strreal emulation
Author |
Message |
chrispminis
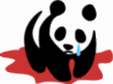
|
Posted: Tue Mar 07, 2006 11:06 pm Post subject: strreal emulation |
|
|
We all know Turing comes with a few helpful conversion commands.
strint
intstr
strreal etc.
But for one of my assignments, these are forbidden. Now one of the sub assignments for this main one is to emulate the strreal function. I have already succeeded in creating a multidigit integer converter but im stumped on decimal numbers. Here's what I have so far:
code: | var str : string
var number : real := 0
var mark : int
var dec : boolean := false
get str
for i : 1 .. length (str) %Check if theres a decimal.
if str (i) = "." then
mark := i
dec := true
end if
end for
for i : 1 .. length (str)
if dec = true then
if str (i) not= "." then
if i < length (str) - mark then
number += (ord (str (* +1 - i)) - 48) * 10 ** (i - 1) %If the number is after the decimal do normal.
elsif i >= length (str) - mark then
number += (ord (str (* +1 - i)) - 48) * 10 ** (i - 2) %If the number is before it then take off a power of 10.
end if
end if
else
number += (ord (str (* +1 - i)) - 48) * 10 ** (i - 1) %Conversion for an integer.
end if
end for
if dec = true then % If there was a decimal then divide the number by appropriate power of 10.
number := number / (10 ** (length (str) - mark))
end if
%Check if conversion was successful and number can be properly manipulated.
put " "
put number
put number * 2
|
You could either improve on this code, or write your own. Im pretty tired right now, and I'm not thinking right, and I've deviated from that code many times, sometimes getting closer but never quite there. If someone could please help. And BTW anyone at my school who stumbles across this, feel free to use it. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Delos

|
Posted: Wed Mar 08, 2006 8:49 am Post subject: (No subject) |
|
|
Quite the problem you have here, eh? A couple of pointers:
code: |
for i : 1 .. length (str) %Check if theres a decimal.
if str (i) = "." then
mark := i
dec := true
end if
end for
|
Could be replaced by:
code: |
mark := index (str, ".")
if mark > 0 then
dec := true
end if
|
Simple, eh?
As for the rest of your code - a big problem arises in that you're assuming the string inputted will always be of a certain length or greater. If it isn't, well - you get some errors occuring in your exponents.
Let's try a different approach. Again, accept a string input of your real number. You can do a proof of it to make sure it is a valid number. Next, identify the position of the decimal (using index()), and use some string manipulation to excise it. (i.e., "5.403" would become "5403"). Convert this number to a real number, but using your integer algorithm. Finally, based upon the initial position of the decimal, divide your number by the requisite expontent of 10.
Yatta! |
|
|
|
|
 |
Andy
|
Posted: Wed Mar 08, 2006 11:05 am Post subject: (No subject) |
|
|
you're trying too hard...
code: |
function myStrreal (s : string) : real
var noDecimal := 0
if index (s (index (s, ".") + 1 .. *), ".") > 0 then
result 31212012
end if
for i : 1 .. length (s)
if s (i) >= '0' and s (i) <= '9' then
noDecimal *= 10
noDecimal += ord (s (i)) - ord ('0')
elsif s (i) not= '.' then
result 31212012
end if
end for
result noDecimal / (10 ** (length (s) - index (s, ".")))
end myStrreal
put myStrreal ("12.2000")
|
EDIT: now its a bit longer, but has error checking. if the thing is not strreal ok, it'll output 31212012, (my pathetic attempt at writeing error in leet) |
|
|
|
|
 |
Tony
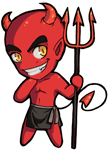
|
|
|
|
 |
jamonathin

|
Posted: Wed Mar 08, 2006 12:54 pm Post subject: (No subject) |
|
|
code: | function myStrrealok (s : string) : string
var ok : string := "false"
var counter : int := 0
for i : 1 .. length (s)
if s (i) not= "." and strintok (s (i)) then
counter += 1
elsif s (i) = "." then
counter += 1
end if
end for
if counter = length (s) then
ok := "true"
end if
result ok
end myStrrealok
put myStrrealok ("12.00001")
|
 |
|
|
|
|
 |
Andy
|
Posted: Wed Mar 08, 2006 1:08 pm Post subject: (No subject) |
|
|
err wtf.. you're using strintok.. the whole point was to not use any of those functions...
code: |
function myStrrealok (s : string) : bool
var hasDecimal := false
for i : 1 .. length (s)
if s (i) < '0' or s (i) > '9' or (s (i) = '.' and hasDecimal) then
result false
elsif s (i) = '.' then
hasDecimal := true
end if
end for
result true
end myStrreal
put myStrreal ("12.20000")
|
|
|
|
|
|
 |
jamonathin

|
Posted: Wed Mar 08, 2006 7:27 pm Post subject: (No subject) |
|
|
Wellz then. here's what I would do instead.
code: | function myStrrealok (s : string) : string
var ok : string := "false"
var counter : int := 0
var numbah : array 1 .. 10 of string := init ("0", "1", "2", "3", "4", "5", "6", "7", "8", "9")
for i : 1 .. length (s)
for w : 1 .. upper (numbah)
if s (i) = numbah (w) then
counter += 1
end if
end for
if s (i) = "." then
counter += 1
end if
end for
if counter = length (s) then
ok := "true"
end if
result ok
end myStrrealok
put myStrrealok ("12.2311312")
|
However comparing string values is much easier and more efficient. |
|
|
|
|
 |
Cervantes

|
Posted: Wed Mar 08, 2006 8:07 pm Post subject: (No subject) |
|
|
You guys are missing one very important feature:
code: |
put myStrrealok ("2e3")
|
Should be true, but it outputs false.  |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Andy
|
Posted: Wed Mar 08, 2006 9:17 pm Post subject: (No subject) |
|
|
blah blah cervantes, shush you.
jamonathin, you're trying too hard.. why use an array when you can simply use ascii values? |
|
|
|
|
 |
jamonathin

|
Posted: Wed Mar 08, 2006 11:17 pm Post subject: (No subject) |
|
|
true true, i forgot about those. I am stumped tho on how to do what cervantes is talkin about; ("2e3"). How can you change it to int, or at least run a for loop with that 3 as a max.  |
|
|
|
|
 |
Andy
|
Posted: Wed Mar 08, 2006 11:57 pm Post subject: (No subject) |
|
|
i update my code tomorrow at work.. |
|
|
|
|
 |
|
|