then
if change div 0.05 > 1
then
put change div 0.05 ..
put " nickels."
else
put "1 nickel."
end if
change := change mod 0.05
end if
pennies := change * 100
if change < 0.015 and change > 0.001
then
put "1 penny."
elsif change > 0.015
then
put pennies ..
put " pennies."
end if
locate (maxrow - 3, 1)
put "Press 'n' to exit the program."
put "Press 'y' to receive change "
put "again."
exit
end loop
loop
Input.KeyDown (answer)
if answer ('y') then
cls
exit
elsif answer ('n') then
quit
end if
end loop
end loop
Window.Close (window)
|
try that instead, it will completely close the program if you press no, or it will start again if you press yes. Just fix it cuz it won't display the purpose of the program when you say yes |
|
|
|
|
 |
Fozmik
|
Posted: Sat Nov 11, 2006 10:19 pm Post subject: (No subject) |
|
|
That works great except I get a runtime error when I run it as an .exe |
|
|
|
|
 |
Tony
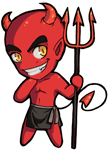
|
Posted: Sat Nov 11, 2006 10:46 pm Post subject: (No subject) |
|
|
that does not sound right.. but then again you provided no details in that statements (what's the error? what version of Turing have you used? any special compile options?) |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Clayton

|
Posted: Sat Nov 11, 2006 10:50 pm Post subject: (No subject) |
|
|
thats because quit simply generates an error to quit the program, instead have some sort of boolean to exit both loops ie:
code: |
var finished : boolean := false
loop
loop
%code here
loop
Input.KeyDown (answer)
if answer ('y') then
cls
exit
elsif answer ('n') then
finished := true
exit
end if
end loop
exit when finished
end loop
|
|
|
|
|
|
 |
Fozmik
|
Posted: Sat Nov 11, 2006 11:04 pm Post subject: (No subject) |
|
|
That fixes it, thanks alot  |
|
|
|
|
 |
|
|