Syntax Typo?
Author |
Message |
chrispminis
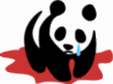
|
Posted: Tue Mar 14, 2006 2:48 pm Post subject: Syntax Typo? |
|
|
Yeah, this isn't a big problem. I've just been staring at my code for about 40 minutes, and I still haven't found the problem. There are 2 errors. One is for a string literal that should end the line, the other is a syntax error expecting then.
code: | %Text Calculator
%by : Chris Pan
%Evaluates expressions by reading and interpreting strings
%Don't Steal
%Variables
var stringy : string
var number : real := 0
var mark, negmark : int
var string1, string2 : string
var dec, neg : boolean := false
var maxsymbol : int := 10
type prioritize :
record
svalue : string
smark : int
spriority : int
sused : boolean
end record
var symbol : array 1 .. maxsymbol of prioritize
for i : 1 .. maxsymbol
symbol (i).svalue := " "
symbol (i).smark := 0
symbol (i).spriority := 0
symbol (i).sused := false
end for
%Functions
function stringreal (str : string) : real
mark := index (str, ".")
negmark := index (str, "-")
if mark > 0 then
dec := true
end if
if negmark > 0 then
neg := true
end if
for i : 1 .. length (str)
if str (i) >= "1" and str (i) <= "9" then
number *= 10
number += (ord (str (i)) - 48)
end if
end for
if dec = true then
number := number / (10 ** (length (str) - mark))
end if
if neg = true then
number := -number
end if
result number
end stringreal
function add (num1, num2 : real) : real
result num1 + num2
end add
function subtract (num1, num2 : real) : real
result num1 - num2
end subtract
function multiply (num1, num2 : real) : real
result num1 * num2
end multiply
function divide (num1, num2 : real) : real
result num1 / num2
end divide
function power (num1, num2 : real) : real
result num1 ** num2
end power
function squareroot (num1 : real) : real
result sqrt (num1)
end squareroot
function estimate (num1 : real) : int
result round (num1)
end estimate
function up (num1 : real) : int
result ceil (num1)
end up
function down (num1 : real) : int
result floor (num1)
end down
%Main
%Input
get stringy : *
%Identify and Prioritize
for i : 1 .. length (stringy)
if stringy (i) not= " " and stringy (i) < "0" or stringy (i) > "9" then
symbol (i).sused := true
symbol (i).svalue := stringy (i)
symbol (i).smark := i
end if
end for
for i : 1 .. length (stringy)
if symbol (i).sused = true then
if symbol (i).svalue = "q" or symbol (i).svalue = "h" then
symbol (i).spriority := 7
elsif symbol (i).svalue = "=" then
symbol (i).spriority := 6
elsif symbol (i).svalue = "~" or symbol (i).svalue = "<" or symbol (i).svalue = ">" then
symbol (i).spriority := 5
elsif symbol (i).svalue = "+" or symbol (i).svalue = "-" then
symbol (i).spriority := 4
elsif symbol (i).svalue = "x" or symbol (i).svalue = "X" or symbol (i).svalue = "*" or symbol (i).svalue = "/" then
symbol (i).spriority := 3
elsif symbol (i).svalue = "^" or symbol (i).svalue = "&" then
symbol (i).spriority := 2
elsif symbol (i).svalue = "(" then
symbol (i).spriority := 1
end if
end if
end for
%Test Output
for i : 1 .. length (stringy)
if symbol (i).sused then
put symbol (i).svalue
put symbol (i).smark
put symbol (i).spriority
end if
end for
|
Here's my code, I was just testing my prioritizing, for BEDMAS. I am sure the error is linked to an extra " somewhere but I can't find it! Please help. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Delos

