[Tutorial] Rotation and Movement
Author |
Message |
AsianSensation
|
Posted: Sat Feb 07, 2004 11:05 am Post subject: [Tutorial] Rotation and Movement |
|
|
This is a tutorial on teaching how to make a image move toward the direction they are facing.
code: | View.Set ("offscreenonly")
var pic := Pic.FileNew ("spaceship.bmp")
var picRotated : array 0 .. 35 of int
for rep : 0 .. 35
picRotated (rep) := Pic.Rotate (pic, rep * 10, Pic.Width (pic) div 2 + 7, Pic.Height (pic) div 2 + 5)
end for
var chars : array char of boolean
var tmpShip := picRotated (0)
var counter := 0
var x, y : real := 0
loop
Input.KeyDown (chars)
if chars (KEY_RIGHT_ARROW) then
counter -= 1
tmpShip := picRotated (counter mod 36)
end if
if chars (KEY_LEFT_ARROW) then
counter += 1
tmpShip := picRotated (counter mod 36)
end if
if chars (KEY_UP_ARROW) then
x += cosd ((counter mod 36) * 10 + 90) * 5
y += sind ((counter mod 36) * 10 + 90) * 5
end if
Pic.Draw (tmpShip, round (x), round (y), picMerge)
View.Update
cls
delay (50)
end loop |
This is the entire code, the picture is uploaded on the bottom. Now I will go into a indepth explaination of the above code.
First, find a picture of the right size that you would wish to rotate, like Pac-Man or something. now code: | var picRotated : array 0 .. 35 of int
for rep : 0 .. 35
picRotated (rep) := Pic.Rotate (pic, rep * 10, Pic.Width (pic) div 2 + 7, Pic.Height (pic) div 2 + 5)
end for | This is where we generate the 36 pictures of the original picture, all at different angle. The reason I used 36 is because it's 1/10 of 360, which is 1 full rotation, and generating 360 pictures just isn't efficient.
Pic.Rotate let you create a new picture at a certain angle, from an original picture, rotating about a certain point. The tricky part here is you need to find the point of rotation. To find it, draw a circle around the entire picture, and find the center of the circle. That would be your point. Notice that if your center of rotation is off, some part of the picture could be cut off. So play around with either the picture or the center until you find a suitable one (Notice how this would be a much easier job if your object to rotate was circular, or near circular.)
Next, I declared a variable "counter", which would keep track of what angle my rotation is. code: | if chars (KEY_RIGHT_ARROW) then
counter -= 1
tmpShip := picRotated (counter mod 36)
end if | Here is where I make use of it. Whenever the right arrow is pressed, I subtract 1 from counter, and get the remainder when I divide counter by 36 (which would give me a number between 0 - 35, which, coincidentally, is the range of the subscripts of the array that holds the rotated picture.) Same thing applies if the left arrow is pressed, but instead of subtracting 1, we add 1 to counter.
code: | if chars (KEY_UP_ARROW) then
x += cosd ((counter mod 36) * 10 + 90) * 5
y += sind ((counter mod 36) * 10 + 90) * 5
end if | Here, if the up arrow is pressed, then we move the ship toward the direction it's facing. How do we know where it's facing? Well, remember how I used the remainder to get a number between 0 - 35? that corresponds to the picture of the ship facing a certain angle. 0 would corresponds 0 degree, 5 would corresponds to 50 degree. so "(counter mod 36) * 10" is the angle of the ship. I added 90 to the angle because my ship started out facing upward, which means it has already rotated 90 degrees counterclockwise. Now, to get the relationship between the angle, and the x and y distance that the ship moves, we use trig. If you have a basic understanding of what trigonometry is , then you would know what I did. Basicly, cosd (theta) is the horizontal component of a vector with angle theta, while sind (theta) is the vertical component of a vector with angle theta. I then multiply the sind or cosd of the angle by 5, which would be the magnitude of the vector.
lastly, I draw the picture of that certain angle, View.Update and whatnot, and we are done.
since compsci doesn't let me upload bmps, and I have no other image editing tool as of right now, I'll just link the image. http://members.lycos.co.uk/asiansensation8733/hpbimg/spaceship.bmp |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Cervantes

|
Posted: Sat Feb 07, 2004 11:25 am Post subject: (No subject) |
|
|
really good tutorial. with that it wouldn't be hard to make your own version of the SpaceGame that comes with Turing. |
|
|
|
|
 |
TheXploder

|
Posted: Sat Feb 07, 2004 11:25 am Post subject: (No subject) |
|
|
Thanx a lot AsianSensation I was trying to get that for weeks.
Great Tutorial.
Is creating 36 pictures efficient? |
|
|
|
|
 |
AsianSensation
|
Posted: Sat Feb 07, 2004 11:48 am Post subject: (No subject) |
|
|
yeah, 36 images is usually efficient. But if you want more precise rotation, you have to have more pictures. This is where loading bars comes into play. |
|
|
|
|
 |
Paul
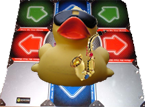
|
Posted: Sat Feb 07, 2004 11:59 am Post subject: (No subject) |
|
|
Thanx alot! I'd give you bits, but you already have 1000 max. If I were to make a game using this kind of code and a bmp, would collision detection with coordinates be better than with whatdotcolor? |
|
|
|
|
 |
TheXploder

|
Posted: Sat Feb 07, 2004 12:04 pm Post subject: (No subject) |
|
|
but you could have only one image saved in memory at a time, and free the other images... |
|
|
|
|
 |
recneps

|
Posted: Sat Feb 07, 2004 12:09 pm Post subject: (No subject) |
|
|
no, the imagesd are stored in arrays, and the image is changed when the angle changes, see? |
|
|
|
|
 |
AsianSensation
|
Posted: Sat Feb 07, 2004 12:10 pm Post subject: (No subject) |
|
|
Paul Bian wrote: Thanx alot! I'd give you bits, but you already have 1000 max. If I were to make a game using this kind of code and a bmp, would collision detection with coordinates be better than with whatdotcolor?
whatdotcolor is evil! Evil I tell you! Seriously though, in a fast action paced game, whatdotcolor would not be as precise as using math and equations of coordinates to detect collision.
TheXploder wrote: but you could have only one image saved in memory at a time, and free the other images...
I am pretty sure calling a picture stored in memory is alot quicker than generating a picture and then freeing it and then generating another picture. That's why at the beginning of your game, make a intro, and generate all your pictures in the background. (and don't use processes to do it.) |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Boiq
|
Posted: Mon Sep 08, 2008 11:01 am Post subject: RE:[Tutorial] Rotation and Movement |
|
|
Great tutorial.
This guide helped me a great amount, thanks! |
|
|
|
|
 |
|
|