Author |
Message |
stef_ios

|
Posted: Tue Apr 04, 2006 8:12 am Post subject: Lower case to upper case |
|
|
Hey. I have to write a program that can get a name in lower case and then change the word and output it in all upper case letters. Here is the code I have so far, but I do not know how to write the most important part. I know I have to (in a loop) get the ASCII code of the letter (using ord), then subtract 32, and then output the number using it's chr equivalent. Can someone help me? Thanks so much. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Andy
|
Posted: Tue Apr 04, 2006 8:24 am Post subject: (No subject) |
|
|
sooo where is this code of yours? |
|
|
|
|
 |
stef_ios

|
Posted: Tue Apr 04, 2006 8:35 am Post subject: (No subject) |
|
|
oops. sorry. here is the start.
var name: string
var strlen: real
put "Please enter your name in lower case letters: "
get name
loop
end loop
I am a noobie at this stuff. Anyways, I know I have to use ord, and chr, and strlen. I don't know how though. |
|
|
|
|
 |
NikG
|
Posted: Tue Apr 04, 2006 8:36 am Post subject: (No subject) |
|
|
Quote: I know I have to (in a loop) get the ASCII code of the letter (using ord), then subtract 32, and then output the number using it's chr equivalent.
That sound's about right. So what's the problem?
Remember, if you're going to need this often then create a function. And don't forget to check whether the letter you are converting is indeed a lower case letter; otherwise you'll get a weird symbol. |
|
|
|
|
 |
NikG
|
Posted: Tue Apr 04, 2006 8:42 am Post subject: (No subject) |
|
|
Oops, too slow with my first post.
Anyways, think about what you need stef_ios.
You're going to need to check each letter of whatever the user inputs. So yes you will need a loop, but is there a better choice than the normal loop?
Here's a tip:
if you have a string variable, you can access individual letters simply by putting a # in brackets.
code: | var myStr:="hello"
put myStr(2) %Output = e |
|
|
|
|
|
 |
Andy
|
Posted: Tue Apr 04, 2006 8:58 am Post subject: (No subject) |
|
|
A few things
the best tip i could give you is that you should create an seperate string to store the UpperCased letters.
Instead of using a loop, use a for loop to go from 1 to length(name)
in the body of the for loop, check to make sure if the current character is between 'a' and 'z' if it is, subtract 32 from the ord(character) then use chr to find the corresponding uppercase and attatch that to the end of your uppercased string
if the character is between 'A' and 'Z' simply attatch it to the end of your uppercased string.
what is the use of strlen? |
|
|
|
|
 |
stef_ios

|
Posted: Tue Apr 04, 2006 6:00 pm Post subject: (No subject) |
|
|
strlen is the variable used to find the length of the word, which is a string. |
|
|
|
|
 |
person
|
Posted: Tue Apr 04, 2006 6:20 pm Post subject: (No subject) |
|
|
y is it of type real? also, its not necessary at all |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
chrispminis
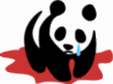
|
Posted: Tue Apr 04, 2006 11:30 pm Post subject: (No subject) |
|
|
Just a note, you may not be permitted to use it, but there is a Turing predefined function that does this. Can't recall atm, but you should be able to find it in the Turing help files. |
|
|
|
|
 |
stef_ios

|
Posted: Wed Apr 05, 2006 7:59 am Post subject: (No subject) |
|
|
can someone just start the code for me, so i can be on the right track, because i have absolutely no clue what i'm doing right now. Thanks. |
|
|
|
|
 |
md

|
Posted: Wed Apr 05, 2006 9:37 am Post subject: (No subject) |
|
|
pseudo: |
for i in string do
string[i] -= 'a'-'A';
|
|
|
|
|
|
 |
stef_ios

|
Posted: Wed Apr 05, 2006 3:03 pm Post subject: (No subject) |
|
|
Could someone please help me. I actually have no clue how to do this, and it's an assignment. Could someone walk me through the steps (with examples), because i'm lost. I know what i have to do, i just don't know how to put it into turing. |
|
|
|
|
 |
stef_ios

|
Posted: Wed Apr 05, 2006 3:21 pm Post subject: (No subject) |
|
|
code: |
var name_1: string
var name_2: string
var name_3: string
var name_4: string
var name_5: string
put "Please enter a five letter word (or name): "
get name_1, name_2, name_3, name_4, name_5
for i: name_1 ..
put ord name_1 - 32
end for
for i: name_2 ..
put ord name_2 - 32
end for
for i :name_3 ..
put ord name_3 - 32
end for
for i: name_4 ..
put ord name_4 - 32
end for
for i :name_5 ..
put ord name_5 - 32
end for
|
Okay. So that is the code I came up with, but it doesn't work. Can someone check it over. Also, I need it to diplay the uppercase letter using the chr. Thanks. |
|
|
|
|
 |
Delos

|
Posted: Wed Apr 05, 2006 3:39 pm Post subject: (No subject) |
|
|
ord() works only on a single character, and you'll need to pass it as a parameter:
code: |
var word : string := "Hello"
for i : 1 .. length (word)
put ord (word (i))
end for
|
Next:
code: |
%...
put ord (word (i)) + 5
%...
|
And finally:
code: |
%...
put chr (ord (word (i)) + 5)
%...
|
There you go, manipulation basics. From here you can figure out how to do the lower->UPPER conversions or vice versa. |
|
|
|
|
 |
stef_ios

|
Posted: Wed Apr 05, 2006 4:24 pm Post subject: (No subject) |
|
|
Thanks for all the tips. Greatly appreciated. |
|
|
|
|
 |
|