[Tutorial] Hello World - The Java Way
Author |
Message |
rizzix
|
Posted: Wed May 28, 2003 4:19 pm Post subject: [Tutorial] Hello World - The Java Way |
|
|
create a new file called HelloWorld.java
type this in...
Java: |
class HelloWorld {
public static void main (String[] args ) {
System. out. println("Hello World");
}
}
|
compile the file in to a .class file (bytecode) like this:
in the terminal
cd to_the_current_working_directory
javac HelloWorld.java
this will create a HelloWorld.class file
to run the HelloWorld.class file do this...
java HelloWorld
note : u do not type in the extension
it should run
------------------------------------------------------------------------------------
this defines and declares a class named HelloWorld
(note the class should have the same name as the .java file it is in, without the .java appended to it, or visa-versa)
Java: |
public static void main (String[] args ) {
|
this line declares the main procedure. C++ programmers will find this fimiliar. The main procedure of a class is the one that the JVM first calls when executing the .class file.
it must always have a return type of void (which means it returns nothing) and must be decalred public
and it has to be decalred static as well, since...
the JVM can only call a static procedure of a class, and not one associated with an object of that class (methods).
if the JVM was to call a method of an object, then how is that object to be created and instantiated in the first place? This is why static methods (methods that are not specific to object instances) are called, which can in turn create and instantiate objects of various kinds and set them to interact with each other!
static methods (procedures), are common to all objects of a class.
you can even call them directly from the class they belong to, ex:
Java: |
System. out. println("Hello World");
|
they do not change the state of an object, i.e they do not, and cannot modify members (variables) of an object.
now you might be wondering what the System.out is all about.
Well really the out is a static member object of type OutputStream of the class System.
(btw: out is technically a reference to an object of type OutputStream, we just call all variables/refrences, members in Java, but they are either members of instances of that class or of the class it self, depending on whether they are declared static or not)
So now that I have explained all about static members and methods, you might be wondering how to create an object.
first let me give u a brief overview on what a class really is:
A class is a template for an object. i.e u can think of them as cookie cutters, from it you can create objects, just as the cookie cutter can be used to create cookies!
Classes may have static members: these are methods and variables that are common to all objects (instances of that class) and specific to that class only. The member is freely accessible within the scope of that class and its objects only.
To call it outside the class you need to use the form:
Class.method();
Class.variable = "value";
Or if you have created an object of that class, you can also use this form:
object.method();
object.variable = "value";
where method() and variable are static members of the class. This and the above syntax are identical.
Classes may also have instance methods/members: these are methods and variables that are specific only to the objects created from that class. Static members of a class can not freely call an instance member of an object, since instance methods of objects affect the state of an object (i.e: they modify that perticular objects variable's values).
To create an instance member means ur defining an object.
Lets take a look at an example.
create a class called TestObject (hence the file should be named: "TestObject.java"), like this...
Java: |
class TestObject {
String the_string;
public void setString (String str ) {
the_string = str;
}
public String getString () {
return the_string;
}
}
|
note that we do not declare them static since they are instance members of a class.
also note: parenthesis are necessay even if there are no arguments passed to methods (procedure/functions). (Java is a strict typed language)
now lets use this class. So, back to our HelloWorld class... modify it...
Java: |
import TestObject;
class HelloWorld {
public static void main (String[] args ) {
TestObject obj;
obj = new TestObject ();
obj. setString("Hello World");
System. out. println(obj. getString());
}
}
|
make sure both files are in the same directory. compile it and run:
cd to_current_working_directory
javac HelloWorld.java
java HelloWorld
it should work.
note: all classes linked directly or indirectly to the HelloWorld class are automatically compiled. cool eh!
we declared a refrence (also called a handle in Java) to an object of type TestObject like this:
the refrence obj does not point or refere to any object at the moment. It is simply declared. And has the default value of null which means it refers to nothing!
We then create a new instance of an object of type TestObject, and get obj to refer to it, like this:
Java: |
obj = new TestObject();
|
you might be wondering why the TestObject(); method is used, when we haven't declared one.
Well.. the TestObject() method is a special kind of method called a Constructor (they are decalred with the same name as the class, and have no return type, No. Not even void!). It is used to initialize members of an object before creating an instance of it. In our case, we use the default Constructor. It is the one that is automatically created by the compiler, when you don't define one yourself. We didn't have to created a constructor in our case since we have no need of initializing any member of that class instance (object) at runtime.
the new keyword creates an instance of the class, it then calls the constructor for that object to initialize its members. after that it gets the refrence, obj to point to the newly created object.
everything else is pretty straight forward.
the get/set mehods (just a naming convention used by Java programmers) set a value to the String member, and returns the String member. (strings are kinda wierd in java, more on that in the next tutorial)
the System.out.println(String arg); method take a string object as its arguments. so we pass it the String object to use:
Java: |
System. out. println(obj. getString());
|
so thats basically it.
o btw: there are no pointers in Java, only refrences. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Asok

|
Posted: Wed May 28, 2003 5:06 pm Post subject: (No subject) |
|
|
Moved because this is a tutorial.
Anyways very nice job +10 bits |
|
|
|
|
 |
Tony
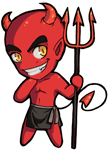
|
Posted: Wed May 28, 2003 5:37 pm Post subject: (No subject) |
|
|
Actually its an amazing tutorial... very detailed and gives nice examples and explanations. Also discusses the structure of the language
+25Bits on top of Asok's |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
rizzix
|
Posted: Wed May 28, 2003 5:38 pm Post subject: (No subject) |
|
|
thanks!! |
|
|
|
|
 |
Dan
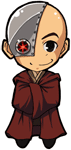
|
Posted: Wed May 28, 2003 6:35 pm Post subject: (No subject) |
|
|
i whould like to add that you can use just print(); insted of println(); if you whont it to saty on the same line like cout.
and make shure you name those files right  |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
|
|