[Tutorial] Modules
Author |
Message |
Delos

|
Posted: Sun Mar 28, 2004 6:14 pm Post subject: [Tutorial] Modules |
|
|
MODULES: A TUTORIAL
Hello all, and welcome to this tutorial on the ins and outs of modules! I'm sure you're all just itching to get started, so let's.
What is a module?
A module is basically a collection or package of a number of procedures, functions, routines etc etc. Modules are particularly useful for packaging algorithms that can be used over and over again.
Not all the contents of a module can be accessed from outside of it. Which can and which can't is up to the programmer of that module. Often, just a few elements of the module are exported, while the many others within it are used by the commands within those elements.
What does a module look like?
Here is a simplified version of modular declarations. Some aspects have been omitted due to either their rarity or the lack of experience with them to aptly describe them.
module moduleName
export export1 (,"¦)
(
procedure notExported
-contents
end notExported
)
procedure export1
-contents
end export1
end moduleName
A Step-By-Step Guide Through The Development Of A Module
Step 1:
Of course one needs and idea of sorts before coding begins. This may be in the form of pseudo code, thoughts, whims, etc. For this demonstration, we shall be making a simple string manipulator.
Our idea is to make an importable module that can do 3 things to text:
- turn it UPPER CASE
- turn it lower case
- turn it to aLtErNaTiNg CaSe
Step 2:
Begin the coding. We know that we will have 3 main procedures/functions that we will be exporting. For this demonstration, a bit of a lengthier and less inefficient means of coding will be used simply to show how modules may incorporate various containers.
code: |
module textMan
export toUpper, toLower, alt
procedure toUpper (var text : string)
end toUpper
procedure toLower (var text : string)
end toLower
procedure alt (var text : string)
end alt
end textMan
|
At the moment we have 3 stubs - declared procedures without any contents within them. This is generally a good way to go about programming with modules as you will know exactly what is needed for each, which are done, which need work, etc, etc.
(Don't forget to save. We're going to call this file "textMan.t". What a coincidence.)
Step 3:
Continue coding.
For this demonstration the 3 exported procedures will not have any of the algorithms in them. The manipulation will be carried out by functions, as previously stated, to show the module's versatility.
This will be added to the file after the export list.
code: |
function makeUpper (var text : string) : string
var temp : string := ""
% Temporary variable used so as not to alter
% 'text' until the final step.
for i : 1 .. length (text)
if ord (text (i)) >= 97 and ord (text (i)) <= 122 then
temp := temp + chr (ord (text (i)) - 32)
else
temp := temp + text (i)
end if
end for
result temp
end makeUpper
function makeLower (var text : string) : string
var temp : string := ""
for i : 1 .. length (text)
if ord (text (i)) >= 65 and ord (text (i)) <= 90 then
temp := temp + chr (ord (text (i)) + 32)
else
temp := temp + text (i)
end if
end for
result temp
end makeLower
function makeAlt (var text : string) : string
var temp : string := ""
var charTemp : string := ""
for i : 1 .. length (text)
charTemp := text (i)
if i mod 2 = 1 then
temp += makeLower (charTemp)
else
temp += makeUpper (charTemp)
end if
% This for loop alternates between capitalizing
% and lowering cases within the word.
% Note how previously declared fucntions are
% employed.
end for
result temp
end makeAlt
|
Step 4:
Time to connect the functions to the modules. This is relatively simple. The exportable procedures are altered thus:
code: |
procedure toUpper (var text : string)
text := makeUpper (text)
end toUpper
procedure toLower (var text : string)
text := makeLower (text)
end toLower
procedure alt (var text : string)
