if statements in for loops
Author |
Message |
signal_1001
|
Posted: Fri Apr 30, 2004 8:15 am Post subject: if statements in for loops |
|
|
Im working on a program that converts roman numerals to arabic numbers. I cannot figure out how to say the roman numeral is invalid if they are not in the right order. this is some of my code code: | void toarabic()
{
int I=1, IV=4, V=5, IX=9, X=10, XL=40, L=50, XC=90, C=100, CD=400, D=500, CM=900, M=1000;
char num[13];
int arabic=0;
cout<<flush;
system("cls");
cout<<"\n\nPlease enter the roman numeral you wish to convert to a number. ";
cin.get(num,13);
cin.ignore(50, '\n');
for(int rom=strlen(num)-1; rom>=0; rom--)
{
if(num[rom]!='I'&& num[rom]!='V'&& num[rom]!='X'&& num[rom]!='L'&& num[rom]!='C'&& num[rom]!='D'&& num[rom]!='M')
{
cout<<"Invalid Roman Numeral, please enter another.";
pause();
return;
}
else if // 'i' cannot come after 'iv'; 'v' cannot come after 'x' etc
else
{
if(num[rom]=='I')
{
arabic+=1;
}
if(num[rom]=='V')
{
if(num[rom-1]=='I')
{
arabic+=4;
rom--;
}
else
arabic+=5;
} |
any ideas? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
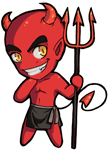
|
Posted: Fri Apr 30, 2004 10:19 am Post subject: (No subject) |
|
|
can't you just search for invalid substrings? ivi for example... |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
signal_1001
|
Posted: Fri Apr 30, 2004 4:48 pm Post subject: (No subject) |
|
|
I was thinking about it... but i was just wondering if there was any faster way. |
|
|
|
|
 |
Tony
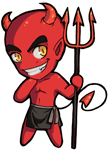
|
|
|
|
 |
wtd
|
Posted: Fri Apr 30, 2004 8:19 pm Post subject: (No subject) |
|
|
code: | #include <iostream>
#include <string>
#include <algorithm>
template <typename _t>
int index(_t * b, _t * e, _t v)
{
int c = 0;
for (; b != e; b++)
{
if (*b == v)
return c;
c++;
}
return c;
}
namespace std
{
void upcase(std::string::iterator b, std::string::iterator e)
{
for (;b != e; b++)
{
if (*b >= 97 && *b <= 122)
*b -= 32;
}
}
void downcase(std::string::iterator b, std::string::iterator e)
{
for (;b != e; b++)
{
if (*b >= 65 && *b <= 90)
*b += 32;
}
}
}
class RomanToArabicNumeralConverter
{
private:
std::string original;
int converted;
void upcase_original()
{
std::upcase(original.begin(), original.end());
}
public:
RomanToArabicNumeralConverter(const char * input)
: original(input), converted(0)
{
upcase_original();
}
std::string get_original() const
{
return original;
}
int get_converted() const
{
return converted;
}
bool valid_input_number() const
{
char roman_numerals[] = { 'I', 'V', 'X', 'L', 'C', 'D', 'M' };
int current_high_numeral_pos = 0;
for (int i = original.length() - 1; i >= 0; i--)
{
int current_char_pos = index(roman_numerals, roman_numerals + 7, original[i]);
if (current_char_pos >= 7)
return false;
else if (current_char_pos >= current_high_numeral_pos)
current_high_numeral_pos = current_char_pos;
else
return false;
}
return true;
}
void convert()
{
if (valid_input_number())
{
converted = 0;
for (int i = original.length() - 1; i >= 0; i--)
{
switch (original[i])
{
case 'I':
converted += 1;
break;
case 'V':
converted += 5;
break;
case 'X':
converted += 10;
break;
case 'L':
converted += 50;
break;
case 'C':
converted += 100;
break;
case 'D':
converted += 500;
break;
case 'M':
converted += 1000;
break;
}
}
}
}
};
int main()
{
RomanToArabicNumeralConverter converter("MVIII");
std::cout << converter.get_original() << std::endl;
converter.convert();
std::cout << converter.get_converted() << std::endl;
}
|
|
|
|
|
|
 |
signal_1001
|
Posted: Sat May 01, 2004 12:03 pm Post subject: (No subject) |
|
|
wow ...... so it seems..... thanks for the help though ...... any idea what im suppose to add or change when this warning appears...
fatal error C1010: unexpected end of file while looking for precompiled header directive |
|
|
|
|
 |
|
|