Author |
Message |
brokeded
|
Posted: Mon Nov 07, 2005 9:48 am Post subject: Help with Arrays in a Procedure... |
|
|
I am using Turing in my Computer Science class and we are currenly working on Procedures, and I need help on a question.
The question I have to do is:
Write a procedure that will output the largest element of an array of size N. The call to the procedure should be largestInArray( A, N).
(((( How would I do it? I am not to good with Procedures right now)))) |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
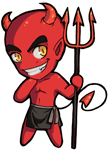
|
Posted: Mon Nov 07, 2005 9:51 am Post subject: (No subject) |
|
|
first - do you know how to do this without a procedure?
if so, then procedure is just a wrapper for that code, and instead of creating an array full of values, you're simply passing one as an argument. |
|
|
|
|
 |
codemage

|
Posted: Mon Nov 07, 2005 9:59 am Post subject: (No subject) |
|
|
If you're learning procedures, you should probably already know how to step through an array. You might want to take a look at the array tutorial for a refresher.
Your teacher probably expects you to step through using a loop and check each element against the biggest element you've found yet.
EDIT: Kind of redundant to Tony's post now. Took too long to respond with the reply window open.  |
|
|
|
|
 |
MysticVegeta

|
Posted: Mon Nov 07, 2005 2:47 pm Post subject: (No subject) |
|
|
Isnt this sorting?
Also, what kind of elements are you talking about? Strings or ints? There are lotsa tutorials for sorting the array. |
|
|
|
|
 |
beard0

|
Posted: Mon Nov 07, 2005 3:35 pm Post subject: (No subject) |
|
|
Given that your teacher wants you to use A and N as parameters, and Turing already has the upper function, are you sure that you aren't expected to write a function using recursion? You should check with your teacher. |
|
|
|
|
 |
[Gandalf]

|
Posted: Mon Nov 07, 2005 3:52 pm Post subject: (No subject) |
|
|
Well... the difference here is that you only need the largest element in the array, you don't have to worry about anything else. This makes it much easier.
Ah, almost didn't catch beard0's post. I doubt that at this level (also just learning procedures) that he knows about recursion. They might need to use N because they haven't covered upper() yet. |
|
|
|
|
 |
MysticVegeta

|
Posted: Mon Nov 07, 2005 7:50 pm Post subject: (No subject) |
|
|
I still support tony's post about how you know how to do it without using procedure. I dont think they have learned the procedure parameters yet so just have to wrap up that code in procedure i guess. |
|
|
|
|
 |
brokeded
|
Posted: Tue Nov 08, 2005 8:36 am Post subject: (No subject) |
|
|
beard0 wrote: Given that your teacher wants you to use A and N as parameters, and Turing already has the upper function, are you sure that you aren't expected to write a function using recursion? You should check with your teacher.
We have learned upper( ), but I am not to good at using it. Plus I can't use a Function because the next question tells me to do this same question but with a function. I just have to find a way to figure out the highest number, but the 'upper ( )' command always comfuses me. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
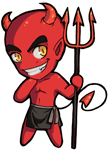
|
Posted: Tue Nov 08, 2005 8:54 am Post subject: (No subject) |
|
|
brokeded wrote: the 'upper ( )' command always comfuses me.
if your procedure is largestInArray( A, N) then upper(A) will be the same as N |
|
|
|
|
 |
brokeded
|
Posted: Tue Nov 08, 2005 8:58 am Post subject: (No subject) |
|
|
Alright, I got it to work. Here is the procedure I have, and it works, so I am happy.
Quote:
procedure largestInArray (var A : array 1 .. * of int, N : int)
var high : int := minint
for i : 1 .. N
high := max (high, A (i))
end for
put high
end largestInArray
Alright, for the next question, I have to:
Quote:
Modify the above procedure so that it is a function that returns the largest value in the array. No output is expected within the function. The function call might be: large := largestInArray(Array, N)
To bad I haven't even been taught functions yet. Now i have to go look throught the Turing Help post thing, and find functions and read up on how to use them.... gonna be a while  |
|
|
|
|
 |
codemage

|
Posted: Tue Nov 08, 2005 9:10 am Post subject: (No subject) |
|
|
Functions are friendly little guys; don't worry.
They're basically procedures that return back a value. |
|
|
|
|
 |
Tony
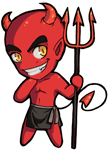
|
Posted: Tue Nov 08, 2005 9:17 am Post subject: (No subject) |
|
|
so instead of
put high
you'll have
result high
you should read up on functions and how they work |
|
|
|
|
 |
brokeded
|
Posted: Tue Nov 08, 2005 9:20 am Post subject: (No subject) |
|
|
Yeah, I got it. My friend in class is a wiz with Turing, and he helped me learn how to use Functions. So I am done that question and am on to the next. Here is what I got for my function, and it seems to work.
Quote:
function largestInArray (var A : array 1 .. * of int, N : int) : int
var high : int := minint
for i : 1 .. N
high := max (high, A (i))
end for
result high
end largestInArray
|
|
|
|
|
 |
[Gandalf]

|
Posted: Tue Nov 08, 2005 4:10 pm Post subject: (No subject) |
|
|
brokeded wrote: My friend in class is a wiz with Turing, and he helped me learn how to use Functions.
Then he should come here .
Here is the exact same thing, but using upper():
code: | function largestInArray (var A : array 1 .. * of int) : int
var high : int := minint
for i : 1 .. upper (A)
high := max (high, A (i))
end for
result high
end largestInArray |
If that example isn't enough, read some of the tutorials we have on arrays, they should be enough to clear things up for you...
One is:
http://www.compsci.ca/v2/viewtopic.php?t=1117 |
|
|
|
|
 |
GlobeTrotter
|
Posted: Tue Nov 08, 2005 4:54 pm Post subject: (No subject) |
|
|
high : int := minint
seems like a strange way to approach this problem. You're introducing needlessly large negative numbers. Why not set high equal to A(1) then loop from the 2nd to the last. |
|
|
|
|
 |
|