Author |
Message |
qwerty
|
Posted: Fri May 28, 2004 5:15 pm Post subject: How to generate random numbers without repetition? |
|
|
I want to generate 22 random numbers between 1 and 52 and store them into an array, but each integer stored in the array must be different, i.e, there has to be repetition. If anyone know how to do it I will appretiate her/his help. Thanks.
Note: I am writing my program in Java. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
zylum

|
Posted: Fri May 28, 2004 5:49 pm Post subject: (No subject) |
|
|
you can take an array of the 52 numbers, scramble it and then choose the first 22 elements...
code: |
int[] numbers = new int[52];
int randNumbers = new int[22];
for (int i = 0; i < 52; i ++) numbers[i] = i;
for (int i = 0; i < 52; i ++) {
int idx = Math.random()*52 //i forgot how to do random in java so im using the action script way which is prolly the same way
int temp = numbers[idx];
numbers[idx] = numbers[i];
numbers[i] = temp;
}
for (int i = 0; i < 22; i ++) randNumbers[i] = numbers[i];
|
edit:
what my code does is basically the same as bugz's except that i dont know if java has a shuffle method so i made one manually... my code first fills an array 1..52 then it goes through each index and swaps values with another random index (this is the shuffle part) then i fill the randNumber array with the first 22 elements of the number array.. if you want you could fill it up with every other element but it doesnt really matter.. |
|
|
|
|
 |
wtd
|
Posted: Sat May 29, 2004 12:05 am Post subject: (No subject) |
|
|
Funny, I just write some code to do this in C++. It's a bit easier there, but it can be done in pretty much any language with some work.
code: | int[] possibles = new int[52];
for (int i = 0; i < 52; i++) possibles[i] = i + 1;
int[] alreadyChosen = new int[52];
for (int i = 0; i < 52; i++)
{
boolean hasInt = false;
int randIndex;
do
{
randIndex = Math.random() * 52;
for (int j = 0; j < i && !hasInt; j++)
{
if (possibles[randIndex] == alreadyChosen[j])
{
hasInt = true;
}
}
} while (hasInt);
alreadyChosen[i] = possibles[randIndex];
} |
Basically, start out with a full deck of cards. Choose one. If it's already in the destination deck, choose another one. If not, throw it on the destination deck and try again. Keep doing this until the destination deck has 52 cards. |
|
|
|
|
 |
Tony
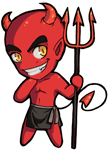
|
Posted: Sat May 29, 2004 11:36 am Post subject: (No subject) |
|
|
well zylum got the right idea... but I don't really understand his code at the moment.
The way I do it is to randomly pick a number from the array of remaining values rether then randomly guessing each time. link to code |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
bugzpodder

|
Posted: Sat May 29, 2004 11:58 am Post subject: (No subject) |
|
|
this is the way to do it in C++
code: |
#include<algorithm>
int main(){
int arr[52];
for (int i=0;i<52;i++) arr[i]=i;
random_shuffle(arr,arr+52);
}
|
or instead of random_shuffle,
code: |
for (int i=0;i<1000;i++)
swap(arr[rand()%52,rand()%52);
|
this may present a problem though, since rand() isnt really random as it uses seeds from the current time... so i would go with random_shuffle if i were you |
|
|
|
|
 |
qwerty
|
Posted: Sun May 30, 2004 4:57 pm Post subject: Thank You All!! |
|
|
Thank you all for helping me. I used the code which was posted by Zylum and it seems to be working perfectly. And now thanks to him I can finally finish my program.
Bye |
|
|
|
|
 |
speedyGonzales

|
Posted: Mon Nov 22, 2010 7:41 am Post subject: Re: How to generate random numbers without repetition? |
|
|
There is already a method that do that for you --> Collections.shuffled();
 |
|
|
|
|
 |
|