overloaded methods.
Author |
Message |
TokenHerbz

|
Posted: Wed Mar 28, 2012 8:08 pm Post subject: overloaded methods. |
|
|
is it normal programming practice to have a lot of over loaded methods?
It seems to be fine as a constructor in your classes that you make, but what about as actual methods that you'll be using.
Please shed some light to this, thank you. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Insectoid

|
Posted: Wed Mar 28, 2012 8:30 pm Post subject: RE:overloaded methods. |
|
|
Definitely. It's common practise to have a 'default' function that does a simple task (or nothing), that is then overridden in child classes. In this way, you can have an array of a superclass and invoke that method on each, where the subclasses have their own implementation.
Say you have a game. Everything that moves derives from the same superclass (let's call it an Entity). The Entity contains a move() method that does nothing. You then create a Person class, and override the move() method to add the Person's X and Y velocity to the X and Y values, and apply gravity. You might then create a thrown weapon class that spins as it moves, where you override the move() method to incorporate rotation. You might also have static platforms that do not override the move() method at all. |
|
|
|
|
 |
DemonWasp
|
Posted: Wed Mar 28, 2012 8:36 pm Post subject: RE:overloaded methods. |
|
|
It's relatively uncommon in practice. I would guess something like 1 in 5 classes have at least one overloaded method name. Many classes will have overloaded constructors, probably about 1 in 2.
That's not an argument for or against, though. The only argument there is: does it make sense?
Your method names should probably all be verbs. The object they are within is the 'do-er' and the arguments they take are the 'do-ee'. The method 'get' on 'List' takes 'int' because you are asking: "List, please get me element (int)". The only time you should overload a method is if there are two different ways to 'do' that verb.
A fairly common example is to have doFoo() that can operate on a default argument list and doFoo(args) that takes custom arguments. The first will just call the second with default arguments, but it's more convenient and easier to write.
It is conceivable that you would have objects that can operate on a lot of different things in essentially the same way, but I've never seen that in practice. In that case, you should probably move the operation from the central class that can do(x) and do(y) into x.do() and y.do(). |
|
|
|
|
 |
DemonWasp
|
Posted: Wed Mar 28, 2012 8:40 pm Post subject: RE:overloaded methods. |
|
|
@Insectoid: You're thinking of "override". "Overload" is different. |
|
|
|
|
 |
Insectoid

|
Posted: Wed Mar 28, 2012 8:47 pm Post subject: RE:overloaded methods. |
|
|
Oh crap, my bad. Overloaded methods are where methods take the same identifier, but different parameters and implementations, no? |
|
|
|
|
 |
Tony
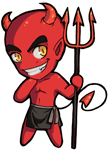
|
Posted: Wed Mar 28, 2012 8:58 pm Post subject: RE:overloaded methods. |
|
|
yup, overload is when you change the signature of a function by supplying it with different arguments.
I don't think it's common to have many overloaded versions of a method in the same class, as it likely suggests a problem with the design. A more generic approach, such as accepting a parent class for an argument, or using templates*, might be feasible and more appropriate.
* edit: was thinking of C++, before I noticed that this was posted in a Java forum. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
TokenHerbz

|
Posted: Wed Mar 28, 2012 9:00 pm Post subject: RE:overloaded methods. |
|
|
over riding isn't something that happens alot tho right? As i understand, each object gets a toString() inherits which displays the location of said object in memory. However i guess if your in the child class and you over ride that, to display details about your object, i guess thats okay.
Thats the only actual thing i can think of where over riding is good?! Are there other examples, i assume its best not to over ride things. |
|
|
|
|
 |
Tony
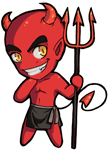
|
Posted: Wed Mar 28, 2012 9:14 pm Post subject: RE:overloaded methods. |
|
|
methods of an abstract class / interface are often overriden. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
DemonWasp
|
Posted: Wed Mar 28, 2012 11:41 pm Post subject: RE:overloaded methods. |
|
|
Actually, overriding happens much more frequently in practice than does overloading (at least, in my experience). Often, the overriding method will call the parent method first, then do some additional things.
If you need a type of thing (class) to have a behaviour (method), you put a definition in that class. If there are variations on that class that need to do different things, they override it. This is fairly common.
ArrayList overrides every method of List.
HashMap overrides every method of Map.
TreeSet overrides every method of Set.
Both overriding and overloading are language features for a reason. Without them, more or less all the use of OOP vanishes. They are not dangerous, slow, or difficult.
There are, however, several "code smells" (signs of poor code) that appear:
1. Overriding methods with the exact same definition as the parent.
2. Overriding methods and then immediately delegating to the parent (non-overridden) version without doing any additional work.
3. Overloading methods to accept every type under the Sun. This is usually fixed by having a better design or using generics (sorta like C++ templates).
4. Overloading methods and copy-pasting code between them with minor tweaks.
Most of those are of the "code duplication" type, but they're worth mentioning because they're very common.
Be careful when defining your class hierarchies too, so you don't end up with what we have at my workplace:
code: | interface com.foo.IView {}
class com.foo.View implements com.foo.IView {}
interface com.bar.IView extends com.foo.IView {}
class com.bar.View extends com.foo.View implements com.bar.IView {} |
I swear I had no part in that. |
|
|
|
|
 |
|
|