text := makeAlt (text)
end alt
|
Step 5:
Now, to access the module. There are 2 ways to do this. The one that really doesn't make much sense, and the one that does make sense.
1st way: Accessing from the same file as the module.
To do this, we need to reference the module, followed by a dot, then the procedure in question's reference:
code: |
var testWord : string := "thequickBROWNfoxJuMpsovertheLazyDoG"
textMan.toUpper (testWord)
put testWord
textMan.toLower (testWord)
put testWord
textMan.alt (testWord)
put testWord
|
This is put after the end of the module. Notice in the output. To get the words to screen, we had to use put commands. This is because the module itself is simply a tool. It does nothing more that change the variables in question. It did no other alterations to the programme. This is why it is so important to ensure that no extraneous code exists (i.e. code that is not contained by a procedure, etc. The exception is for the declaration variables etc local to the module). This can have its advantages and drawbacks. But that is up to you to find out.
2nd way: Accessing the module from another file. This makes far more sense as modules are nice and neat packages that we want to get to from another file.
To do this, two alterations have to be made to the file containing the file. First, to the beginning of the file, we must add the key word unit, like thus:
Next, we must resave the file but under the name "textMan.tu" as opposed to the regular .t extension. Why is this important? Well, it's not essential but it makes your life quite some easier.
We will now open a new file and import out module unit.
code: |
import textMan
var test : string := "QWERTY"
textMan.toLower (test)
put test
|
Here's a little side note. If you don't want to resave your file as .t, then do this. Make sure you have the 'unit' keyword at the top of the file as usual. Then:
code: |
import textMan in "textMan.t"
|
Also note that files with the unit header cannot be run directly. I.e., you cannot open textMan.tu and press F1. Try it, you'll see.
And there you have it folks. A brief look at modules. The more you use them, the more you'll like them. Of course there are several other aspects which have not been touched upon, such as pre and post conditions, implementation, and deference. But these are not used very often for the average programmer. Of course if you are in the position to be needed to use them, you'd be better off figuring them out for yourself.
To end off, here is the final module we have developed:
code: |
unit
module textMan
export toUpper, toLower, alt
function makeUpper (var text : string) : string
var temp : string := ""
% Temporary variable used so as not to alter
% 'text' until the final step.
for i : 1 .. length (text)
if ord (text (i)) >= 97 and ord (text (i)) <= 122 then
temp := temp + chr (ord (text (i)) - 32)
else
temp := temp + text (i)
end if
end for
result temp
end makeUpper
function makeLower (var text : string) : string
var temp : string := ""
for i : 1 .. length (text)
if ord (text (i)) >= 65 and ord (text (i)) <= 90 then
temp := temp + chr (ord (text (i)) + 32)
else
temp := temp + text (i)
end if
end for
result temp
end makeLower
function makeAlt (var text : string) : string
var temp : string := ""
var charTemp : string := ""
for i : 1 .. length (text)
charTemp := text (i)
if i mod 2 = 1 then
temp += makeLower (charTemp)
else
temp += makeUpper (charTemp)
end if
% This for loop alternates between capitalizing
% and lowering cases within the word.
% Note how previously declared fucntions are
% employed.
end for
result temp
end makeAlt
procedure toUpper (var text : string)
text := makeUpper (text)
end toUpper
procedure toLower (var text : string)
text := makeLower (text)
end toLower
procedure alt (var text : string)
text := makeAlt (text)
end alt
end textMan
|
Et, ça c'est le fin! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
shorthair

