Realm Of The Mad God / MiniCraft Terrain System [Incomplete] [Source]
Author |
Message |
pers2981
|
Posted: Tue Jan 03, 2012 10:53 pm Post subject: Realm Of The Mad God / MiniCraft Terrain System [Incomplete] [Source] |
|
|
NOTE: This is a very 'Odd' way of making a terrain generation system; It was my first go at it and I have learned a ton since the start.
What is it?
A some what random terrain generation system / tile engine.
What do you mean "Some what random"?
Well its not completely random. If it were then there would be grass 'blocks' in the middle of the ocean and water randomly flooding your island.
In the title it says '[Incomplete] whats the deal with that?
Well I started this mini project planning to finish it as a whole game, but I realise now that my main goal isnt possible with turing. It requires a quicker/more powerful language to keep up with all the crap I want to do with it. Since its incomplete it has a bug or two.
What are said bugs?
Well I didn't finish coding the map boundaries for 'Left' and 'up' so if you attempt to go far left or far up you will receive an error. I have fixed said error for down and right. It should be easy to fix. There is most likely another bug or two but I have yet to find it.
Screen shot please?
No, Not now at least. I'm lazy.
Download please?
No, you get to copy + paste it.
Turing: |
%===========================================================================%
% = == = == = == = == = == = Initial Setup = == = == = == = == = == = == = %
%===========================================================================%
import GUI
setscreen ("graphics:500;500,nobuttonbar,offscreenonly")
View.Set ("title:Realm Of The Mad God Clone")
var SpawnX : int := maxx div 2
var SpawnY : int := maxy div 2
var CharX : int := 100
var CharY : int := 0
var RowX : int := 0
var RowY : int := 0
var TileType : array 1 .. 625 of string
var TempColor : int := 0
var TempOdds : int := 0
var PHealth : int := 0
var chars : array char of boolean
var WorldX : int := 0
var WorldY : int := 0
for i : 1 .. 625
TileType (i ) := "Water"
end for
%===========================================================================%
% = == = == = == = == = == = GenerateWorld = == = == = == = == = == = == = %
%===========================================================================%
procedure GenerateWorld
for i : 1 .. 625
TempOdds := 0
if RowX > 24 then
RowX := 0
RowY := RowY + 1
end if
if RowX = 0 or RowX = 24 or RowY = 0 or RowY = 24 then
TileType (i ) := "Water"
else
if TileType (i - 1) = "Water" and TileType (i - 10) = "Water" then
TempOdds := TempOdds + 50 + Rand.Int (0, 50)
if TempOdds > 75 then
TileType (i ) := "Water"
end if
if TempOdds < 75 then
TileType (i ) := "Sand"
end if
end if
if TileType (i - 1) = "Sand" and TileType (i - 10) = "Water" then
TempOdds := TempOdds + 50 + Rand.Int (0, 50)
if TempOdds > 90 then
TileType (i ) := "Water"
end if
if TempOdds < 90 then
TileType (i ) := "Sand"
