Multiple Falling.
Author |
Message |
chrispminis
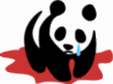
|
Posted: Sun Dec 18, 2005 11:49 pm Post subject: Multiple Falling. |
|
|
Hey, I need some help. I'm trying to get "coins" to fall from the air. So far I have this.
code: | var mx, my, mb : int
var totalcoins : int
var fallspeed : int := 5
View.Set ("graphics:offscreenonly:300;600")
get totalcoins
var coins : array 1 .. totalcoins of int
loop
Mouse.Where (mx, my, mb)
for coinx : 1 .. totalcoins
coins (coinx) := Rand.Int (1, maxx)
end for
for coinx : 1 .. totalcoins
for decreasing coiny : maxy .. 1
Draw.FillOval (coins (coinx), coiny, 5, 5, 14)
delay (fallspeed)
View.Update
cls
end for
end for
end loop
|
1. Why doesn't the View.Set have any effect?
2. How would I get it to drop multiple coins at a time instead of just one (I'm trying to get it so that totalcoins is the amount of coins is the max amount of coins on screen)?
3. How would I get the coins to drop at different times (preferably at a manipulatable rate)?
You don't have to do the work, but some sample source code so I could see the logic would be great. I can't think of how to do it, although i have been looking through the sources from other programs. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Carino

|
Posted: Mon Dec 19, 2005 3:20 am Post subject: (No subject) |
|
|
I got bored, hope this helps.
code: |
var mx, my, mb : int
var totalcoins : int
var fallspeed : int := 5
View.Set ("graphics:offscreenonly:300;600")
type attrib :
record
speed : int
x : int
pos : int
end record
get totalcoins
var coins : array 1 .. totalcoins of attrib
for i : 1 .. totalcoins
coins (i).speed := Rand.Int (1, 5)
coins (i).x := Rand.Int (1, maxx)
coins (i).pos := maxy
end for
loop
%Mouse.Where (mx, my, mb)
for i : 1 .. totalcoins
coins (i).pos -= coins (i).speed
Draw.FillOval (coins (i).x, coins (i).pos, 5, 5, 14)
if coins (i).pos < 0 then
coins (i).speed := Rand.Int (1, 5)
coins (i).x := Rand.Int (1, maxx)
coins (i).pos := maxy
end if
end for
View.Update
delay (10)
cls
end loop
|
|
|
|
|
|
 |
Albrecd
|
Posted: Mon Dec 19, 2005 9:44 am Post subject: (No subject) |
|
|
The View.Set has no effect because it has errors, instead it should be:
code: | View.Set ("graphics:300;600,offscreenonly) |
In order to have multiple coins at one time, you will need multiple X and Y variables: one for each coin. If you want them to fall at different times, you could do something like:
code: | var WhenCoinFall : array 1 .. 3 of int %however many you want, we shall say 3
var counter := 0 %automatically set as an integer
var CoinFalling : array 1 .. 3 of boolean
for i : 1 .. 3
CoinFalling (i) := false
end for
for i : 1 .. 3
randint (WhenCoinFall (i), 1, 5) % min and max can be whatever, we shall say 1 and 5
end for
loop
counter += 1
for i : 1 .. 3
if WhenCoinFall (i) = counter then
CoinFalling (i) := true
end if
end for
for i : 1 .. 3
if CoinFalling (i) = true then
%Put your Y -= or whatever here
end if
end for
end loop |
|
|
|
|
|
 |
Albrecd
|
Posted: Mon Dec 19, 2005 9:47 am Post subject: (No subject) |
|
|
Note: the two for loops in the loop in my example could really be one... Why is there no edit button??? |
|
|
|
|
 |
do_pete

|
Posted: Mon Dec 19, 2005 11:59 am Post subject: (No subject) |
|
|
try something like this:
code: | type Coin :
record
X : int
Y : int
Speed : int
end record
const RADIUS := 5
const AMOUNT := 20
var Coins : array 1 .. AMOUNT of Coin
for i : lower (Coins) .. upper (Coins)
Coins (i).Y := Rand.Int (maxy + RADIUS, maxy * 2)
Coins (i).X := Rand.Int (RADIUS, maxx - RADIUS)
Coins (i).Speed := Rand.Int (1, 5)
end for
View.Set ("offscreenonly")
loop
for i : lower (Coins) .. upper (Coins)
if Coins (i).Y <= -RADIUS then
Coins (i).Y := maxy + RADIUS
Coins (i).X := Rand.Int (RADIUS, maxx - RADIUS)
end if
Coins (i).Y -= Coins (i).Speed
Draw.FillOval (Coins (i).X, Coins (i).Y, RADIUS, RADIUS, yellow)
end for
View.Update
cls
end loop
|
|
|
|
|
|
 |
Martin

