[Tutorial] SWING -- The Basics
Author |
Message |
rizzix
|
Posted: Fri Sep 26, 2003 7:48 pm Post subject: [Tutorial] SWING -- The Basics |
|
|
SWING -- Lightweight GUI Framework
What's the deal with SWING?
All components in SWING are written in Java. Hence it's called a lightweight GUI framework. This brings about consistancy in terms of look & feel as compared to AWT. AWT on the other hand is tied to native libraries of that system (hence it's called a heavyweight GUI framework). So AWT in windows gives a different look & feel than the AWT on a Macintosh system. Although the SWING components are written in Java, it finally does interact with the underlying AWT framework to do the actual displaying or drawing of the components.
Why use SWING?
One reason to use SWING is consistancy in terms of look and feel. But another important reason is that the SWING framework is a lot more complete as compared to the AWT framework.
And the looks...?
Yes I'd have to agree. The default SWING PLAF (Plugable Look And Feel) called 'Metal', is not the best. It's not as good looking as the mordern "3D widget" PLAFs out there. I'm sure the JCP (Java Community Process) is working on a more mordern, standard PLAF for SWING, but it's not released yet.
That does not mean you are stuck to the Metal theme. You can use system specific themes, that some vendors provide for some Operating Systems. Windows has it's own PLAF and so does the Macintosh.
Since the themes are plugable, all you need to do is plugin in a new PLAF and you get a new look and feel. The system specific themes are available only on specific systems. So using these themes on other systems, will get SWING to switch back to the default theme (Metal).
Are there any other GUI frameworks?
Yes there are. AWT (Abstract Windowing Toolkit) as I said is the heavyweight GUI framework for java. It directly communicates with the underlying operating system. One thing is for sure AWT is not as complete as SWING.
The other framework I'm aware of is written by IBM, called SWT. It actually is really good in terms of looks and has a feel as that of apps written in windows. So yes, not an excellent portability choice in terms of feel, otherwise it's great.
So lets SWING!
This is the basic structure of a SWING app: An Application encapsulating a Frame, which contians Panes, to which you add Components, of which you may optionally add Event Listener(s) to.
Let's define the above terms.
Components:
Are really any object that you can see, like a button, textbox, a window (frame), etc. There are two different kinds of components: those that are containers and those that are not. The containers have the ability to contain other components, that means it can also contain other containers, since a container is a type of component.
Frame:
Is a component that is a container. It is the root container. It contains 4 different panes: root, layered, content, and glass pane. Only root containers contain panes. So this is different, you don't really add components to the container directly but to the panes. Usually components are added to the content pane. The glass pane is used for animation and stuff.
Event Handlers:
Are special objects that are specific to components. You add a event handler to a component. When an event is triggered that event handler object handles it. You add a event handler to a component that can accept that particular event handler. You may add a particular event handler to more than one component.
Application:
This is the class that includes the public static void main(String[] args) method. It is responsible to setup the frame, get it visible and contain application specific properties.
The example:
Java: |
import javax.swing.*;
import java.awt.Dimension;
public class Test {
public static void main (String[] args ) {
// initialised with the title for the title bar
JFrame frame = new JFrame("Test Application");
Dimension screenSize = frame. getToolkit(). getScreenSize();
frame. getContentPane(). add(new JLabel("Hello World!"));
frame. setBounds(screenSize. width/ 4, screenSize. height/ 4,
screenSize. width/ 2, screenSize. height/ 2);
frame. setDefaultCloseOperation(JFrame. EXIT_ON_CLOSE);
frame. setVisible(true);
}
}
|
Lets explain what's been done up there.
We first create a JFrame (a frame component) and get frame (the reference) to point to the object.
A Dimension object is used in SWING and AWT GUI frameworks to represent the dimensions of a window or your desktop screen. So thats exactly what we do next. We get the dimensions of the viewable area of the monitor.
What happens next (and happened a statement before) are chain method calls. We first call getContentPane() of the JFrame object, which returns the content pane object. We then call the add(Component com) method of the content pane object and pass it a newly created component: JLabel, which we initialised its text to 'Hello World!'. Yea i know we did a lot in that one statement. But you could have broken it down into smaller steps. It's just that I wanted to show you, that you can do a chain call, which makes coding quicker.
Next we call the public void setBounds(int x, int y, int width, int height) and the first two arguments is the top left position of the frame to be positioned in the desktop (where [0,0] is the top left corner of the screen). The next two arguments are the width and height of the frame.
Next we show the frame component. by default all the components in the frame are set to visible. but unitl the parent (the frame) is visible none will display.
Note: we haven't added an event handler to any component.
Thats all for the basics.. More to come. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
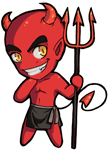
|
Posted: Fri Sep 26, 2003 8:49 pm Post subject: (No subject) |
|
|
awesome intro rizzix
very well explained Can't wait for event handlers to actually make those buttons work  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
krishon
|
Posted: Fri Sep 26, 2003 9:30 pm Post subject: (No subject) |
|
|
definitely good...u can write ur own book with all these tuts u make my god |
|
|
|
|
 |
the_short1
|
Posted: Fri Apr 08, 2005 1:44 pm Post subject: (No subject) |
|
|
TY! TY! TY!.. awsome tut.. i was looking in the SUN help section dealy for GUI.. and its just so FUWKED UP and bloated. . never cut and dry.. i really like your tutorials .. . make more .. ... .. . . please..  |
|
|
|
|
 |
1of42
|
Posted: Wed Apr 20, 2005 7:51 pm Post subject: (No subject) |
|
|
I like the sound of a default PLAF for Windows - how do I set it up to make Swing use that one? The default is fugly. |
|
|
|
|
 |
rizzix
|
Posted: Wed Apr 20, 2005 11:27 pm Post subject: (No subject) |
|
|
ehm its as simple as this:
btw: the 1.5 jre comes with a better default PLAF called 'Ocean'. |
|
|
|
|
 |
Martin

