Highscores List
Author |
Message |
TFish

|
Posted: Sat Feb 18, 2012 9:13 pm Post subject: Highscores List |
|
|
This is a highscore procedure that I've used in a few games I've made, but I thought I'd post it here in case someone doesn't know how to do it. Here is the entire code:
Turing: | const numScores : int := 5 % number of saved highscores
var names, hNames, scores, hScores : array 1 .. numScores of string % stores the highscore names/scores, names/scores from the text file
var highScorestxt : int % variable where the txt is stored
var space : string % variable for the space
open : highScorestxt, "highscores.txt", get % open the highscores text file
for i : 1 .. numScores % 1 to 5 for loop for 5 names
exit when eof (highScorestxt ) % exit the loop when turing reaches the end of the file
get : highScorestxt, hNames (i ) % get the name
get : highScorestxt, hScores (i ) % get the score
get : highScorestxt, space : * % get the space between highscores
names (i ) := hNames (i ) % store the name in another variable
scores (i ) := hScores (i ) % store the score in another variable
end for
close : highScorestxt % close the file
for decreasing i : numScores .. 1 % starting from the bottom score
if user.score > strint (scores (i )) then % if the current score is higher than the one you're checking
for decreasing j : numScores .. i % starting from the bottom score
if j not= numScores then
hNames (j + 1) := names (j ) % move the name down
hScores (j + 1) := scores (j ) % move the score down
end if
end for
hNames (i ) := user.name % put the current user's name in
hScores (i ) := intstr (user.score ) % put the current user's score in
end if
end for
open : highScorestxt, "highscores.txt", put % open the highscores text file
for i : 1 .. numScores
put : highScorestxt, hNames (i ) % write the name in the txt
put : highScorestxt, hScores (i ) % write the score in the txt
put : highScorestxt, space % write the space in the txt
end for
close : highScorestxt % close the txt file
|
This opens up the highscores.txt file (where the scores are kept), and it saves every line into a variable. The first line is the name, and is saved into hNames (i), the second line is the number, and is saved into hScores (i) and the space is saved into the space variable. This is repeated for however many scores there are. At the end, it closes the file
Turing: | open : highScorestxt, "highscores.txt", get % open the highscores text file
for i : 1 .. numScores % 1 to 5 for loop for 5 names
exit when eof (highScorestxt ) % exit the loop when turing reaches the end of the file
get : highScorestxt, hNames (i ) % get the name
get : highScorestxt, hScores (i ) % get the score
get : highScorestxt, space : * % get the space between highscores
names (i ) := hNames (i ) % store the name in another variable
scores (i ) := hScores (i ) % store the score in another variable
end for
close : highScorestxt % close the file
|
This part checks where the current score (the one the player just got), and finds out where it fits in the list (if at all). You want to start from the bottom, because your list should already be highest at the top and lowest at the bottom. If the current score is higher than the one saved, then you have to move all of the scores down, and save the current score in the spot where it fits (e.g., if the current score is higher than the 4th, but lower than the 3rd, the current score will be saved in the 4th position, the 4th place score will be saved in the 5th position and the 5th place score will be knocked off the leaderboards).
Turing: | for decreasing i : numScores .. 1 % starting from the bottom score
if user.score > strint (scores (i )) then % if the current score is higher than the one you're checking
for decreasing j : numScores .. i % starting from the bottom score
if j not= numScores then
hNames (j + 1) := names (j ) % move the name down
hScores (j + 1) := scores (j ) % move the score down
end if
end for
hNames (i ) := user.name % put the current user's name in
hScores (i ) := intstr (user.score ) % put the current user's score in
end if
end for |
This will now save the revised score in the highscores.txt file.
Turing: | open : highScorestxt, "highscores.txt", put % open the highscores text file
for i : 1 .. numScores
put : highScorestxt, hNames (i) % write the name in the txt
put : highScorestxt, hScores (i) % write the score in the txt
put : highScorestxt, space % write the space in the txt
end for
close : highScorestxt % close the txt file
|
This is the format the highscores.txt file has to be in. Remember to have an extra space at the end of the file.
code: | none
0
none
0
none
0
none
0
none
0
|
If you have any improvements, feel free to post them. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
TW iz Rippin
|
Posted: Thu Feb 23, 2012 11:57 am Post subject: RE:Highscores List |
|
|
+bits ty |
|
|
|
|
 |
Flying Carpet
|
Posted: Wed Jun 13, 2012 9:02 pm Post subject: RE:Highscores List |
|
|
Well it is obvious that the User var that you use has to bewhatever your "user" var is, but, when I create a new var "user" or whatever I want and change the code accordingly I get an error.
Turing tells me "user cannot be followed by a "."
Any help? |
|
|
|
|
 |
Tony
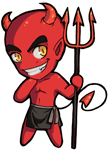
|
Posted: Wed Jun 13, 2012 9:52 pm Post subject: RE:Highscores List |
|
|
what's the type of the user variable that you created? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Flying Carpet
|
Posted: Thu Jun 14, 2012 5:58 pm Post subject: RE:Highscores List |
|
|
Well its a char1 variable, implying to player 1, which has an assigned sprite.
This is a game for a Grade 10 Final project, with a partner, still a work in progress.
What I don't get is why use such a long code. I saw a tut that has maybe 5-10 lines for highscores.
Another problem is we cant figure out for the hell of us how to pause the game, even our teacher doesn't know lol. The game itself is a procedure.
While using the pause command, you set time "UNITS" Even if this is set to 1, the pause goes on forever. Why? Also the Input.Pause wont work either.
What we want is an interactive pause screen to pop up once a certain key is pressed, and to unpause when the SAME
key is pressed. |
|
|
|
|
 |
Tony
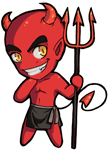
|
Posted: Thu Jun 14, 2012 7:07 pm Post subject: RE:Highscores List |
|
|
Turing is right. Char1 type does not contain any properties/methods, so using the .-operator is meaningless.
It would be best for you to simply implement your own code -- easier to understand too. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Flying Carpet
|
Posted: Thu Jun 14, 2012 7:13 pm Post subject: RE:Highscores List |
|
|
About the pause why is that?
I tried the Input.Keydown, then an Input.Pause. If you press P it pauses, and waits for a keystroke, and will unpause when any key is pressed, how can I make it so it only unpauses after the P key is pressed again?
Also, while it is in Input.Pause, there is an annoying screen flash in a corner (waiting for input), I tried noecho and it still flashes. |
|
|
|
|
 |
Tony
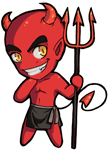
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Flying Carpet
|
Posted: Thu Jun 14, 2012 10:47 pm Post subject: RE:Highscores List |
|
|
Thanks for all the info Tony, but yes, I tried noecho before and it still happened. |
|
|
|
|
 |
|
|