end if
end if
if TileType (i - 1) = "Water" and TileType (i - 10) = "Sand" then
TempOdds := TempOdds + 50 + Rand.Int (0, 50)
if TempOdds > 95 then
TileType (i ) := "Water"
end if
if TempOdds < 95 then
TileType (i ) := "Sand"
end if
end if
if TileType (i - 1) = "Sand" and TileType (i - 10) = "Sand" then
TempOdds := TempOdds + 50 + Rand.Int (0, 50)
if TempOdds > 95 then
TileType (i ) := "Water"
end if
if TempOdds < 95 then
TileType (i ) := "Sand"
end if
end if
end if
RowX := RowX + 1
end for
end GenerateWorld
%===========================================================================%
% = == = == = == = == = == = Draw World = == = == = == = == = == = == = %
%===========================================================================%
var MapInt : int := 1
var MapIntX : int := 0
var MapIntY : int := 0
proc DrawWorld
if MapIntY > - 1 then
if TileType (MapInt ) = "Sand" then
Draw.FillBox (0 * 50, 0 * 50, 0 * 50 + 50, 0 * 50 + 50, 14)
elsif TileType (MapInt ) = "Water" then
Draw.FillBox (0 * 50, 0 * 50, 0 * 50 + 50, 0 * 50 + 50, 100)
end if
if TileType (MapInt + 1) = "Sand" then
Draw.FillBox (1 * 50, 0 * 50, 1 * 50 + 50, 0 * 50 + 50, 14)
elsif TileType (MapInt + 1) = "Water" then
Draw.FillBox (1 * 50, 0 * 50, 1 * 50 + 50, 0 * 50 + 50, 100)
end if
if TileType (MapInt + 2) = "Sand" then
Draw.FillBox (2 * 50, 0 * 50, 2 * 50 + 50, 0 * 50 + 50, 14)
elsif TileType (MapInt + 2) = "Water" then
Draw.FillBox (2 * 50, 0 * 50, 2 * 50 + 50, 0 * 50 + 50, 100)
end if
if TileType (MapInt + 3) = "Sand" then
Draw.FillBox (3 * 50, 0 * 50, 3 * 50 + 50, 0 * 50 + 50, 14)
elsif TileType (MapInt + 3) = "Water" then
Draw.FillBox (3 * 50, 0 * 50, 3 * 50 + 50, 0 * 50 + 50, 100)
end if
if TileType (MapInt + 4) = "Sand" then
Draw.FillBox (4 * 50, 0 * 50, 4 * 50 + 50, 0 * 50 + 50, 14)
elsif TileType (MapInt + 4) = "Water" then
Draw.FillBox (4 * 50, 0 * 50, 4 * 50 + 50, 0 * 50 + 50, 100)
end if
if TileType (MapInt + 5) = "Sand" then
Draw.FillBox (5 * 50, 0 * 50, 5 * 50 + 50, 0 * 50 + 50, 14)
elsif TileType (MapInt + 5) = "Water" then
Draw.FillBox (5 * 50, 0 * 50, 5 * 50 + 50, 0 * 50 + 50, 100)
end if
if TileType (MapInt + 6) = "Sand" then
Draw.FillBox (6 * 50, 0 * 50, 6 * 50 + 50, 0 * 50 + 50, 14)
elsif TileType (MapInt + 6) = "Water" then
Draw.FillBox (6 * 50, 0 * 50, 6 * 50 + 50, 0 * 50 + 50, 100)
end if
if TileType (MapInt + 7) = "Sand" then
Draw.FillBox (7 * 50, 0 * 50, 7 * 50 + 50, 0 * 50 + 50, 14)
elsif TileType (MapInt + 7) = "Water" then
Draw.FillBox (7 * 50, 0 * 50, 7 * 50 + 50, 0 * 50 + 50, 100)
end if
if TileType (MapInt + 8) = "Sand" then
Draw.FillBox (8 * 50, 0 * 50, 8 * 50 + 50, 0 * 50 + 50, 14)
elsif TileType (MapInt + 8) = "Water" then
Draw.FillBox (8 * 50, 0 * 50, 8 * 50 + 50, 0 * 50 + 50, 100)
end if
if TileType (MapInt + 9) = "Sand" then
Draw.FillBox (9 * 50, 0 * 50, 9 * 50 + 50, 0 * 50 + 50, 14)
elsif TileType (MapInt + 9) = "Water" then
Draw.FillBox (9 * 50, 0 * 50, 9 * 50 + 50, 0 * 50 + 50, 100)
