Author |
Message |
Tony
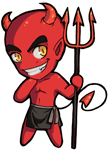
|
Posted: Sat Sep 21, 2002 1:18 pm Post subject: (No subject) |
|
|
array is a group of similar variables, combined for organization and easier access to this particular group of variables.
code: | Dim MyArray (9) as String |
will declear 10 string varaibles called MyArray(0), MyArray(1) ... MyArray(9)
You can also specify the range of the array:
code: | Dim MyArray(1 to 10) as String |
This will result in declaration of variables MyArray(1) ... MyArray(10)
Now you can use each variable just by using the name and desired position of the array. Such as MyArray(1) will use first variable in the group and MyArray(10) will use 10th.
The best and mose useful part of the array is that you can pick a variable based on yet another variable.
will use a variable MyArray with position in the array determined by variable index. So if index is 1, then MyArray(1) would be called. If index is 10, then MyArray(10) will be used.
This is useful in cases where the user or the program picks what variable to use. So instead of writing up 100s of IF statments, an array would be a better choice. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
jonos

|
Posted: Wed Jan 28, 2004 4:22 pm Post subject: (No subject) |
|
|
How would I initialize an array (like init in Turing), instead of having to go
MyArray(1) = "blah"
MyArray(2) = "blahblah"
MyArray(3) = "blahblahblah"
thankyou |
|
|
|
|
 |
Tony
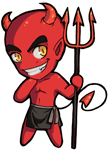
|
Posted: Wed Jan 28, 2004 4:25 pm Post subject: (No subject) |
|
|
well tuirng's init doesn't do much... you still have to write out all the number
what I can sujest is to load the values from a file though  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
jonos

|
Posted: Wed Jan 28, 2004 4:33 pm Post subject: (No subject) |
|
|
wow, fast reply... ill just do it the long way, dosn't matter... thanks a lot. |
|
|
|
|
 |
Acid

|
Posted: Wed Jan 28, 2004 5:14 pm Post subject: (No subject) |
|
|
I guess if you wanted each to have one more "blah" than the last you could make a loop do that
code: | Dim X as Integer
MyArray(1) = "Blah"
X = 2
For X = 2 to 10
MyArray(X) = MyArray(X - 1) & "blah"
next X
|
|
|
|
|
|
 |
jonos

|
Posted: Wed Jan 28, 2004 5:43 pm Post subject: (No subject) |
|
|
sorry, i meant the extra blahs to show a difference between the different elements of the array. i should have put different names for each array element. |
|
|
|
|
 |
McKenzie

|
Posted: Wed Jan 28, 2004 6:29 pm Post subject: (No subject) |
|
|
May sound cheap but often times in VB it is actually easier to place a listbox on your form and set visible to false. You can load the listbox at design time with the List property, you can set it to be automatically sorted. You can add and remove elements without worrying about using redim. |
|
|
|
|
 |
Tony
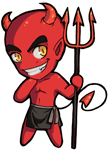
|
Posted: Wed Jan 28, 2004 8:29 pm Post subject: (No subject) |
|
|
actually it's a very creative idea i probably wouldn't have thought of it like that  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
Acid

|
Posted: Mon Feb 02, 2004 1:09 pm Post subject: (No subject) |
|
|
When you create a control array how do you set up a Select Case Index? |
|
|
|
|
 |
Tony
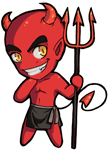
|
Posted: Mon Feb 02, 2004 2:35 pm Post subject: (No subject) |
|
|
not sure what you're asking, but in a control array all events will have
as their parameter. Such as
code: |
sub Buttons_Click(index as integer)
Buttons(index).Caption = "you clicked me"
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Acid

|
Posted: Mon Feb 02, 2004 4:37 pm Post subject: (No subject) |
|
|
Thanks, I figured it out. Sorry for the confusing description of what I needed explained, that was the best I could describe it from what I'd been told I needed to do |
|
|
|
|
 |
robert396
|
Posted: Tue May 20, 2008 8:54 am Post subject: RE:[tutorial] arrays |
|
|
How do i link a card array to card images from a folder for a Black jack Game |
|
|
|
|
 |
unoho

|
Posted: Mon Sep 29, 2008 7:46 pm Post subject: RE:[tutorial] arrays |
|
|
hi, does anyone know how i can arrange this code??
dim intCount as integer, intLotto(1 to 6) as integer
For intCount = 1 To 6
Randomize
intLotto(intCount) = Int(Rnd() * 49) + 1
picOutput.Print intLotto(intCount);
Next intCount
what i wanna do i like sort the output for example, 5 17 25 28 41 47 etc.
also, i don't wanna repeat any number.
you don't have to tell me the code but please some clue would be really appriciated.
thanks soo much |
|
|
|
|
 |
|