need help in making the ball bounce in a parabolic
Author |
Message |
waleedalidogar
|
Posted: Wed Dec 14, 2005 9:21 pm Post subject: need help in making the ball bounce in a parabolic |
|
|
need help in making the ball bounce in a parabolic
loop % This tells the program to start the loop.
var x, y, c, i, r, p : int %This is a variable it makes a space in the memory for the x, y, c, i, r, p;
%I selected int because i know the input has to be in integers.
var n, e, s : string %This is a variable it makes a space in the memory for the n, e, s;
%I selected string because i know the input has to be in letters.
put "Welcome to Waleed's bouncing ball program" % This displays the text on the run screen.
put " " % This displays the text on the run screen.
put "What is your name?" % This displays the text on the run screen.
get n % This gets the input from the user for the n.
put "Hi, ", n % This displays the text on the run screen with the n.
put n, ", What colour do you want the ball to be?(give a number 1=Blue, 10=Green, 15=grey, 255=Black or a number between 1-255) " % This displays the text on the run screen with the n.
get p % This gets the input from the user for the p.
put n, ", How big do you want the ball to be?(1-50)" % This displays the text on the run screen with the n.
get r % This gets the input from the user for the r.
put n, ", How fast do you want the ball to move?(1-30)" % This displays the text on the run screen with the n.
get i % This gets the input from the user for the i.
x := 10 % This declares what x is.
y := 600 % This declares what y is.
var yLimit : int % This sets the yLimit which means that the highest point the ball can reach.
yLimit := 400 % This declares what the ylimit is for the next for loop.
for cnt : 1 .. 15 % This is a for loop; it sets cnt as a variable and tells it to do something 15 times.
y := yLimit % This declares what y is equal to; y=yLimit.
loop % This tells the program to loop itself.
drawfilloval (x, y, r, r, p) % This tells the program to draw an oval and fill it, in which all the measurements are given by the user.
delay (i) % This delays the program for i seconds; i is a variable.
drawfilloval (x, y, r, r, white) % This tells the program to draw an oval and fill it, in which all the measurements are given by the user.
x := x + 2 % This declares what x is.
y := y - 10 % This declares what y is.
exit when y <= 25 % This tells the loop to exit when y=<25.
end loop % This tells the program to end the loop.
yLimit := yLimit - 30 % This declares what the ylimit is for the next for loop.
loop % This tells the program to loop itself.
drawfilloval (x, y, r, r, p) % This tells the program to draw an oval and fill it, in which all the measurements are given by the user.
delay (i) % This delays the program for i seconds; i is a variable.
drawfilloval (x, y, r, r, white) % This tells the program to draw an oval and fill it, in which all the measurements are given by the user.
x := x + 2 % This declares what x is.
y := y + 10 % This declares what y is.
exit when y >= yLimit % This tells the loop to exit when y>= yLimit.
end loop % This tells the program to end the loop.
exit when cnt <= 0 % This tells the big loop to exit when the cnt <= 0
end for % This ends this for loop.
put "Congratulations ", n, ", you just made a bouncing ball" % This displays the text on the run screen with the n.
put n, " type exit to exit or press c" % This displays the text on the run screen with the n.
get e % This gets the input from the user for the e.
if e = "exit" then
exit % This exits the program.
end if % This ends this if.
put n, " type start to try again" % This displays the text on the run screen with the n.
get s % This gets the input from the user for the s.
put " " % This displays the text on the run screen.
delay (5) % This delays the program for 0.05 seconds.
cls % This clears the screen.
end loop % This tells the program to end the loop.
Description: |
|
 Download |
Filename: |
9 bouncing ball program.t |
Filesize: |
4.48 KB |
Downloaded: |
148 Time(s) |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Paul
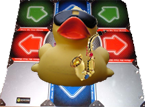
|
Posted: Wed Dec 14, 2005 9:37 pm Post subject: (No subject) |
|
|
Didn't I post in reply to your last topic in "turing tutorials"? You need to simulate gravity or use a parabolic equation. I find gravity to be the easiest way. Unfortunately I don't have enough time to type up that code again.
|
|
|
|
|
 |
McKenzie

|
Posted: Wed Dec 14, 2005 9:50 pm Post subject: (No subject) |
|
|
First, don't spam the comments. I know you are trying to make the program clearer and use good style. Only add comments that clarify the code. Stuff like "% This displays the text on the run screen." is only in the way.
