Moving Ball, Flicker Reduction
Author |
Message |
TheXploder

|
Posted: Mon Jan 19, 2004 11:06 pm Post subject: Moving Ball, Flicker Reduction |
|
|
There are many ways to update the position of a ball one of which is
'cls' which stands for 'clear screen' I'll give you a simple tutorial on how to reduce that flicker.
The code using cls:
code: |
var x : int := 0 % creates a new variable of integer type and sets the
% value to 0
proc objBall (x, y, ballSize, clr : int) % starts a procedure with the
% variable name 'objBall' and containing 'x, y, ballsize, clr' variables,
% which are considered to be local variables
drawfilloval (x + ballSize, y + ballSize, ballSize, ballSize, clr)
% draws an oval
end objBall
% ends the 'objBall' procedure
loop
objBall (x, 0, 10, 1) % "objBall's" variables that are contained can be
% called and given a value by using subscripts
delay (10)
x += 1 % increases x value by one each loop cycle
cls %clears the screen
end loop
%%%%And now using a better technigue:%%%%
var x : int := 0
proc objBall (x, y, ballSize, clr : int)
drawfilloval (x + ballSize, y + ballSize, ballSize, ballSize, clr)
end objBall
loop
objBall (x, 0, 10, 1)
% The colour of the ball here is represented by the last number...
delay (10)
x += 1
% Instead of using cls this time I'll just change the colour of the ball to 0
% or white
objBall (x, 0, 10, 0)
end loop
|
As you can see this reduces the fickering to a minimum. Because the screen is not redrawn completely, only were the ball previously was.
Hope this tutorial helps..
-=TheXploder=- |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
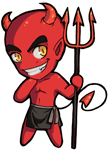
|
Posted: Mon Jan 19, 2004 11:27 pm Post subject: (No subject) |
|
|
or you could just use View.Update (or now that 4.0.5 is out, View.UpdateArea)
good job for trying though+Bits |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Skizzarz
|
Posted: Tue Jan 20, 2004 6:54 pm Post subject: (No subject) |
|
|
thx for the great tutorial, i used some of the code in my "hockey puck" program for school and all my classmates wonder how its so smooth  |
|
|
|
|
 |
Mazer

|
Posted: Tue Jan 20, 2004 7:51 pm Post subject: Re: Moving Ball, Flicker Reduction |
|
|
TheXploder wrote:
code: |
objBall (x, 0, 10, 1)
% The colour of the ball here is represented by the last number...
delay (10)
x += 1
% Instead of using cls this time I'll just change the colour of the ball to 0
% or white
objBall (x, 0, 10, 0)
|
You should have blanked out the ball before increasing the x coordinate, otherwise you get this blue streak.
code: |
objBall (x, 0, 10, 1)
% The colour of the ball here is represented by the last number...
delay (10)
% Instead of using cls this time I'll just change the colour of the ball to 0
% or white
objBall (x, 0, 10, 0)
x += 1
|
|
|
|
|
|
 |
LiquidDragon
|
Posted: Wed Feb 18, 2004 8:16 pm Post subject: (No subject) |
|
|
well couldnt your just do something like this?
code: |
View.Set ("offscreenonly")
var x : int := 0
proc objBall (x, y, ballSize, clr : int)
drawfilloval (x + ballSize, y + ballSize, ballSize, ballSize, clr)
end objBall
loop
objBall (x, 0, 10, 1)
View.Update
% The colour of the ball here is represented by the last number...
delay (10)
% Instead of using cls this time I'll just change the colour of the ball to 0
% or white
objBall (x, 0, 10, 0)
x += 1
end loop
|
|
|
|
|
|
 |
Kamikagushi

|
Posted: Thu Jun 10, 2004 4:34 pm Post subject: (No subject) |
|
|
~a question~after you use View.Update~how would you turn it off~ |
|
|
|
|
 |
Delos

|
Posted: Thu Jun 10, 2004 5:18 pm Post subject: (No subject) |
|
|
Repost your question in the Help forum, you'll get better answers... |
|
|
|
|
 |
nerdie
|
Posted: Mon Jun 21, 2004 3:06 pm Post subject: (No subject) |
|
|
does View.Set ("nooffscreenonly") exist?
and if it does did i spell it correctly? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
GlobeTrotter
|
Posted: Mon Jun 21, 2004 3:37 pm Post subject: (No subject) |
|
|
It does exist, you spelled it right. |
|
|
|
|
 |
naoki

|
Posted: Fri Jun 25, 2004 4:36 pm Post subject: (No subject) |
|
|
View.Update is merely a one time thing, like cls. It acts by basically showing you anything drawn on the screen before View.Update was called.
Thus, you may need to call View.Update in several places of your program in order to see different things.
As well, isn't Turing set by default to nooffscreenonly? So why bother unless you set to offscreen in the first place |
|
|
|
|
 |
|
|