OOP
Author |
Message |
jonos

|
Posted: Sun Mar 21, 2004 12:00 am Post subject: OOP |
|
|
I've heard this quite often, and I've heared of OOT and about Java being object oriented and same with c++. can anyone clarify what this means? I've read some stuff on other pages, but it doesn't make a lot of sense. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Dan
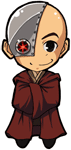
|
Posted: Sun Mar 21, 2004 12:05 am Post subject: (No subject) |
|
|
OOP = clases
OOP = object oriented progaming
java has to be OOP, c++ can be and c can not. turing has classes but the way they do it is a bit odd. |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
jonos

|
Posted: Sun Mar 21, 2004 12:08 am Post subject: (No subject) |
|
|
ahh, what are classes? |
|
|
|
|
 |
Dan
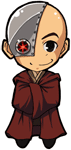
|
Posted: Sun Mar 21, 2004 12:15 am Post subject: (No subject) |
|
|
kind of hard to explaine off the top of my head and at 12 at nigth.
may be this page can help:
http://java.sun.com/docs/books/tutorial/java/concepts/ |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
wtd
|
Posted: Sun Mar 21, 2004 12:44 am Post subject: (No subject) |
|
|
Classes are a sort of blueprint for objects. They provide the two pieces of information about objects that are critical to object-oriented programming.
What does an object have.
What can an object do.
Object-oriented programming is more a way of thinking than a language extension. You can write object-oriented assembly code if you really want to.
It's a way of thinking where you think about the things you're manipulating in your program and organize the rest of the program around it. You then make these things into nice little packages with a consistent interface. Someone using the objects shouldn't have to know anything about how that little package does anything in order to use it.
Let's say you want a program that has people with names (pretty basic people) who can say hello to each other.
code: | #include <string>
#include <iostream>
class person
{
// These are things no one else
// can touch directly. They're in
// the "box".
private:
std::string name;
// Things the outside world can
// interact with.
public:
// A construct which accepts
// a string. The string initializes
// the name, but in the interface
// we don't care about the name
// of the parameter.
person(std::string);
// Since our name is hidden away
// we need an "accessor" to get
// to it, preventing direct access.
std::string get_name() const;
// We give ourperson the ability
// to say hello to another person.
void say_hello_to(const person&) const;
// You'll notice the use of "const".
// This can be used by any action
// that doesn't change the inner state
// of our object.
// Overload the << operator to make
// it easy to output the person's name.
friend std::ostream& operator<<(std::ostream&, const person&);
};
int main()
{
person bob("Bob"), john("John");
bob.say_hello_to(john);
john.say_hello_to(bob);
return 0;
}
// Now we can define "how"
// our class does what it does.
person::person(std::string init_name) : name(init_name) { }
std::string person::get_name() const
{
return name;
}
void person::say_hello_to(const person& p) const
{
std::cout << "Hi, " << p << "! My name is " << *this << std::endl;
}
std::ostream& operator<<(std::ostream& out, const person& p)
{
return out << p.name;
}
|
|
|
|
|
|
 |
PaddyLong
|
Posted: Sun Mar 21, 2004 1:12 am Post subject: (No subject) |
|
|
OOP is a pretty different way of programming than the structural form that you're probably used to... I'll try to give you a bit of an idea of the ideas behind OOP
INTRODUCTION
what is an object?
in OOP you've got classes which are like templates for Objects....
an Object is a variable with the type of your class (say you had a class Foo, you could then have object Bar by defining it as type Foo)
what makes up a class/object?
an object has its own set of data (set of variables) available to it, you can either choose to make these available to the rest of the program (public) or have them only available to the object's methods (private). this is the concept of encapsulation or information hiding...
objects also have methods (another word for functions) which it can perform. like the data, the methods can also be made available to the rest of the program or only available to the rest of the methods.
inheritance/polymorphism
in OOP it is also possible to have a class be like a sub-class of another... when you do this, the sub-class inherits all of the parent class's data and methods. but, the sub-class also has some of its own unique data and method.
EXAMPLE
here's a psuedo example to try to help clarify some of this
let's say you have a person object...
what attributes might a person have? (what is its data)
name, age, sex, appearance
now what can a person do? (what are its methods)
walk, talk, jump, run
now, consider a sub-class of person... let's say a programmer for example
remember the programmer gets all the attributes and methods that person had so, it has a name, age, sex and appearance and can walk, talk, jump and run
what additional attributes might a programmer have?
languagesKnown
(hmm probably a bad example lol)
what can a programmer do that other persons can't
writeProgram, learnLanguage, forgetLanguage
now let's consider how you would use this in a program...
you would define your class person and define the data types and the methods
then you would make your object by declaring a variable to be of type person (well only declaring it doesn't actually make it, you have to tell the program to actually make the object - ie allocate the memory for it)
then you can make calls on the person's methods...
like
person.walk(store)
person.talk(hello)
things like this... or if your data is encapulated (as it normally should be), use get/set methods (also known as inspector/modifier) to set the variables
Happy birthday person.getName!
person.setAge(person.getAge+1)
CONCLUSION
so what are the advantages to OOP over structural programming?
