Final Fantasy III battle game help
Author |
Message |
do_pete

|
Posted: Mon Jan 02, 2006 12:02 pm Post subject: (No subject) |
|
|
What's the difference between getch and getchar? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
DIIST

|
Posted: Mon Jan 02, 2006 1:46 pm Post subject: (No subject) |
|
|
do_pete wrote: What's the difference between getch and getchar?
Getch is a procedure which waits until a specific character is pressed. Getchar is function that returns the character that is pressed. |
|
|
|
|
 |
do_pete

|
Posted: Mon Jan 02, 2006 2:11 pm Post subject: (No subject) |
|
|
Thanks, I understand now |
|
|
|
|
 |
Az
|
Posted: Mon Jan 02, 2006 2:27 pm Post subject: question about getch |
|
|
code: |
loop
put ""
put " " ..
%get user account name
put "Account Name: " ..
get acntName
if acntName = "q" or acntName = "Q" then
exit
end if
put ""
put " " ..
%get user password
put "Password: " ..
get password
put ""
put ""
exit
end loop |
ok that is my code so far for login.. but I dont understand where I would put the getch for the * for the password. i'm not really sure how I code the loop in there |
|
|
|
|
 |
do_pete

|
Posted: Mon Jan 02, 2006 3:13 pm Post subject: (No subject) |
|
|
You need 2 seperate loops; one for the password and one for the account. You'll also need to place the put "Password: " .. and put "Account Name: " .. outside of the loops. The getch and put "*" .. goes inside each loop |
|
|
|
|
 |
Az
|
Posted: Mon Jan 02, 2006 7:39 pm Post subject: password problem |
|
|
code: | put "Password: " ..
loop
%get user password
var ctr : int := 0
var ch : string (1)
ctr += 1
exit when ctr = 10
getch (ch)
put "*"..
end loop |
ok thats what i have for the password... but it still isnt working properly.
whenit asks for a password, the stuff you type in shows up as * but for keys like backspace, it just adds a * insted of backspacing, and the enter key add a * aswell insted of exiting the loop when pressed.
(also i would like to know how to get the ESC key to exit the whole program when the account is being entered or the password) |
|
|
|
|
 |
Cervantes

