Getters and Setters, why?
Author |
Message |
Nathan4102
|
Posted: Mon Jun 23, 2014 4:35 pm Post subject: Getters and Setters, why? |
|
|
I don't understand the reasoning and necessity of getters and setters in Java. Here is an example I found online:
Java: | private String myField;
public String getMyField()
{
return this.myField;
}
public void setMyField(String value)
{
this.myField = value;
} |
Now what's the advantages of doing the above, OR setting myField to public and accessing it directly? They both seem to work in the exact same way, I don't understand why getters and setters are necessary. I'm sure I'm missing something though :p
Thanks! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Insectoid

|
Posted: Mon Jun 23, 2014 5:10 pm Post subject: RE:Getters and Setters, why? |
|
|
It hides the implementation. Maybe the setters do more than just set the field. Consider a class Circle, with fields x, y, radius, diameter, area, circumference, etc. Getters and setters for x and y might do nothing special, but the setter for radius might also reset the diameter, area, and circumference.
code: |
public void setRadius (int i){
radius = i
diameter = 2*i
circumference = 2*Pi*i
area = Pi*i**2
} |
If someone were to manually change the radius, the other fields would never be updated and the object would become corrupt. |
|
|
|
|
 |
Tony
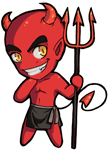
|
Posted: Mon Jun 23, 2014 5:29 pm Post subject: RE:Getters and Setters, why? |
|
|
on the getter side, you might want to perform other actions such as logging, caching, or changing implementation.
code: |
public int getCreditScore(Customer user) {
log("credit score request for " + user);
score = cache.get("creditscore", user) || calculateCreditScore(user); // maybe change calculateCreditScore() in the future
return score;
}
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Nathan4102
|
Posted: Mon Jun 23, 2014 6:37 pm Post subject: RE:Getters and Setters, why? |
|
|
Ahh ok, I've only ever seen getters and setters with just the one line changing the variable, that makes sense. And Insectoid, what do you mean "hides the implementation"? |
|
|
|
|
 |
Insectoid

|
Posted: Mon Jun 23, 2014 7:18 pm Post subject: RE:Getters and Setters, why? |
|
|
When you use an external library that isn't open-source (a game engine, for example), you aren't provided with source code. The owners of that code might not want you to know how it works. Letting you access variables directly can give some insight into how they've done things, so they just give you a bunch of functions (including getters & setters) instead. |
|
|
|
|
 |
|
|