Author |
Message |
Martin

|
Posted: Thu Aug 19, 2004 5:46 pm Post subject: The Other Official "Hello World" Thread. |
|
|
Let's make the hello world program in as many languages as possible.
Turing
C++
code: | #include <iostream>
int main()
{
std::cout << "Hello, world!\n";
return 0;
} |
Continue! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Mazer

|
Posted: Thu Aug 19, 2004 6:48 pm Post subject: (No subject) |
|
|
I'd like to start off by pointing out you've forgotten to return 0. Darkness. Tsk tsk.
EDIT: seems I've forgotten some code here.
Python
code: |
print "Hello, World!"
|
|
|
|
|
|
 |
wtd
|
Posted: Thu Aug 19, 2004 7:08 pm Post subject: (No subject) |
|
|
Ruby:
code: | STDOUT << "Hello world" << "\n" |
code: | $stdout << "Hello world" << "\n" |
O'Caml:
code: | print_endline "Hello world" |
C:
code: | #include <stdio.h>
int main()
{
printf("Hello world\n");
return 0;
} |
code: | #include <stdio.h>
int main(int argc, char * argv[])
{
printf("Hello world\n");
return 0;
} |
Perl:
code: | print "Hello world\n" |
code: | print STDOUT "Hello world\n" |
Eiffel:
code: | class HELLO_WORLD
creation make
feature { ANY }
make is
do
std_output.put_string("Hello world")
std_output.put_new_line
end
end |
Java:
code: | import java.lang.*;
import java.io.*;
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello world");
}
} |
Groovy:
code: | println "Hello world" |
PHP:
code: | <?php echo "Hello world" ?> |
Javascript:
code: | <script type="text/javascript">
document.write("Hello world<br/>");
</script> |
Pike:
code: | int main()
{
write("Hello world!\n");
return 0;
} |
code: | int main(int argc, array(string) argv)
{
write("Hello world!\n");
return 0;
} |
Haskell:
code: | main = print("Hello World") |
Icon:
code: | procedure main (args)
write ("Hello World")
end |
C#:
code: | using System;
public class HelloWorld {
public static void Main(string[] args) {
Console.WriteLine("Hello world");
}
} |
AppleScript:
code: | on run
display dialog ("Hello world" as text) buttons {"OK"}
end run |
|
|
|
|
|
 |
wtd
|
Posted: Thu Aug 19, 2004 7:35 pm Post subject: (No subject) |
|
|
Object-oriented versions here. I create a Greeter class in each language that takes a name (in this case simply "world") and greets it with "Hello".
Ruby:
In file "greeter.rb":
code: | class Greeter
def initialize(name)
@name = name
end
def greet
puts greeting
end
private
def greeting
"Hello #{name}"
end
attr_reader :name
end |
In file "main.rb":
code: | require "greeter"
hw = Greeter.new("world")
hw.greet |
O'Caml:
code: | class greeter name =
object(self)
method private greeting = "Hello " ^ name
method greet = print_endline self#greeting
end
let hw = new greeter "world" in hw#greet |
Objective-C:
In file "Greeter.h":
code: | #import <objc/Object.h>
@interface Greeter : Object
{
char * name;
}
- initWithName: (const char *) initName;
- (const char *) getName;
- (const char *) greeting;
- (void) greet;
@end |
In file "Greeter.m":
code: | #import "Greeter.h"
#include <stdio.h>
#include <string.h>
@implementation Greeter
- initWithName: (const char *) initName
{
[super init];
name = (char *)malloc(sizeof(char *));
strcpy(name, initName);
return self;
}
- (const char *) getName
{
return name;
}
- (const char *) greeting
{
char * output = (char *)malloc(sizeof(char *));
strcpy(output, "Hello ");
strcat(output, [self getName]);
return output;
}
- (void) greet
{
printf("%s\n", [self greeting]);
}
@end |
In file "main.m":
code: | #import "Greeter.h"
#include <stdio.h>
#include <string.h>
int main()
{
Greeter * hw = [[Greeter alloc] initWithName: "world"];
[hw greet];
} |
Perl:
In file "Greeter.pm":
code: | package Greeter;
use strict;
use warnings;
sub new {
my $class = shift;
my $name = shift;
my $self = {"name" => $name};
return bless $self, $class;
}
sub name {
my $self = shift;
return $self->{"name"};
}
sub greeting {
my $self = shift;
return "Hello " . $self->name;
}
sub greet {
my $self = shift;
print $self->greeting . "\n";
}
1; |
In file "main.pl":
code: | use Greeter;
use strict;
use warnings;
my $hw = new Greeter("world");
$hw->greet; |
Eiffel:
In file "greeter.e":
code: | class GREETER
creation with_name
feature { ANY }
with_name(init_name : STRING) is
do
name := init_name
end
greet is
do
std_output.put_string(greeting)
std_output.put_new_line
