Functions and Updating two values
Author |
Message |
EiCCA
|
Posted: Wed Jun 14, 2006 11:43 am Post subject: Functions and Updating two values |
|
|
There are two questions I have here in this topic
1) In C++, you can declare a function type as void, meaning it does not return a value. What is the term for this in Turing?
2) I have a situation where I need two variables in a funtion to update their values after the function closes.
For example:
code: |
function asdf(x : int, y : int) : <void>
x := 5
y := 6
end asdf
put x
put y
|
In C++, you can do this using refrence paramaters, using the & beside the paramater name, however I do not know how this is done in Turing.
Help? Thanks a lot |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Wed Jun 14, 2006 12:54 pm Post subject: (No subject) |
|
|
1. Such things are known as procedures in Turing.
2. You can do this in Turing by prefixing the parameter name with "var ". However, for such a simple case, there is a better approach. Simply link both variables into a single data type, then return that.
code: | type Point :
record
x, y : int
end record |
Or in C++ simply use the std::pair class as defined by the STL. |
|
|
|
|
 |
Tony
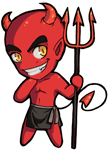
|
Posted: Wed Jun 14, 2006 6:47 pm Post subject: (No subject) |
|
|
code: |
function asdf(x : int, y : int) : <void>
x := 5
y := 6
end asdf
put asdf(1,2)
|
the above clearly doesn't work. X and Y are local variables.
What'd you'd need to do is pass the reference to the variable's address. Now you're getting into pointers and what not. Turing might actually have a similar syntax involving &, but I don't recall at the moment. Consult your F10 manual.
Raguardless, the best practice is to have the function return the updated values. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
[Gandalf]

|
Posted: Wed Jun 14, 2006 7:01 pm Post subject: (No subject) |
|
|
Tony, like wtd said you can get the pass by reference effect by prefixing the parameter name with "var", for example:
code: | procedure changeValue (var num : int)
num += 5
end changeValue
var myValue : int := 7
put myValue
changeValue (myValue)
put myValue %outputs 12 |
Though yes, you could also return a type containing all your data. |
|
|
|
|
 |
Tony
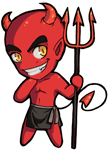
|
|
|
|
 |
|
|