|
Posted: Sun Mar 28, 2004 10:07 pm Post subject: (No subject) |
|
|
Dude + 100 bits
Id read it but i know it ,
You put Uber amounts of effort into this , but if i find out its not your tuturial , its -200 bits for you ,
Thanks For contributing to te Forums Delos , THis is a clean and Well Layed out turtorial , its easy on the eyes , Well Done again |
|
|
|
|
 |
recneps

|
Posted: Mon Mar 29, 2004 4:00 pm Post subject: (No subject) |
|
|
Wow. Bravo. Now all we need is the compsci community to make its own turing commands because holtsoft is too slow +BITS
C'est très bien Delos, tu dois continuer à créer des instructions bons!!
Edit: Ajouter 26 bits pour avoir un rond 500! |
|
|
|
|
 |
Tony
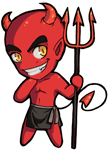
|
Posted: Mon Mar 29, 2004 4:34 pm Post subject: (No subject) |
|
|
except that external does not yet allow linkage to dll files It's kind of in the documentation but not really... its one of those "to be implemented" things.
So we can't really optimize turing's commands since we'd have to use turing's core anyways. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
AsianSensation
|
Posted: Mon Mar 29, 2004 8:18 pm Post subject: (No subject) |
|
|
nice nice....+bits |
|
|
|
|
 |
wtd
|
Posted: Sat Sep 25, 2004 7:34 pm Post subject: (No subject) |
|
|
Agh!
Someday the world will realize the evils of procedures and learn to embrace functions. Mutable state is something that should be avoided when possible.  |
|
|
|
|
 |
atrain
|
Posted: Tue May 17, 2005 4:46 pm Post subject: (No subject) |
|
|
so what does the funtion do??
can i just stick everything in a process and go away happy?? |
|
|
|
|
 |
Cervantes

|
Posted: Tue May 17, 2005 5:03 pm Post subject: (No subject) |
|
|
atrain wrote: so what does the funtion do??
can i just stick everything in a process and go away happy??
Dear lord, no! Not another process! :gagingSmiley:
Excuse me. What function are you asking about? Or, are you asking about functions in general? In any case, this sounds like a discussion for the Turing Help forum. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Mistro
|
Posted: Wed Jun 01, 2005 6:32 pm Post subject: (No subject) |
|
|
Hi, I would like to start by saying that I am quite new at Turing modules and external files. I have searchd for the issue on the forums and i have not found an answer which is why im posting this here since it made sense.
Lets say i have two modules. How would i go about importing them into my program? It would probably make sense to do soemthing like:
code: |
import (modulename in "filename")
import (modulename2 in "filename2")
|
However this generates an error
This occurs whenever i import more that two modules. They do not even have to by my own. I also tried to import "GUI" but it also failed when it was second on the list.
I then tried putting GUI first, and the error came up but this time on the second "import" which was my file that i had created.
Some help would be appreciated.
The error is generated at compile.
The compiler highlights the second word "import" alone
The error message says "syntax error at import)
here is the exact code:
code: | import (DragStrip in "dragstripModule.tu")
import GUI |
this generates an error for the second import (the one for GUI)
when flipped like so:
code: | import GUI
import (DragStrip in "dragstripModule.tu") |
the error occurs on the second import again (this time my own module) |
|
|
|
|
 |
Delos

|
Posted: Wed Jun 01, 2005 6:57 pm Post subject: (No subject) |
|
|
Turing: |
import GUI, DragStrip in "dragstripModule.tu"
|
You might get a warning popping up there. Import the entire module instead, safer. Easy, wasn't that.
Also, this is the Tutorials section. In future, please post questions in the Help forum, which could be accompanied by a link to the applicable Tutorial if the case might be. |
|
|
|
|
 |
Italian[Boy]

|
Posted: Wed Oct 31, 2007 3:52 pm Post subject: Re: [Tutorial] Modules |
|
|
wow, this was really helpful thanks so much, I really needed it for the mario game I'm making, props  |
|
|
|
|
 |
darkangel

|
Posted: Wed Jan 16, 2008 2:02 am Post subject: Re: [Tutorial] Modules |
|
|
lol, I'd rather just stick to my Str.Upper and my Str.Lower its just easier
but ya, decent tutorial |
|
|
|
|
 |
ericfourfour
|
Posted: Wed Jan 16, 2008 4:48 pm Post subject: RE:[Tutorial] Modules |
|
|
It is generally frowned upon, on this forum, to revive an old thread unless you have something worthwile to add.
For a bit more information, look into the predefined modules ("Support\predefs"). The Str module is structured the same way as in this guide.
This is an observation I just had. The Upper and Lower functions work very much like a finite state machine. Each character is either upper or lower case. When you call Upper on an upper case character nothing happens. When you call it on a lower case character, it switches to upper case. It is similar for the Lower function.
To expand a string manipulation module, a capitalize function can be added. Adding functions that interpret regular expressions (a.k.a. regex or regexp) would be handy as well. |
|
|
|
|
 |
|
|