well, it makes programs a LOT easier to write and understand...
consider having a bunch of variables that are all decribing something in your program and each one having to have a unique name (you could use arrays or structs, but structs are almost OOP any way)...
and then having to have functions in the program to modify these variables in different ways ...
all of this could get confusing and cluttered...
so with OOP, your class would be defined most likely in another file or just once in your program. then your objects are also declared once, like a variables, but instead of having to define 4 arrays, one each for name, age, sex, appearance, you can declare ONE array of type person to have an array of person objects.
then instead of having a function control the attributes of the person (ie give the function the index of the arrays and modify that index in all your arrays) you call the person object's method and the method changes the person's attributes as required
so, hopefully this helped give you some understanding of the what/why of OOP. it can be a kind of tricky concept at first, but once you get it, you'll see why it's gaining favourability. |
|
|
|
|
 |
PaddyLong
|
Posted: Sun Mar 21, 2004 1:14 am Post subject: (No subject) |
|
|
wtd wrote: Object-oriented programming is more a way of thinking than a language extension. You can write object-oriented assembly code if you really want to.
nah, you couldn't... because assembly is all direct memory and register access and manipulation.
the language needs to provide the tools for it, because it is more than just a way of thinking. it includes some concepts that you can't do without the propper language constructs. |
|
|
|
|
 |
wtd
|
Posted: Sun Mar 21, 2004 1:16 am Post subject: (No subject) |
|
|
And the above in a few other languages, in case that can help. I know sometimes it helps me see the common things that aren't just language syntax.
code: | // in Objective-C
#import <objc/Object.h>
#import <string.h>
#import <stdio.h>
@interface Person : Object
{
@private
char * name;
}
- initWithName: (const char *) initName;
- (char *) getName;
- (void) sayHelloTo: (Person *) p;
@end
int main()
{
Person * bob = [[Person alloc] initWithName: "Bob" ];
Person * john = [[Person alloc] initWithName: "John"];
[bob sayHelloTo: john];
[john sayHelloTo: bob];
return 0;
}
@implementation Person
- initWithName: (const char *) initName
{
[super init];
name = (char *)malloc(sizeof(char *));
strcpy(name, initName);
return self;
}
- (char *) getName
{
return name;
}
- (void) sayHelloTo: (Person *) p
{
printf("Hi, %s! My name is %s\n", [p getName], [self getName]);
}
@end
// in Java
import java.lang.*;
import java.io.*;
public class MyProgram {
private class Person {
private String name;
public Person(String initName) {
name = initName;
}
public String getName() {
return name;
}
public void sayHelloTo(Person p) {
System.out.println("Hi, " + p.getName() + "! My name is " + getName());
}
}
public static void main(String[] args) {
Person bob = new Person("Bob");
Person john = new Person("John");
bob.sayHelloTo(john);
john.sayHelloTo(bob);
}
}
# in Python
class Person:
def __init__(self, initName):
self.__name = initName
def getName(self):
return self.__name
def sayHelloTo(self, p):
print "Hi, " + p.getName() + "! My name is " + self.getName()
bob = Person("Bob")
john = Person("John")
bob.sayHelloTo(john)
john.sayHelloTo(bob)
# in Ruby
class Person
private
attr_writer :name
public
attr_reader :name
def initialize(name)
@name = name
end
def say_hello_to(p)
puts "Hi, " + p.name + "! My name is " + name
end
end
bob = Person.new("Bob")
john = Person.new("John")
bob.say_hello_to(john)
john.say_hello_to(bob)
-- in Eiffel
class PERSON
creation with_name
feature { NONE } name : STRING
feature { ANY }
with_name(init_name : STRING) is
do
name := init_name
end
get_name : STRING is
do
Result := name
end
say_hello_to(p : PERSON) is
do
std_output.put_string("Hi, " + p.get_name + "! My name is " + name)
std_output.put_new_line
end
end
class TEST
creation make
feature { ANY }
make is
local
bob, john : PERSON
do
create bob.with_name("Bob")
create john.with_name("John")
bob.say_hello_to(john)
john.say_hello_to(bob)
end
end
# in Perl 5
package Person;
sub new {
my $class = shift;
my $name = shift || "";
my $self = {"name" => $name};
bless $self, $class
}
sub name
{
my $self = shift;
return $self->{"name"}
}
sub say_hello_to {
my $self = shift;
my $p = shift;
print "Hi, ${$p->name}! My name is ${$self->name}\n"
}
package main;
my $bob = Person->new("Bob");
my $john = Person->new("John");
$bob->say_hello_to($john);
$john->say_hello_to($bob); |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Sun Mar 21, 2004 1:19 am Post subject: (No subject) |
|
|
PaddyLong wrote: wtd wrote: Object-oriented programming is more a way of thinking than a language extension. You can write object-oriented assembly code if you really want to.
nah, you couldn't... because assembly is all direct memory and register access and manipulation.
the language needs to provide the tools for it, because it is more than just a way of thinking. it includes some concepts that you can't do without the propper language constructs.
Well, you can do it, you just can't necessarily enforce it. My final for my assembly course in college was all object-oriented (using MASM 6.15).  |
|
|
|
|
 |
|
|