|
Posted: Mon Jan 02, 2006 8:12 pm Post subject: (No subject) |
|
|
Az wrote:
whenit asks for a password, the stuff you type in shows up as * but for keys like backspace, it just adds a * insted of backspacing, and the enter key add a * aswell insted of exiting the loop when pressed.
Cervantes wrote:
Exit the loop if the enter key is pressed (if you getched chr (10) or something like that.)
code: |
exit when input_char = chr (10)
|
code: |
var str := ""
var ch : string (1)
loop
ch := getchar
exit when ch = KEY_ENTER
if ch = KEY_BACKSPACE & length (str) > 0 then
str := str (1 .. * -1)
elsif index ("abcdefghijklmnopqrstuvwxyzQWERTYUIOPASDFGHJKLZXCVBNM.,;:'\"?()&%$#@!", ch) not= 0 then
str += ch
end if
locate (1, 1)
put str
end loop
|
|
|
|
|
|
 |
Az
|
Posted: Mon Jan 02, 2006 10:18 pm Post subject: password |
|
|
ok this is really bugging me
code: | put "Password: " ..
%get user password
loop
getch (ch)
exit when ch = KEY_ENTER %exit when enter key is pressed
if ch = KEY_BACKSPACE and length (password) > 0 then
password := password (1 .. * -1)
elsif index("abcdefghijklmnopqrstuvwxyzQWERTYUIOPASDFGHJKLZXCVBNM.,;:'\"?()&%$#@!", ch) not= 0 then
password += ch
put "*"
end if
end loop |
that code is for the password in my login, it all works EXCEPT that when I type in the password and press backspace, the password changes but the "*" still remains on the screen.
for example: let's say the password is "hello". when i type in the password "*****" (5) is displayed on the screen but if i press backspace, the password string changes to "hell" but the "*****" (5) is still on the screen rather than "****" (4). so the password changes but the "*" does not.
how do i fix this? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
chrispminis
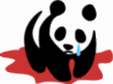
|
Posted: Tue Jan 03, 2006 12:39 am Post subject: (No subject) |
|
|
Not too hard, in your if ch = KEY_BACKSPACE just add afterwards a
put "\B" .. This backspaces and is one of the formatting tools often forgotten Well here's the changes in your code. GL with the rest of the program.
code: |
%get user password
loop
getch (ch)
exit when ch = KEY_ENTER %exit when enter key is pressed
if ch = KEY_BACKSPACE and length (password) > 0 then
password := password (1 .. * -1)
put "\B" ..
elsif index("abcdefghijklmnopqrstuvwxyzQWERTYUIOPASDFGHJKLZXCVBNM.,;:'\"?()&%$#@!", ch) not= 0 then
password += ch
put "*" ..
end if
end loop |
|
|
|
|
|
 |
Az
|
Posted: Tue Jan 03, 2006 12:54 am Post subject: (No subject) |
|
|
chrispminis wrote: Not too hard, in your if ch = KEY_BACKSPACE just add afterwards a
put "\B" .. This backspaces and is one of the formatting tools often forgotten  Well here's the changes in your code. GL with the rest of the program.
thanks! i was stuggling with that for awhile .
anyway, I seemm to be done the login programming. but currently its just a dull white screen with boring text. How would i change the text size/colour/font and also make the background a different colour?.. I am trying to figure that out now but alittle lost so i thought i would post this. any help would be great, thanks |
|
|
|
|
 |
chrispminis
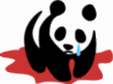
|
Posted: Tue Jan 03, 2006 2:08 am Post subject: (No subject) |
|
|
Quote: but currently its just a dull white screen with boring text. How would i change the text size/colour/font and also make the background a different colour?..
First of all, to change background colour use colourback.
The format is colourback (colourofchoice). Also works with American "color".
ex.
code: |
colourback (12)
cls
|
outputs a red background.
Then to change the colour of normal size text use colour (or color).
colour (colourofchoice)
ex.
code: |
colour (2)
put "This outputs text in Green"
|
But I think you want the Font commands. This is easy enough to understand. You can get the idea from this source code found in the Turing Help Files, on Font.Draw
code: |
var font1, font2, font3, font4 : int
font1 := Font.New ("serif:12")
assert font1 > 0
font2 := Font.New ("sans serif:18:bold")
assert font2 > 0
font3 := Font.New ("mono:9")
assert font3 > 0
font4 := Font.New ("Palatino:24:bold,italic")
assert font4 > 0
Font.Draw ("This is in a serif font", 50, 30, font1, red)
Font.Draw ("This is in a sans serif font", 50, 80, font2, brightblue)
Font.Draw ("This is in a mono font", 50, 130, font3, colorfg)
Font.Draw ("This is in Palatino (if available)", 50, 180, font4, green)
Font.Free (font1)
Font.Free (font2)
Font.Free (font3)
Font.Free (font4)
|
For more detailed information read the Turing Help File on Font.Draw.
Don't forget the locate command if you haven't already learned about them. It locates normal text to a certain row and column. Read about that in the Turing Help File as well. In fact, you can find most of what you need to know in the Turing Help Files, and if it isn't there then check the Tutorials here at compsci.
Also for more professional looking programs, look into buttons. |
|
|
|
|
 |
Cervantes

|
Posted: Tue Jan 03, 2006 8:58 am Post subject: Re: password |
|
|
Az wrote:
for example: let's say the password is "hello". when i type in the password "*****" (5) is displayed on the screen but if i press backspace, the password string changes to "hell" but the "*****" (5) is still on the screen rather than "****" (4). so the password changes but the "*" does not.
how do i fix this?
You've got one solution, but let's examine an alternative, just because knowledge is good.
code: |
var str := "hello"
put repeat ("*", length (str))
|
|
|
|
|
|
 |
Albrecd
|
Posted: Tue Jan 03, 2006 9:13 am Post subject: (No subject) |
|
|
Quote: First of all, to change background colour use colourback.
colourback will not work if you are using graphics too, in that case you just Draw.FillBox (0, 0, maxx, maxy, whatevercolour) |
|
|
|
|
 |
do_pete

|
Posted: Tue Jan 03, 2006 12:35 pm Post subject: (No subject) |
|
|
What are you talking about colourback works |
|
|
|
|
 |
Az
|
Posted: Tue Jan 03, 2006 1:24 pm Post subject: starting the game |
|
|
ok I seem to be done the login of the game, so next I guess I would like to start the battle. So with that in mind, I want to open a new window and imput some GUI or something? lol not really sure what I do but i want it to look something like this...
if you dont know, that is a battle in final fantasy, and i would like my game to turn out with the same concept. I am not sure how i would draw the background, the menu or any of that I have some sort of idea but i would probably struggle for days trying to figure it out I'm thinking for the boxes... just do a Draw.Box or Draw.FillBox, but the background and putting the characters/enemies in, not so sure about that. |
|
|
|
|
 |
|
|