Java and Graphics... update screen...
Author |
Message |
Homer_simpson

|
Posted: Tue Feb 17, 2004 5:45 pm Post subject: Java and Graphics... update screen... |
|
|
let's say i gots this:
code: | import java.io.*;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.math.*;
class gui
{
public static void main (String args [])
{
JFrame frame = new JFrame ("Someshit");
frame.addWindowListener (new WindowAdapter ()
{
public void windowClosing (WindowEvent e)
{
System.exit (0);
}
}
);
frame.setSize (600, 400);
JPanel pane = (JPanel) frame.getContentPane ();
pane.add (new SysDraw ());
frame.setVisible (true);
}
}
class SysDraw extends JComponent
{
public SysDraw ()
{
repaint ();
}
public void paint (Graphics g)
{
for (int i=0;i<600;i++)
{
g.drawLine(0,0,i,400);
}
}
}
|
got my gui window setup and everything but when i draw stuffs on the page... in this case a line...it doesn't update the screen so i can delete that and draw again... now i'm wondering if there is a command like View.Update in turing for java? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Homer_simpson

|
Posted: Wed Feb 18, 2004 10:52 am Post subject: (No subject) |
|
|
basically all i need is an equivalent for View.Update for java!  |
|
|
|
|
 |
Dan
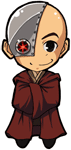
|
Posted: Wed Feb 18, 2004 11:29 am Post subject: (No subject) |
|
|
i know there is one in the java 2d api, i will check when i get home on how to use it.... |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
Homer_simpson

|
Posted: Wed Feb 18, 2004 5:33 pm Post subject: (No subject) |
|
|
awesome! |
|
|
|
|
 |
rizzix
|
Posted: Fri Feb 20, 2004 5:55 pm Post subject: (No subject) |
|
|
extending my previous example.. (once again)
Java: | import javax.swing.*;
import java.awt.*;
class DrawView extends Canvas {
private Image bImage; // offscreen buffer
private Graphics bg; // offscreen graphics context
private Graphics2D g2d; // onscreen graphics context
private int w, h; // width and height of canvas (Component)
DrawView (int width, int height ) {
super ();
setBackground (Color. black);
w = width;
h = height;
}
public void paint (Graphics g ) {
g2d = (Graphics2D) g; // cast to Graphics2D (just in case)
bImage = createImage (w, h ); // create a new buffer image
bg = bImage. getGraphics(); // get Graphics context for image
bg. setXORMode(Color. white); // set white as the XORed colour
for (int i = 0; i < 600; i++ )
{
bg. drawLine(0, 0,i, 400); // drawn in white (on buffer)
updateScreen ();
bg. drawLine(0, 0,i, 400); // drawn in background color (XORed)
}
bg. dispose(); // dispose graphics context
bImage. flush(); // flush (empty) image
}
private void updateScreen () {
g2d. drawImage(bImage, 0, 0, this); // load buffer to screen
}
}
|
|
|
|
|
|
 |
Homer_simpson

|
Posted: Sun Feb 22, 2004 7:19 pm Post subject: (No subject) |
|
|
ummm... dude... how do i run a program that doesn't have a main method :S |
|
|
|
|
 |
rizzix
|
Posted: Mon Feb 23, 2004 3:45 pm Post subject: (No subject) |
|
|
Java: | import javax.swing.*;
import java.awt.Dimension;
public class Test {
public static void main (String[] args ) {
// initialised with the title for the title bar
JFrame frame = new JFrame("Test Application");
Dimension screenSize = frame. getToolkit(). getScreenSize();
frame. setBounds(screenSize. width/ 4, screenSize. height/ 4,
screenSize. width/ 2, screenSize. height/ 2);
frame. setDefaultCloseOperation(JFrame. EXIT_ON_CLOSE);
int w = frame. getBounds(). width;
int h = frame. getBounds(). height;
frame. getContentPane(). add(new DrawView (w, h ));
frame. show();
}
} |
|
|
|
|
|
 |
Homer_simpson

|
Posted: Tue Feb 24, 2004 6:01 pm Post subject: (No subject) |
|
|
makes me feel such a noobie at this...  |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
rizzix
|
Posted: Tue Feb 24, 2004 11:25 pm Post subject: (No subject) |
|
|
yea, it might take awhile to get used to. u basically need to think Objects heh.
anyways..
the DrawView class i wrote above is basically this:
i create an image (its better if you use a BufferedImage.. but Image will do)
now i do all my drawing onto this image. when i need to update the screen, i just load the image on to the screen.
to make this process simpler i wrote this: http://www.compsci.ca/v2/viewtopic.php?t=3817
try it out.. i've wraped a turing like syntax over the java methods.. so it should be easier to concentrate on drawing than the actual process of buffering etc..
here's an example using that class:
Java: | import java.awt.*;
import javax.swing.JFrame;
class DrawView extends BufferedCanvas {
DrawView (JFrame parent ) {
super (parent );
}
protected void offscreenPaint () {
for (int i = 0; i < maxx (); i++ )
{
drawline (0, 0 ,i, 400, Color. white);
drawfilloval (0, 0, i, i, Color. red);
update ();
drawline (0, 0, i, 400);
drawfilloval (0, 0, i, i );
}
}
} |
and here's the application class:
Java: | import javax.swing.*;
import java.awt.Dimension;
import java.awt.Color;
public final class Test {
public static void main (String[] args ) {
JFrame frame = new JFrame("Test Application");
Dimension screenSize = frame. getToolkit(). getScreenSize();
frame. setBounds(screenSize. width/ 4, screenSize. height/ 4,
screenSize. width/ 2, screenSize. height/ 2);
frame. getContentPane(). add(new DrawView (frame ));
frame. setDefaultCloseOperation(JFrame. EXIT_ON_CLOSE);
frame. show();
}
}
|
|
|
|
|
|
 |
|
|