Calling Methods From Different CLasses?
Author |
Message |
Krocker
|
Posted: Thu Jan 05, 2012 12:08 pm Post subject: Calling Methods From Different CLasses? |
|
|
hiya guys, ok so im creating this program that deletes certain files. I have 2 classes, the main program and dProcess (handles the deleting part). However, i keep getting this error when i am calling DProcess in the main program:
No application overload for the method named "main" was found in type "kcleaner.dProcess". Perhaps you wanted overload version "void main (java.lang.String[] argv) throws java.lang.Exception;" instead?
Heres my Code:
Main
code: |
package kcleaner;
import java.awt.event.*;
import javax.swing.*;
import java.io.*;
import java.awt.*;
public class Test extends JFrame implements ActionListener
{
public JFrame f1 = new JFrame ("KCleaner");
public JPanel p1 = new JPanel ();
public JButton b1 = new JButton ("Clean");
public JButton b2 = new JButton ("Close");
public Test ()
{
Image im = Toolkit.getDefaultToolkit ().getImage ("kcleaner/Icon.gif");
f1.setIconImage (im);
b1.addActionListener (this);
b2.addActionListener (this);
f1.setContentPane (p1);
f1.setSize (400, 400);
p1.add (b1);
p1.add (b2);
f1.setVisible (true);
}
public void actionPerformed (ActionEvent e)
{
dProcess method = new dProcess ();
if (e.getSource () == b1)
{
JOptionPane.showMessageDialog (null, "KCleaner is Done!");
method.main (); // THE ERROR IS COMING FROM HERE!!!!!!!!
}
else if (e.getSource () == b2)
{
JOptionPane.showMessageDialog (null, "Thank You For Using KCleaner");
f1.setVisible (false);
}
}
public static void main (String[] args)
{
new Test ();
}
}
|
dProcess
code: |
package kcleaner;
import java.awt.event.*;
import javax.swing.*;
import java.io.*;
public class dProcess
{
public static void main (String[] arg) throws Exception
{
String drive = JOptionPane.showInputDialog(null, "Please Enter Your Main Hard Drive Letter", "Hardrive", JOptionPane.QUESTION_MESSAGE );
// String user = JOptionPane.showInputDialog(null, "Please Enter The User Accout Name", "User Account", JOptionPane.QUESTION_MESSAGE );
String folder = drive.toUpperCase()+":\\Nexon/Combat Arms/BlackCipher";
boolean file = directory (new File (folder));
if (file == false)
{
JOptionPane.showMessageDialog (null, "Directory does not exist.");
}
else
{
}
}
public static boolean directory (File dir)
{
if (dir.isDirectory ())
{
String[] children = dir.list ();
for (int i = 0 ; i < children.length ; i++)
{
boolean success = directory (new File (dir, children [i]));
if (!success)
{
return false;
}
}
}
return dir.delete ();
}
}
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
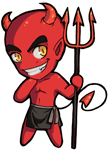
|
Posted: Thu Jan 05, 2012 12:23 pm Post subject: RE:Calling Methods From Different CLasses? |
|
|
looks like the method is expecting some arguments and you are not giving it any. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Krocker
|
Posted: Thu Jan 05, 2012 3:04 pm Post subject: Re: RE:Calling Methods From Different CLasses? |
|
|
Tony @ Thu Jan 05, 2012 12:23 pm wrote: looks like the method is expecting some arguments and you are not giving it any.
ok... but im not sure what im supposed to argue and how. |
|
|
|
|
 |
Tony
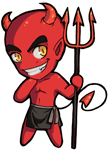
|
Posted: Thu Jan 05, 2012 3:24 pm Post subject: RE:Calling Methods From Different CLasses? |
|
|
Quote:
public static void main (String[] arg) throws Exception
an array of String |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Krocker
|
Posted: Thu Jan 05, 2012 6:14 pm Post subject: Re: RE:Calling Methods From Different CLasses? |
|
|
Tony @ Thu Jan 05, 2012 3:24 pm wrote: Quote:
public static void main (String[] arg) throws Exception
an array of String
so i would do
public static void main (String[] arg) throws Exception
{
Method.main()
} |
|
|
|
|
 |
Tony
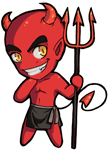
|
Posted: Thu Jan 05, 2012 6:21 pm Post subject: RE:Calling Methods From Different CLasses? |
|
|
I don't know. What kind of arguments (if any?) does Method.main() expect? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Krocker
|
Posted: Thu Jan 05, 2012 7:37 pm Post subject: Re: RE:Calling Methods From Different CLasses? |
|
|
Tony @ Thu Jan 05, 2012 6:21 pm wrote: I don't know. What kind of arguments (if any?) does Method.main() expect?