end
feature { NONE }
name : STRING
greeting : STRING is
do
Result := "Hello " + name
end
end |
In file "hello_world.e":
code: | class HELLO_WORLD
creation make
feature { ANY }
make is
local
hw : GREETER
do
create hw.with_name("world")
hw.greet
end
end |
Java
code: | import java.lang.*;
import java.io.*;
public class HelloWorld {
public static class Greeter {
private String name;
public Greeter(String name) {
this.name = name;
}
private String getName() {
return name;
}
private String greeting() {
return "Hello " + getName();
}
public void greet() {
System.out.println(greeting());
}
}
public static void main(String[] args) {
Greeter hw = new Greeter("world");
hw.greet();
}
} |
Python:
In file "greeter.py":
code: | class Greeter:
def __init__(self, name):
self.name = name
def __greeting(self):
return "Hello " + self.name
def greet(self):
print self.__greeting() |
In file "main.py":
code: | from greeter import *
hw = Greeter("world")
hw.greet() |
C#:
code: | using System;
public class HelloWorld {
public class Greeter {
private string _name;
private string name {
get {
return _name;
}
set { }
}
public Greeter(string name) {
this._name = name;
}
private string Greeting() {
return "Hello " + name;
}
public void Greet() {
Console.WriteLine(Greeting());
}
}
public static void Main(string[] args) {
Greeter hw = new Greeter("world");
hw.Greet();
}
} |
C++:
code: | #include <string>
#include <iostream>
class greeter
{
private:
std::string name;
std::string greeting()
{
return "Hello " + name;
}
public:
greeter(std::string init_name) : name (init_name) { }
void greet()
{
std::cout << greeting() << std::endl;
}
};
int main()
{
greeter * hw = new greeter("world");
hw->greet();
return 0;
} |
|
|
|
|
|
 |
Paul
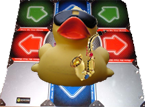
|
Posted: Thu Aug 19, 2004 9:32 pm Post subject: (No subject) |
|
|
Um... I think wtd's got them covered  |
|
|
|
|
 |
wtd
|
Posted: Thu Aug 19, 2004 9:50 pm Post subject: (No subject) |
|
|
Paul Bian wrote: Um... I think wtd's got them covered 
The scary thing is, I haven't even scratched the surface. Be afraid. Be very afraid.
Oh, and inheritance to provide a fancy greeting class where one can specify the greeting...
Ruby:
In file "flexible-greeter.rb":
code: | require "greeter"
class FlexibleGreeter < Greeter
def initialize(greeting_msg, name)
super(name)
@greeting_msg = greeting_msg
end
private
def greeting
"#{greeting_msg} #{name}"
end
attr_reader :greeting_msg, :name
end |
In file "main.rb":
code: | require "flexible-greeter"
hw = FlexibleGreeter.new("Hello", "world")
hw.greet |
O'Caml:
code: | class greeter name =
object(self)
method private greeting = "Hello " ^ name
method greet = print_endline self#greeting
end
class flexible_greeting greeting_msg name =
object(self)
inherit greeter as super
method private greeting = greeting_msg ^ " " ^ name
end
let hw = new flexible_greeter "Hello" "world" in hw#greet |
Python:
In file "greeter.py":
code: | class Greeter:
def __init__(self, name):
self.name = name
def __greeting(self):
return "Hello " + self.name
def greet(self):
print self.__greeting()
class FlexibleGreeter(Greeter):
def __init__(self, greeting_msg, name):
Greeter.__init__(self, name)
self.greeting_msg = greeting_msg
def __greeting(self):
return self.greeting_msg + " " + self.name |
In file "main.py":
code: | from greeter import FlexibleGreeter
hw = FlexibleGreeter("Hello", "world")
hw.greet() |
Eiffel:
In file "greeter.e":
code: | indexing
author: "Chris"
title: "Greeter"
purpose: "Greet someone"
class
GREETER
creation { ANY }
make
feature { NONE }
name: STRING
greeting: STRING is
do
Result := "Hello " + name
end
feature { ANY }
make(init_name: STRING) is
do
name := init_name
end
greet is
do
std_output.put_string(greeting)
std_output.put_new_line
end
end |
In file "flexible_greeter.e":
code: | indexing
author: "Chris"
title: "Greeter"
purpose: "Greet someone flexibly"
class
FLEXIBLE_GREETER
inherit
GREETER
rename
make as old_make
redefine
greeting
end
creation { ANY }
make
feature { NONE }
greeting_msg : STRING
greeting : STRING is
do
Result := greeting_msg + " " + name
end
feature { ANY }
make(init_greeting_msg, init_name : STRING) is
do
old_make(init_name)
greeting_msg := init_greeting_msg
end
end |
In file "hw.e":
code: | indexing
author: "Chris"
name: "Hello world!"
