Boolean Algebra
Author |
Message |
Tony
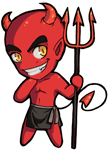
|
Posted: Tue Nov 22, 2005 4:05 pm Post subject: Boolean Algebra |
|
|
Boolean Algebra with Ruby
What is Boolean Algebra?
The Columbia Electronic Encyclopedia defined "Boolean Algebra" as "an abstract mathematical system primarily used in computer science and in expressing the relationships between sets (groups of objects or concepts)."
In essence, it's an expression that does a number of logical comparisons, such as and, or, not, on a set of objects and returns ether true or false.
What would be an example?
Ruby: |
puts "any fruit but orange" if apple.class == Fruit and apple.name != "orange"
|
Our apple object must be of Fruit class, but its name must not be "orange".
That seems simple enough..
The trick with Ruby, is to keep in mind the order of operations, what is calculated first, and what is not. That's correct, not every evaluation has to be made.
Back to our fruit example:
apple must be a fruit and not be named "orange"
What happens if someone is trying to trick us, and apple is not even a fruit to begin with?
Ruby: |
apple = Shoe.new(:name => "not orange")
|
That's crazy! A Shoe is not a Fruit. Now even if its name isn't "orange", there's no way it will satisfy both conditions. What happens? Ruby is not going to check for remainder of the conditions, once the result becomes obvious.
Oh really?
Yes, check this out
code: |
irb(main):001:0> foo
NameError: undefined local variable or method `foo' for main:Object
from (irb):1
irb(main):002:0> puts "apples are yummy" if true or foo
apples are yummy
=> nil
irb(main):003:0> puts "is foo true?" if true and foo
NameError: undefined local variable or method `foo' for main:Object
from (irb):3
|
foo is undefined. When evaluating
Ruby views it as "true or something_else". Reguardless of the outcome of that something_else, the statement is still going to be true, so Ruby doesn't bother with it. Good thing too, since if we were to compare them the other way (if foo or true), we would get an undefined error as we did earlier on.
What does this all mean?
As long as you keep the order in mind, you could do a variety of tricks.
Perform inline specific operations on abstract objects (no nested type checks):
Ruby: |
if mystery.isFruit? and mystery.eat then
"successfully ate a fruit"
else
"what are you trying to feed me?"
end
|
Optimization of code:
Ruby: |
apple.discard if apple.isRotten? or apple.complexOperation
|
apple will be discarded after a complexOperation has been successfully performed (such as eating the adable part). In case of apple being rotten though, it will be discarded right away, and we will not waste our time on unneccessary complex operations.
One thing to remember
The above could cause some elusive bugs as well. For example, the default structure in Rails for saving or updating models is as follows
Ruby: |
if @model.update_attributes(params[:model_name])
flash[:notice] = 'Model_name was successfully updated.'
redirect_to :action => 'show', :id => @model
else
render :action => 'edit'
end
|
If everything has updated correctly, show the result, otherwise go back to editing.
The problems appear when you have more than one model present on the screen, and both need to be updated. The intuitive guess to have
Ruby: |
if @model.update_attributes(params[:model_name]) and @another.update_attributes(params[:another_name]) |
would cause a bug to appear if the first @model fails to update. The second will not be attemped.
Replacing and with or will spawn another type of bug, as when the first model updates successfully, the second will not be tried.
You would have to take updates out of the conditional statement, save each result in a boolean flag, and compare those after every update has been executed. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Tue Nov 22, 2005 4:56 pm Post subject: (No subject) |
|
|
Excellent.
I would also add that this behavior is not peculiar to "if". It applies anywhere "and" or "or" are used to implement boolean logic. |
|
|
|
|
 |
|
|