|
Posted: Tue Mar 14, 2006 3:27 pm Post subject: (No subject) |
|
|
code: |
elsif symbol (i).svalue = "\^" or symbol (i).svalue = "&" then
|
Not sure if it achieves what you want it to though... |
|
|
|
|
 |
chrispminis
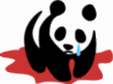
|
Posted: Tue Mar 14, 2006 3:33 pm Post subject: (No subject) |
|
|
I'm not sure what you are trying to point me towards. But where did the "\^" come from?
Im trying to prioritize them, "&" is my chosen square root sign. So exponents and square root go to the same priority level. |
|
|
|
|
 |
pavol
|
Posted: Tue Mar 14, 2006 4:48 pm Post subject: (No subject) |
|
|
im not sure, you have more knowledge in this matter than i do, but if you take ^ out of:
code: | elsif symbol (i).svalue = "^" or symbol (i).svalue = "&" then
symbol (i).spriority := 2 |
to make it:
code: | elsif symbol (i).svalue = "" or symbol (i).svalue = "&" then
symbol (i).spriority := 2 |
i know it probably doesn't make your program do what you want, but what i'm trying to point out is that maybe "^" is the problem and maybe it is some kind of thing held special by Turing and you're not really allowed using it.
hope it gives some insight into your problem  |
|
|
|
|
 |
Delos

|
Posted: Tue Mar 14, 2006 4:55 pm Post subject: (No subject) |
|
|
pavol is on the right track. '^' is a 'special thing' in Turing, but you are allowed to use it, provided you know how. In this case, if you wish to use it in a string, you need to provide the escape character '\' first. This is similar to using things like '\n' or '\"'.
I'm guessing the '^' would be for an exponent... |
|
|
|
|
 |
MysticVegeta

|
Posted: Tue Mar 14, 2006 6:42 pm Post subject: (No subject) |
|
|
no ** is for exponent. ^ is reserved for other string purpose, and yes you would need to put "\" before the ^ so it would be "\^", its the same as saying "^", its for the same reason as for in java when you have to put "\\+" due to regex issues. |
|
|
|
|
 |
Delos

|
Posted: Tue Mar 14, 2006 8:46 pm Post subject: (No subject) |
|
|
MysticVegeta wrote: no ** is for exponent. ^ is reserved for other string purpose, and yes you would need to put "\" before the ^ so it would be "\^", its the same as saying "^", its for the same reason as for in java when you have to put "\\+" due to regex issues.
I meant in his proggie. You know, typing in "4^3" meaning four raised to the third power... |
|
|
|
|
 |
chrispminis
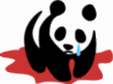
|
Posted: Wed Mar 15, 2006 2:16 pm Post subject: (No subject) |
|
|
well, Ill try that, that makes sense. And Delos it is ** for exponent. Turing doesn't evaluate 2^3 = 8. Just another thing. If you wanted the backslash character (\) in a string would it be "\\"? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
[Gandalf]

|
Posted: Wed Mar 15, 2006 4:39 pm Post subject: (No subject) |
|
|
^ is the more 'universal' exponent representation, while ** is what Turing uses since ^ is reserved for other things.
Yes, to output a backslash you would use escape + \, or else "\\". |
|
|
|
|
 |
Clayton

|
Posted: Wed Mar 15, 2006 4:57 pm Post subject: (No subject) |
|
|
i have to ask what do you mean by using backslash as an escape character? what is an escape character and what are they used for? i know this is off topic but i didnt want to start another thread just for this |
|
|
|
|
 |
Delos

|
Posted: Wed Mar 15, 2006 10:50 pm Post subject: (No subject) |
|
|
Well, the most practical interpretation would be that you 'escape' from the literal string that you're using, and can implement a secondary command instead. So, for instance, you have "Hello /n World", which prints Hello and World on seperate lines. Since it's all in one string, the '/' allows you to drop out from the usual translation and actually do some manipulations.
This is rather limited though, since any more graphically-oriented procedures (Font. etc) don't support this (seeing as they create pictures). And of course, everyone loves pictures.
 |
|
|
|
|
 |
|
|