purpose: "Test GREETER and FLEXIBLE_GREETER classes."
class
HW
creation { ANY }
make
feature { ANY }
make is
local
hw1 : GREETER
hw2 : FLEXIBLE_GREETER
do
create hw1.make("world")
hw1.greet
create hw2.make("Hello", "world")
hw2.greet
end
end |
|
|
|
|
|
 |
templest
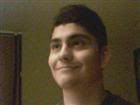
|
|
|
|
 |
wtd
|
Posted: Thu Aug 19, 2004 11:43 pm Post subject: (No subject) |
|
|
templest wrote: or you can just go here; http://www2.latech.edu/~acm/HelloWorld.shtml It's hello world in EVERY concievable language. 
I know a few languages that aren't on that list. Where're Ruby, Rexx, Rebol?
Besides, a lot of the examples there are very poor code.
This, though, is beautiful. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
templest
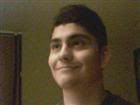
|
|
|
|
 |
the_short1
|
Posted: Fri Aug 20, 2004 1:11 pm Post subject: (No subject) |
|
|
hahah.. u all forgot one
the old school basic on tandy
5 cls 3 $$$ collors the screen blue i beleive..
10 print "hello world" % outputs hello world
%% those numbers are line numbers
then u can tye 'list' to see em all
and if u made a mistake u had to do hte whole lien again..
and thats hard if the lines really long.. it overwrites old line if u use same ien number twice.. and if u dont have spaces (i put every 5) if u needed to add a line in the middle there... if u dont have spaces... ur screwed ..
heheheh that was the first programmign language i ever knew..
i made some sick stuff with it.. forgot most of it now.. (i got it on paper somewhere tho)... i was able to do get ur name then put happy bday that person.. or whatever... plus osme nice graphic stuff....
tahts crzy WTD.,. or did u get a lot of those from that website u mentioned?
dam.. why cant they be semi easy like basic and turing? lot of them are so more complext..
sad... |
|
|
|
|
 |
wtd
|
Posted: Fri Aug 20, 2004 3:00 pm Post subject: (No subject) |
|
|
the_short1 wrote: tahts crzy WTD.,. or did u get a lot of those from that website u mentioned?
With the exception of the Shaespeare program, I wrote all of that code myself. |
|
|
|
|
 |
wtd
|
Posted: Sat Aug 28, 2004 2:29 pm Post subject: (No subject) |
|
|
A blast from the past!
Algol68:
code: | write ("Hello world!") |
Fortan77:
code: | program HelloWorld
write (*,*) 'Hello world!'
stop
end |
ABC:
code: | WRITE "Hello world!" |
|
|
|
|
|
 |
Tony
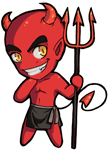
|
Posted: Sat Aug 28, 2004 2:43 pm Post subject: (No subject) |
|
|
the_short1 wrote: lot of them are so more complex
you think?
Quote:
Hello World in BrainF***, posted by Dmitri Katchalov <dmitrik@my-deja.com>
on comp.lang.misc on Feb. 14, 2000.
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
+++++++.+++++++++++++++++++++++++++++.+++++++..+++.--------------
-----------------------------------------------------.-----------
-.+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
++++++++++++++++++++++++.--------.+++.------.--------.-----------
--------------------------------------------------------.
 |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
wtd
|
Posted: Sat Aug 28, 2004 2:48 pm Post subject: (No subject) |
|
|
tony wrote: the_short1 wrote: lot of them are so more complex
you think?
Quote:
Hello World in BrainF***, posted by Dmitri Katchalov <dmitrik@my-deja.com>
on comp.lang.misc on Feb. 14, 2000.
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
+++++++.+++++++++++++++++++++++++++++.+++++++..+++.--------------
-----------------------------------------------------.-----------
-.+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
++++++++++++++++++++++++.--------.+++.------.--------.-----------
--------------------------------------------------------.

Real geeks code in Whitespace.  |
|
|
|
|
 |
|