|
Posted: Mon Dec 19, 2005 7:27 pm Post subject: (No subject) |
|
|
Albrecd wrote: Note: the two for loops in the loop in my example could really be one... Why is there no edit button???
No edit button because people would ask for help, get their answer and then they'd edit their post and delete their question. Just post another reply. |
|
|
|
|
 |
chrispminis
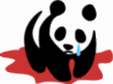
|
Posted: Mon Dec 19, 2005 10:44 pm Post subject: (No subject) |
|
|
Wow! Thx, a lot of good responses. Can't say I understand it all, but no worries, over the holidays ill teach it to myself. |
|
|
|
|
 |
chrispminis
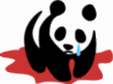
|
Posted: Tue Dec 20, 2005 10:31 pm Post subject: (No subject) |
|
|
Ok, Right now im trying to get it so that different coloured coins are worth different amounts of points. Unfortunately I haven't found a place for my
code: | cointype := Rand.Int (1, 200) | to go. Either it changes the value while its falling, or it has the same value forever. Here is the source and you get the gist of the game im trying to create.
code: | var mx, my, mb : int
var totalcoins : int
var score : real := 0
var scoredegree : real
var cointype : int
var coincol : int := 14
View.Set ("graphics:500;600,offscreenonly")
type attributes :
record
speed : int
xpos : int
ypos : int
end record
get totalcoins
var coins : array 1 .. totalcoins of attributes
var whenfall : array 1 .. totalcoins of int
var fall : array 1 .. totalcoins of boolean
var counter : int := 0
for i : 1 .. totalcoins
fall (i) := false
end for
for i : 1 .. totalcoins
whenfall (i) := Rand.Int (1, 5)
end for
for i : 1 .. totalcoins
coins (i).speed := Rand.Int (3, 4)
coins (i).xpos := Rand.Int (1, maxx)
coins (i).ypos := maxy - 50
end for
loop
Mouse.Where (mx, my, mb)
counter := counter + 1
cointype := Rand.Int (1, 200)
for i : 1 .. totalcoins
if whenfall (i) = counter then
fall (i) := true
end if
end for
for i : 1 .. totalcoins
if fall (i) = true then
if cointype < 150 then
coincol := 184
scoredegree := 0.01
elsif cointype >= 150 and cointype < 180 then
coincol := 25
scoredegree := 0.25
elsif cointype >= 180 and cointype < 190 then
coincol := 6
scoredegree := 1
elsif cointype >= 190 and cointype < 198 then
coincol := 30
scoredegree := 5
else
coincol := 14
scoredegree := 10
end if
coins (i).ypos -= coins (i).speed
Draw.FillOval (coins (i).xpos, coins (i).ypos, 5, 5, coincol)
end if
if coins (i).ypos <= 10 then
if coins (i).xpos >= mx - 50 and coins (i).xpos <= mx + 50 then
score := score + scoredegree
elsif coins (i).xpos < mx - 49 or coins (i).xpos > mx + 51 then
score := score - scoredegree
end if
end if
if coins (i).ypos <= 10 then
coins (i).speed := Rand.Int (3, 4)
coins (i).xpos := Rand.Int (1, maxx)
coins (i).ypos := maxy - 50
end if
end for
Draw.Line (1, maxy - 50, maxx, maxy - 50, black)
Draw.FillBox (mx - 50, 1, mx + 50, 10, black)
if score < 0 then
score := 0
end if
locate (1, 40)
put score
View.Update
delay (10)
cls
end loop
|
So please debug for me, would be great help. And i used albrects timing idea, and Carinos and do_pete's help with multiple falling (both of your ideas were essentially the same). |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
do_pete

|
Posted: Wed Dec 21, 2005 11:40 am Post subject: (No subject) |
|
|
Here's my above idea modified to do that
code: | type Coin :
record
X : int
Y : int
Speed : int
Colour : int
end record
const RADIUS := 5
const AMOUNT := 4
const PADDLE_LENGTH := 100
const PADDLE_WIDTH := 10
var Coins : array 1 .. AMOUNT of Coin
var MouseX, MouseY, MouseButton : int
var Score : int := 0
for i : lower (Coins) .. upper (Coins)
Coins (i).Y := Rand.Int (maxy + RADIUS, maxy * 2)
Coins (i).X := Rand.Int (RADIUS, maxx - RADIUS)
Coins (i).Speed := Rand.Int (1, 5)
Coins (i).Colour := Rand.Int (1, 16)
end for
View.Set ("offscreenonly")
loop
Mouse.Where (MouseX, MouseY, MouseButton)
for i : lower (Coins) .. upper (Coins)
if Coins (i).Y <= -RADIUS then
Coins (i).Y := maxy + RADIUS
Coins (i).X := Rand.Int (RADIUS, maxx - RADIUS)
Score := max (0, Score - Coins (i).Colour)
elsif Coins (i).Y <= PADDLE_WIDTH + RADIUS and Coins (i).X <= MouseX + PADDLE_LENGTH and Coins (i).X >= MouseX - PADDLE_LENGTH then
Coins (i).Y := maxy + RADIUS
Coins (i).X := Rand.Int (RADIUS, maxx - RADIUS)
Score += Coins (i).Colour
end if
Coins (i).Y -= Coins (i).Speed
Draw.FillOval (Coins (i).X, Coins (i).Y, RADIUS, RADIUS, Coins (i).Colour)
end for
Draw.FillBox (MouseX + PADDLE_LENGTH div 2, 0, MouseX - PADDLE_LENGTH div 2, PADDLE_WIDTH, black)
locate (1, 1)
put "Score: ", Score
View.Update
cls
end loop
|
|
|
|
|
|
 |
Albrecd
|
Posted: Wed Dec 21, 2005 12:52 pm Post subject: (No subject) |
|
|
You need to declare the type of coin at the beginning of the program [i]and[/a] with the code that determines what happens after the coin has finished falling. If you put it in the main loop, it will constantly change, and if you only declare it once, it will keep the same value for the entire program. |
|
|
|
|
 |
chrispminis
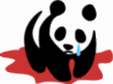
|
Posted: Wed Dec 21, 2005 10:35 pm Post subject: (No subject) |
|
|
Excellent, thx to do_pete and albrecd, used both of your ideas. Will credit you in comments  |
|
|
|
|
 |
|
|