Symbols
Author |
Message |
Cervantes

|
Posted: Sat Oct 15, 2005 9:36 pm Post subject: Symbols |
|
|
Symbols
"Curses and pandemonium!" you shout as your RAM meter rapidly rises to 100%. Rhythmbox stalls for a moment, destroying Mark Knopfler's solo in "Sultans of Swing". Nautilus stops responding. Firefox bites its own tail in a desperate search for energy. Yes, your precious Ruby program has become a hog, eating your RAM like there is no tommorow. And there may never be.
The Advantage of Symbols
So what can you do about it? As it turns out, Ruby has these little things called symbols. They are :words :with :eyes, as why would say. They behave much like strings, but they are stored differently.
Let's look at a very simple example. I will create an array of three strings, all of which are equivalent. Yet, despite being equal, they have different id's, which means that they live in their own spot in our RAM.
code: |
irb(main):001:0> strings = [ "name", "name", "name" ]
=> ["name", "name", "name"]
irb(main):002:0> strings.each { |s| puts s.object_id }
-605472036
-605472046
-605472056
=> ["name", "name", "name"]
|
So, each occurance of "name" lives on its own. Consider a more realistic example: a database of sorts. The database has an array of hashes to store client's names and email addresses. The hashes look something like this:
code: |
{ "name" => "John Blackthorne", "email" => "jblackthorne@osaka.com" }
|
This is one hash that is part of an array that contains many hashes. Each hash stores the same type of information, so "name" and "email" are part of every hash. Having "name" and "email" as strings will create many homes for these strings in our RAM, when really we could lump them all "names" together in the same location and all "emails" in another location. So let's switch to using symbols.
Symbols are denoted by a colon ( : ) in front of the variable name. As I said earlier, they are :words :with :eyes. Symbols have their own class, so you won't be able to use methods of the String class on them. More on that later. Now let's look at the first example, except using symbols:
code: |
irb(main):003:0> strings = [ :name, :name, :name ]
=> [:name, :name, :name]
irb(main):004:0> strings.each { |s| puts s.object_id }
880910
880910
880910
=> [:name, :name, :name]
|
Success! Each occurance of :name is stored in the same location in the RAM. Thus, if we were to use :name and :email instead of "name" and "email" in the example of an array of many hashes, we would save lots of space. That is a good thing.
Usage of Symbols
So far we've discussed the advantage of symbols over strings. Of course, symbols have a downside as well. They are more difficult to work with. The Symbol class does not have very many methods to it. If you want to do string manipulation on symbols, you'll first have to convert it to a string. This is achieved by calling the to_s method:
code: |
irb(main):001:0> :foo.to_s
=> "foo"
|
So now we can use string methods.
code: |
irb(main):002:0> :foo.to_s.upcase
=> "FOO"
|
What about dynamically creating symbols?
code: |
irb(main):003:0> "foo".to_sym
=> :foo
|
code: |
irb(main):004:0> :foo.to_s.upcase.to_sym
=> :FOO
|
We can also create multi-word symbols:
code: |
irb(main):005:0> :"Quicksilver Messenger Service"
=> :"Quicksilver Messenger Service"
|
code: |
irb(main):006:0> :"Quicksilver Messenger Service".to_s.squeeze.to_sym
=> :"Quicksilver Mesenger Service"
|
Note the missing 's' in "Messenger".
I hope this helps to clarify symbols. If it doesn't, my apologies. Perhaps this will explain it better: http://glu.ttono.us/articles/2005/08/19/understanding-ruby-symbols. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Sat Oct 15, 2005 10:38 pm Post subject: (No subject) |
|
|
Indeed. Imagine parsing a log file. The log file is a flat text file where each line represents a record. Each piece of data in this is represented as a name/value pair.
Now... imagine there are two million lines in the file. Represent each name as a symbol and save loads of memory.  |
|
|
|
|
 |
Tony
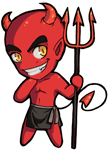
|
Posted: Sun Oct 16, 2005 4:46 pm Post subject: (No subject) |
|
|
you would usually use symbols when you need a meaningfull name, but don't intend to display that name to the user. |
|
|
|
|
 |
|
|