[Tutorial] Net Command
Author |
Message |
recneps

|
Posted: Mon Mar 29, 2004 4:23 pm Post subject: [Tutorial] Net Command |
|
|
Well i dont quite have the Net command mastered (at least for binary, maybe someone could add to this..) Anywho lets begin.
The net command is used to make a connection between 2 computers, which they can then send data via tcp/ip (normal internet protocol)
So lets look at the basic syntax for the major parts.
code: |
var ip:string
var net:int
net:=Net.WaitForConnection (port, ip)
|
This will tell Turing to wait for a connection on the port port and when it receives a connection, the variable ip will be filled with the IP address of the person connecting. When connected, the variable net will be a value higher than 0.
code: |
Net.OpenConnection (ip, port)
|
This will open a connection to another computer with the IP address ip and at the port port. The computer at the IP address must have already called Net.WaitForConnection in order for the connection to be a success. When the connection has been established, the variable net will be a value higher than 0.
When a connection has been established, a stream for sending data has been opened, much like the streams created with the open: and close: commands, which can be used with put, get, read, and write (read and write do not work for me, that is the problem with binary for me.)
So, to send data, simple use
where net is the net stream that was opened, and variable is either text in quotes (" ") or a variable.
To receive data, the computer must check if there is data to be received.
It does this using:
code: | if Net.LineAvailable (net) then
get: net, line
end if |
where net is the net connection, and line is a string variable.
If input is being sent character by character, then you can send it with
code: | var ch:char
put:net,char
|
and receive it using the Net.CharAvailable command.
code: | if Net.CharAvailable(net) then
get:net,char
|
Then, to close the connection, you must call
code: | Net.CloseConnection(net) |
where net is the variable of your net connection.
There is another way of using the Net module to access web pages rather than computers.
this is called by
code: | net:=Net.OpenURLConnection(url) |
where url is the address to a webpage in quotes, or a string variable containing the url. If a connection is successful, the stream variable, net will be a number greater than 0.
To get the webpage contents, use the commands put, get, read, and write, like you would when connecting to computer.
Note: This gets you the html code, does not display the webapge.
Here is a little program that demonstrates the URL connection.
code: |
setscreen("text")
var code:string
var net:int
net:=Net.OpenURLConnection("http://www.compsci.ca")
if net >0 then
loop
get:net,code:*
put code
exit when eof(net)
end loop
end if
|
Note: These methods all use the eof commands to tell when there is no more data, just like input from files. And the URL connection automatically closes at the eof of the stream.
Well thats about it i believe. Enjoy!
P.S. Kazix internet?? lol  |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
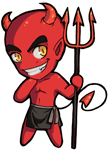
|
Posted: Mon Mar 29, 2004 4:44 pm Post subject: (No subject) |
|
|
It helps to understand if you think of net connection as reading from a file. In fact, as recneps pointed out - the syntax is quite similar, you just open the "file" differently.
And this net file does not have an eof, that is why you check if there's any info waiting to be read before reading it.
Also when reading a line, you use variable:*, same as when reading from a file
Overall its a nice tutorial, but there's quite a bit of consepts yet to be covered:? +50 Bits though |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
recneps

|
Posted: Mon Mar 29, 2004 7:24 pm Post subject: (No subject) |
|
|
the only concepts im missing are the binary stuff, which ive tried, it doesnr work :/ |
|
|
|
|
 |
Tony
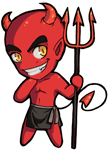
|
Posted: Mon Mar 29, 2004 9:17 pm Post subject: (No subject) |
|
|
well I mean all the server/client setups... multiperson chatroom... seting up procedures to listen to ports... that kind of stuff |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
SuperGenius
|
Posted: Tue Mar 30, 2004 9:03 pm Post subject: (No subject) |
|
|
I was working with the Net module a little, and i was able to establish a connection between two of my computers which happen are both wired(only one really) to my home network. Using the exact same program, I was not able to connect to someone else's computer. The address that the client types to connect is the result of the Net.LocalAddress function on the host computer. Is it possible for turing running on a firewalled coimputer to connect to another computer, or do I have to go and open up some ports or something? |
|
|
|
|
 |
recneps

|
Posted: Tue Mar 30, 2004 9:19 pm Post subject: (No subject) |
|
|
Oooooooooooooohhhhhhhhhh ok hehe.
Well for multiple connections, you would have to have it connect to different ports, then when it receives data, theres a loop in the server side, which will check if theres a connection on a port, and then send the data.
And for chatroom setup, there is a good example in the turing examples, except that the way they did it really sucks (char by char)
The best way to do it is have it
get text
put:net,text
and
if Net.LineAvailable(net) then
get:net,text
put text
and i believe there was a post on here about input / output at same time, and i believe the best answer was have processes, one to receive input, and one to get input.
There are also a couple commands i missed in the tutorial...
Net.CharAvailable(net)
returns true if there is a character available on the stream.
Net.TokenAvailable(net)
returns true if there is a token(single char surrounded by whitespace on either side) available.
Net.BytesAvailable(net)
returns the number of bytes available on the stream,(part of the binary which does not work for me.) |
|
|
|
|
 |
RobinK

|
Posted: Tue Mar 30, 2004 11:05 pm Post subject: (No subject) |
|
|
I keep getting WaitForConnection and OpenConnection is not in the export list of Net error. |
|
|
|
|
 |
Tony
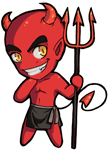
|
Posted: Tue Mar 30, 2004 11:38 pm Post subject: (No subject) |
|
|
you're more then likely using an old version of the compiler The most up to date as of right now is v4.0.5 |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
|
|