|
Posted: Sat Apr 23, 2005 7:47 am Post subject: (No subject) |
|
|
Wow these syntax tags are really useful.
Amazing job Rizzix. I'd give you bits, but you'd probably just spend them on alcohol. |
|
|
|
|
 |
Aziz

|
Posted: Sat Jul 02, 2005 3:01 pm Post subject: (No subject) |
|
|
Great. Thanks, helped me a lot
Btw, I got a "using depreceated API" message in the first example. This is what the API says is supposed to be used now
Java: | import javax.swing.*;
import java.awt.Dimension;
public class Test {
public static void main (String[] args ) {
// initialised with the title for the title bar
JFrame frame = new JFrame("Test Application");
Dimension screenSize = frame. getToolkit(). getScreenSize();
frame. getContentPane(). add(new JLabel("Hello World!"));
frame. setBounds(screenSize. width/ 4, screenSize. height/ 4,
screenSize. width/ 2, screenSize. height/ 2);
frame. setDefaultCloseOperation(JFrame. EXIT_ON_CLOSE);
frame. setVisible(true);
}
} |
MOD Edit: yes, i wrote that tutorial in 1.4.. 1.5 has deprecated the .show() method. i was to change it, but.. anyways thanks for brining it to my attention.
-- rizzix
+10 bits |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
the_short1
|
Posted: Sat Jul 02, 2005 9:40 pm Post subject: (No subject) |
|
|
yea. i must say .. having tag identifiers with colors and everything makes it a LOT easier to understand code..
well rizix.. if all goes good soon you wont be the only one making kick ass tuts.. mine will never be as good as yours.. but hopefully they will kick some ass  |
|
|
|
|
 |
rizzix
|
Posted: Tue Sep 06, 2005 4:34 pm Post subject: (No subject) |
|
|
for those i interested here's a some pretty good extention of the synth PLAF (a skinable look and feel that comes with Java SE 5): http://www.javasoft.de/jsf/public/products/synthetica/themes (note: you have to download the synthetica PLAF before you use those themes)
the QuaQua PLAF rectifies problems with Apple's default implementation of the Aqua look and feel: http://www.randelshofer.ch/quaqua/download.html (note: can only be used on Mac OS X 3.x and greater. also make sure you take a look at this) |
|
|
|
|
 |
JMG
|
Posted: Fri Feb 15, 2008 10:13 am Post subject: RE:[Tutorial] SWING -- The Basics |
|
|
awsome tut. thanx a ton. way better than how my crappy CS teacher explains things XD |
|
|
|
|
 |
jacklarry
|
Posted: Wed Feb 20, 2008 10:28 pm Post subject: RE:[Tutorial] SWING -- The Basics |
|
|
thnx for the code...it was helpful |
|
|
|
|
 |
shadowman544

|
Posted: Mon May 26, 2008 8:42 pm Post subject: RE:[Tutorial] SWING -- The Basics |
|
|
thanks guys |
|
|
|
|
 |
|
|