Author |
Message |
Mithokey
|
Posted: Sat Apr 28, 2012 12:58 am Post subject: Compare Two Strings in if Statement |
|
|
I keep on getting an error for the line where it says if (pepperoni == YES);
Does anyone know how I can fix this? Thanks!
code: | package testjava;
import java.util.Scanner;
public class pizzaApp {
public void program() {
String pepperoni;
String mushroom;
int pizzaNumber;
double price;
double total;
Scanner input = new Scanner(System.in);
System.out.println("Enter YES if you want pepperoni on your pizza. Enter NO if you do not: ");
pepperoni = input.next();
System.out.println("Enter YES if you want mushrooms on your pizza. Enter NO if you do not: ");
mushroom = input.next();
System.out.println("Enter the number of pizzas you want: ");
pizzaNumber = input.nextInt();
if (pepperoni == YES) {
if (mushroom == YES) {
total = 1;
} else {
total = 0.5;
}
} else if (mushroom == YES) {
total = 0.5;
} else {
total = 0;
}
price = total + 10 * pizzaNumber;
System.out.println("Your total price comes to: $" + price);
}
} |
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
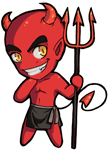
|
Posted: Sat Apr 28, 2012 10:47 pm Post subject: RE:Compare Two Strings in if Statement |
|
|
- what is the value of YES
- will the entered string be the same object as the literal that you are comparing against? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
joshm
|
Posted: Mon Apr 30, 2012 9:09 am Post subject: RE:Compare Two Strings in if Statement |
|
|
pepperoni.equals("YES") |
|
|
|
|
 |
wtd
|
Posted: Tue May 01, 2012 1:21 am Post subject: RE:Compare Two Strings in if Statement |
|
|
It is poor form to simply provide working code with no explanation.
The best thing to do is to respond with leading questions, as Tony has done. Failing that, you could at least provide an explanation as to why your working code provides the desired result. |
|
|
|
|
 |
TokenHerbz

|
Posted: Tue May 01, 2012 8:09 pm Post subject: RE:Compare Two Strings in if Statement |
|
|
//your code...
//if (pepperoni == YES) {
if (pepperoni == "YES") {
//that works...
//change all your YES to "YES"... |
|
|
|
|
 |
DemonWasp
|
Posted: Tue May 01, 2012 9:35 pm Post subject: RE:Compare Two Strings in if Statement |
|
|
@Token: That doesn't (always) work right in Java -- Strings are compared by reference equality if you use ==. To compare by content, you need to use .equals(), like every other class in Java. |
|
|
|
|
 |
TokenHerbz

|
Posted: Mon May 07, 2012 12:13 am Post subject: RE:Compare Two Strings in if Statement |
|
|
what if your checking a specific thing against something else specifically in memory, still better to use .equals()? |
|
|
|
|
 |
Tony
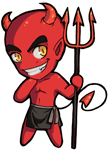
|
Posted: Mon May 07, 2012 1:03 am Post subject: RE:Compare Two Strings in if Statement |
|
|
If you are checking to see that two objects point to the same location in memory, use ==. Though with strings, that is almost never the correct thing to do. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
|