oh.... im new here so ya... heres the coding for that:
code: |
package kcleaner;
import java.awt.event.*;
import javax.swing.*;
import java.io.*;
import java.awt.*;
public class Test extends JFrame implements ActionListener
{
public JFrame f1 = new JFrame ("KCleaner");
public JPanel p1 = new JPanel ();
public JButton b1 = new JButton ("Clean");
public JButton b2 = new JButton ("Close");
public Test ()
{
Image im = Toolkit.getDefaultToolkit ().getImage ("kcleaner/Icon.gif");
f1.setIconImage (im);
b1.addActionListener (this);
b2.addActionListener (this);
f1.setContentPane (p1);
f1.setSize (400, 400);
p1.add (b1);
p1.add (b2);
f1.setVisible (true);
}
public void actionPerformed (ActionEvent e)
{
dProcess method = new dProcess ();
if (e.getSource () == b1)
{
JOptionPane.showMessageDialog (null, "KCleaner is Done!");
method.directory (File );
}
else if (e.getSource () == b2)
{
JOptionPane.showMessageDialog (null, "Thank You For Using KCleaner");
f1.setVisible (false);
}
}
public static void main (String[] args)
{
new Test ();
}
}
|
|
|
|
|
|
 |
Tony
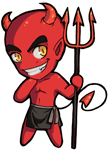
|
Posted: Thu Jan 05, 2012 7:40 pm Post subject: RE:Calling Methods From Different CLasses? |
|
|
I was hoping you could figure out the answer to that question on your own. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
Krocker
|
Posted: Thu Jan 05, 2012 7:42 pm Post subject: Re: Calling Methods From Different CLasses? |
|
|
srry wrong codes, heres the right one
code: | package kcleaner;
import java.awt.event.*;
import javax.swing.*;
import java.io.*;
public class dProcess
{
public static void main (String[] arg) throws Exception
{
String drive = JOptionPane.showInputDialog(null, "Please Enter Your Main Hard Drive Letter", "Hardrive", JOptionPane.QUESTION_MESSAGE );
// String user = JOptionPane.showInputDialog(null, "Please Enter The User Accout Name", "User Account", JOptionPane.QUESTION_MESSAGE );
String folder = drive.toUpperCase()+":\\Nexon/Combat Arms/BlackCipher";
boolean file = directory (new File (folder));
if (file == false)
{
JOptionPane.showMessageDialog (null, "Directory does not exist.");
}
else
{
}
}
public static boolean directory (File dir)
{
if (dir.isDirectory ())
{
String[] children = dir.list ();
for (int i = 0 ; i < children.length ; i++)
{
boolean success = directory (new File (dir, children [i]));
if (!success)
{
return false;
}
}
}
return dir.delete ();
}
}
|
|
|
|
|
|
 |
Krocker
|
Posted: Thu Jan 05, 2012 7:43 pm Post subject: Re: RE:Calling Methods From Different CLasses? |
|
|
Tony @ Thu Jan 05, 2012 7:40 pm wrote: I was hoping you could figure out the answer to that question on your own.
i have tried, it has been 1 month of research and such, but nothing has come up. Im frustrated! |
|
|
|
|
 |
Tony
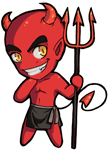
|
Posted: Thu Jan 05, 2012 8:01 pm Post subject: RE:Calling Methods From Different CLasses? |
|
|
lets step back to the basics then.
- functions -- http://en.wikipedia.org/wiki/Subroutine
- functions with parameters -- http://en.wikipedia.org/wiki/Parameter_(computer_programming)
the example given in those pages is
code: |
double sales_tax(double price)
{
return 0.05 * price;
}
|
which can be invoked as
which will take 10.00, assign it to "price", and compute the result of 0.5. This makes sense so far, but we could also throw other random values at the function
code: |
sales_tax("derp");
sales_tax();
|
What does it mean to take 5% of a word or of nothing? I don't know. I actually don't care. The function specifies the type and number of parameters (in this case, just one double), and so the compiler will make sure that when you ask for a function to receive some values, that you don't forget to give those specific types of values later on. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
|
|