two procedures at once
Author |
Message |
teh_1337_1
|
Posted: Sun Apr 16, 2006 10:23 pm Post subject: two procedures at once |
|
|
i was wondering this after i played a game made in turing. how can you have two procedures go off at once? i have this as an example kinda:
code: | var x, y, button, oldx, oldy : int
setscreen ("offscreenonly")
procedure makeCircle
if button = 1 then
oldx := x
oldy := y
for i : 1 .. 25
Draw.Oval (oldx, oldy, i, i, blue)
View.Update
delay (20)
cls
end for
end if
end makeCircle
loop
Mouse.Where (x, y, button)
makeCircle
View.Update
delay (100)
cls
end loop
|
how would i make that so you could make 2 circles or as many circles as the user wants without having to wait for the other ones to end? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
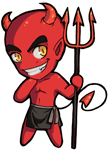
|
Posted: Sun Apr 16, 2006 10:45 pm Post subject: (No subject) |
|
|
you need an array for the circles, and each time through the loop you just take a look at what circles are not complete yet, and advance a frame.
Check the Turing Walkthrough for flexible arrays, records |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
NikG
|
Posted: Mon Apr 17, 2006 7:59 am Post subject: (No subject) |
|
|
You may need to change your makeCircle proc though. Specifically, I would remove that cls from it (since you already have one in your main loop.
Also, I would remove the button click code from the proc and put it in your main loop.
Post here (or PM me) if you didn't get what I meant. |
|
|
|
|
 |
teh_1337_1
|
Posted: Mon Apr 17, 2006 10:02 am Post subject: (No subject) |
|
|
im still not exactly sure what you mean, could you show me an example or something? |
|
|
|
|
 |
NikG
|
Posted: Mon Apr 17, 2006 2:26 pm Post subject: (No subject) |
|
|
Here's a modified version of your code: code: | setscreen ("offscreenonly")
var x, y, button, counter : int
var circles : array 1 .. 100, 1 .. 3 of int
procedure makeCircle
circles (counter, 1) := x
circles (counter, 2) := y
circles (counter, 3) := 1
end makeCircle
procedure drawCircle (x, y : int, var r : int)
Draw.Oval (x, y, r, r, blue)
r += 1
if r > 25 then
r := -1
end if
end drawCircle
counter := 0
loop
Mouse.Where (x, y, button)
if button = 1 then
counter += 1
makeCircle
end if
for i : 1 .. counter
if circles (i, 3) not= -1 then
drawCircle (circles (i, 1), circles (i, 2), circles (i, 3))
end if
end for
View.Update
delay (50)
cls
end loop |
Look at the code carefully, and be warned, you are entering more advanced coding now (mutidimensional arrays, passing variables into procedures by reference...) |
|
|
|
|
 |
[Gandalf]

|
Posted: Mon Apr 17, 2006 5:30 pm Post subject: (No subject) |
|
|
NikG, note that multidimensional arrays as used in your example code can be avoided by using records. The record would hold the x and y co-ordinates and the radius of your circle so you would only need to create a one dimensional array of your record. Check out the Turing Walkthrough for the tutorial on them. |
|
|
|
|
 |
|
|