Multi-tasking
Author |
Message |
jono11
|
Posted: Fri May 21, 2004 10:11 am Post subject: Multi-tasking |
|
|
Hello. I am creating a program that is a rip off of Splinter Cell on a much smaller level (I have a previous post.) I am running into another problem. I need my AI to move independently no matter what the main character is doing. I want the AI to follow a predetermined path consistantely while the player stragetizes. However, I think its the loops but they are not allowing this to happen and in return nothing happens; the guard shows up on the screen and then the window header says running...but nothing happens. I was just wondering if anyone could help me because we are getting down to the crunch in our class and I just really could use the help. Please respond if you need a look at the program or for further clarification, or solution. Thanks for your time...
Jono |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
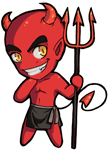
|
Posted: Fri May 21, 2004 4:24 pm Post subject: (No subject) |
|
|
in theory your loop structure should be something like
code: |
while (true)
{
processPlayerInput;
processAIstep;
}
|
both player and AI alternate taking turns. The key here is to take minor turns (such as move a step instead of run though the entire screen) to make it appear simultanious.
Also you've got to check for available player input, not wait for it as to not make the AI wait for you to make your move. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
da_foz

|
Posted: Fri May 21, 2004 7:28 pm Post subject: (No subject) |
|
|
Have you ever used threads? They are a bit advanced as I don't know what you have done up to now. I'm in third year university and we just covered them in operating systems last term.
Anyways, threads would be the best way to do that. You could have a thread for each player (one for the human and one for each AI).
Back the your thing not updating. I'm not great with graphics in Java, but is there an update something that you would need to use to update the screen?
The one the you have to be careful with tony's solution is that you would want to put a very short TIME limit on each player. I say TIME limit because what if the player does not more, then there is no input and the you might not get to the AI's turn. Could that be what is happening right now? |
|
|
|
|
 |
Tony
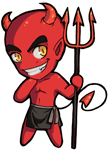
|
Posted: Fri May 21, 2004 8:02 pm Post subject: (No subject) |
|
|
da_foz wrote: then there is no input and the you might not get to the AI's turn.
have I not mentioned that already?
tony wrote: you've got to check for available player input, not wait for it as to not make the AI wait for you to make your move. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
da_foz

|
Posted: Sat May 22, 2004 12:06 am Post subject: (No subject) |
|
|
My bad, I was in a bit of a rush and did not read your whole post. |
|
|
|
|
 |
jono11
|
Posted: Wed May 26, 2004 12:08 pm Post subject: Ahhhhh!!!!! |
|
|
I tried that example earlier and I couldnt get it to work. It might be because I didnt check to see though. But that is the problem...I dont get what you mean by check. Is there a command for that? Do I need to make a method that performs that? What exactly do I need to do (and no, we have not learned about threads.) Thanks for the help and thanks in advance for the help on this topic...peace
Jono
(here is the program structure I have for the 3 classes...I have one more but it is strictly the different animations and doesnt really need to be shown. Its just used to draw everything. If you think you need it though let me know and you can have it.)
//The guard movement class
import java.awt.*;
import hsa.Console;
public class GuardMovement
{
public void delay (int howLong)
{
for (int i = 1 ; 1 <= howLong ; i++)
{
double garbage = Math.PI * Math.PI;
}
}
public void enemyMovement (Console c)
{
int a = 30;
int b = 20;
int width = 6;
int height = 18;
int x = 500;
int y = 50;
char input = ' ';
int i = 1000;
Animation character = new Animation (c, a, b, x, y, width, height);
while (true)
{
if (x >= 500 && y == 50)
{
x -= 40;
c.clear ();
character.drawGuardLeft (x, y, width, height);
}
else if (x == 100 && y < 400)
{
y -= 40;
character.drawGuardBackward (x, y, width, height);
}
else if (x == 100 && y >= 400)
{
x += 40;
character.drawGuardRight (x, y, width, height);
}
else if (x < 100 && y == 400)
{
y += 40;
character.drawGuardForward (x, y, width, height);
}
}
}
}
// The "Movement" class.
import java.awt.*;
import hsa.Console;
public class Movement
{
protected char input;
static Console c;
public void doIt (Console c)
{
charMovement (input);
}
public void charMovement (char input)
{
int a = 30;
int b = 20;
int width = 6;
int height = 18;
int x = 0;
int y = 0;
c = new Console ();
int i = 1000;
while (true)
{
input = c.getChar ();
Animation mainer = new Animation (c, a, b, x, y, width, height);
if (input == '8')
{
c.clear ();
b -= 40;
mainer.drawSmithForward (a, b, width, height);
}
else if (input == '4')
{
c.clear ();
a -= 40;
mainer.drawSmithLeft (a, b, width, height);
}
else if (input == '2')
{
c.clear ();
b += 40;
mainer.drawSmithBackward (a, b, width, height);
}
else if (input == '6')
{
c.clear ();
a += 40;
mainer.drawSmithRight (a, b, width, height);
}
else if (input == '7')
{
c.clear ();
a -= 40;
b -= 40;
mainer.drawSmithForward (a, b, width, height);
}
else if (input == '9')
{
c.clear ();
a += 40;
b -= 40;
mainer.drawSmithForward (a, b, width, height);
}
else if (input == '1')
{
c.clear ();
a -= 40;
b += 40;
mainer.drawSmithBackward (a, b, width, height);
}
else if (input == '3')
{
c.clear ();
a += 40;
b += 40;
mainer.drawSmithBackward (a, b, width, height);
}
else
{
mainer.drawSmithForward (a, b, width, height);
}
}
}
}
// The "Runner" class.
import java.awt.*;
import hsa.Console;
public class Runner
{
static Console c;
public static void main (String[] args)
{
char input = ' ';
Movement person = new Movement ();
GuardMovement enemy = new GuardMovement ();
while (true)
{
person.charMovement (input);
enemy.enemyMovement (c);
}
// Place your program here. 'c' is the output console
} // main method
} // Runner class |
|
|
|
|
 |
|
|