For a parabolic arc there are two approaches, Math and Physics. I think the Physics approach is much cleaner. Consider the velocity of the ball as a vector. This vector has a horizontal and vertical component. After the initial velocity is established the horizontal component will not change without some interference (like a wall.) The vertical component will be affected by gravity. Because the scale is artificial don't worry about using 9.8 m/ss. Here is a simple example. No this is not an example of proper comment either. Some point in between is best.
code: | var x,y:=100.0
var dx,dy:real
dx:=5
dy:=20
loop
x+=dx
dy-=.5
y+=dy
if x>maxx or x< 0 then
dx*=-1
end if
if y< 0 then
dy*=-1
end if
cls
drawfilloval(round(x),round(y),10,10,4)
delay(20)
end loop
|
|
|
|
|
|
 |
Paul
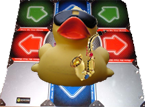
|
Posted: Wed Dec 14, 2005 9:58 pm Post subject: (No subject) |
|
|
Er actually, I didn't know cervantes moved it.
I would encourage you to stop making multiple posts of the same topic.
Here's my code again
code: |
setscreen ("graphics: max; max")
setscreen ("offscreenonly")
var Hspeed, Vspeed, Haccel, Vaccel, Posx, Posy, Decay: real
Hspeed:=3
Vspeed:=0
Haccel:=0
Vaccel:=-0.2%force of gravity
Posx:= 100
Posy:= maxy-200
Decay:= 0.75
loop
Draw.FillOval (round(Posx), round(Posy), 10, 10, brightred)
delay (10)
View.Update
Draw.FillOval (round(Posx), round(Posy), 10, 10, white)
Hspeed+=Haccel
Vspeed+=Vaccel
Posx+=Hspeed
Posy+=Vspeed
if Posy-11 <= 0 then
Posy:= 11
Vspeed:= -Vspeed*Decay
end if
end loop
|
Remove the second drawoval command to see the actual trajectory.
|
|
|
|
|
 |
waleedalidogar
|
Posted: Wed Dec 14, 2005 10:04 pm Post subject: can you please help me do my program with using parabola |
|
|
Paul wrote: Er actually, I didn't know cervantes moved it.
I would encourage you to stop making multiple posts of the same topic.
Here's my code again
code: |
setscreen ("graphics: max; max")
setscreen ("offscreenonly")
var Hspeed, Vspeed, Haccel, Vaccel, Posx, Posy, Decay: real
Hspeed:=3
Vspeed:=0
Haccel:=0
Vaccel:=-0.2%force of gravity
Posx:= 100
Posy:= maxy-200
Decay:= 0.75
loop
Draw.FillOval (round(Posx), round(Posy), 10, 10, brightred)
delay (10)
View.Update
Draw.FillOval (round(Posx), round(Posy), 10, 10, white)
Hspeed+=Haccel
Vspeed+=Vaccel
Posx+=Hspeed
Posy+=Vspeed
if Posy-11 <= 0 then
Posy:= 11
Vspeed:= -Vspeed*Decay
end if
end loop
|
Remove the second drawoval command to see the actual trajectory.
|
|
|
|
|
 |
md

|
Posted: Wed Dec 14, 2005 11:23 pm Post subject: (No subject) |
|
|
please don't quote someone without saying anything; it's just pointless.
|
|
|
|
|
 |
timmytheturtle
|
Posted: Thu Dec 15, 2005 6:42 am Post subject: (No subject) |
|
|
Cornflake, you should also have said: "waleedalidogar you the code tag"
|
|
|
|
|
 |
|
|