end if
end if
if MapIntY > - 2 then
if TileType (MapInt + 25) = "Sand" then
Draw.FillBox (0 * 50, 1 * 50, 0 * 50 + 50, 1 * 50 + 50, 14)
elsif TileType (MapInt + 25) = "Water" then
Draw.FillBox (0 * 50, 1 * 50, 0 * 50 + 50, 1 * 50 + 50, 100)
end if
if TileType (MapInt + 26) = "Sand" then
Draw.FillBox (1 * 50, 1 * 50, 1 * 50 + 50, 1 * 50 + 50, 14)
elsif TileType (MapInt + 26) = "Water" then
Draw.FillBox (1 * 50, 1 * 50, 1 * 50 + 50, 1 * 50 + 50, 100)
end if
if TileType (MapInt + 27) = "Sand" then
Draw.FillBox (2 * 50, 1 * 50, 2 * 50 + 50, 1 * 50 + 50, 14)
elsif TileType (MapInt + 27) = "Water" then
Draw.FillBox (2 * 50, 1 * 50, 2 * 50 + 50, 1 * 50 + 50, 100)
end if
if TileType (MapInt + 28) = "Sand" then
Draw.FillBox (3 * 50, 1 * 50, 3 * 50 + 50, 1 * 50 + 50, 14)
elsif TileType (MapInt + 28) = "Water" then
Draw.FillBox (3 * 50, 1 * 50, 3 * 50 + 50, 1 * 50 + 50, 100)
end if
if TileType (MapInt + 29) = "Sand" then
Draw.FillBox (4 * 50, 1 * 50, 4 * 50 + 50, 1 * 50 + 50, 14)
elsif TileType (MapInt + 29) = "Water" then
Draw.FillBox (4 * 50, 1 * 50, 4 * 50 + 50, 1 * 50 + 50, 100)
end if
if TileType (MapInt + 30) = "Sand" then
Draw.FillBox (5 * 50, 1 * 50, 5 * 50 + 50, 1 * 50 + 50, 14)
elsif TileType (MapInt + 30) = "Water" then
Draw.FillBox (5 * 50, 1 * 50, 5 * 50 + 50, 1 * 50 + 50, 100)
end if
if TileType (MapInt + 31) = "Sand" then
Draw.FillBox (6 * 50, 1 * 50, 6 * 50 + 50, 1 * 50 + 50, 14)
elsif TileType (MapInt + 31) = "Water" then
Draw.FillBox (6 * 50, 1 * 50, 6 * 50 + 50, 1 * 50 + 50, 100)
end if
if TileType (MapInt + 32) = "Sand" then
Draw.FillBox (7 * 50, 1 * 50, 7 * 50 + 50, 1 * 50 + 50, 14)
elsif TileType (MapInt + 32) = "Water" then
Draw.FillBox (7 * 50, 1 * 50, 7 * 50 + 50, 1 * 50 + 50, 100)
end if
if TileType (MapInt + 33) = "Sand" then
Draw.FillBox (8 * 50, 1 * 50, 8 * 50 + 50, 1 * 50 + 50, 14)
elsif TileType (MapInt + 33) = "Water" then
Draw.FillBox (8 * 50, 1 * 50, 8 * 50 + 50, 1 * 50 + 50, 100)
end if
if TileType (MapInt + 34) = "Sand" then
Draw.FillBox (9 * 50, 1 * 50, 9 * 50 + 50, 1 * 50 + 50, 14)
elsif TileType (MapInt + 34) = "Water" then
Draw.FillBox (9 * 50, 1 * 50, 9 * 50 + 50, 1 * 50 + 50, 100)
end if
end if
if MapIntY > - 3 then
if TileType (MapInt + 50) = "Sand" then
Draw.FillBox (0 * 50, 2 * 50, 0 * 50 + 50, 2 * 50 + 50, 14)
elsif TileType (MapInt + 50) = "Water" then
Draw.FillBox (0 * 50, 2 * 50, 0 * 50 + 50, 2 * 50 + 50, 100)
end if
if TileType (MapInt + 51) = "Sand" then
Draw.FillBox (1 * 50, 2 * 50, 1 * 50 + 50, 2 * 50 + 50, 14)
elsif TileType (MapInt + 51) = "Water" then
Draw.FillBox (1 * 50, 2 * 50, 1 * 50 + 50, 2 * 50 + 50, 100)
end if
if TileType (MapInt + 52) = "Sand" then
Draw.FillBox (2 * 50, 2 * 50, 2 * 50 + 50, 2 * 50 + 50, 14)
elsif TileType (MapInt + 52) = "Water" then
Draw.FillBox (2 * 50, 2 * 50, 2 * 50 + 50, 2 * 50 + 50, 100)
end if
if TileType (MapInt + 53) = "Sand" then
Draw.FillBox (3 * 50, 2 * 50, 3 * 50 + 50, 2 * 50 + 50, 14)
elsif TileType (MapInt + 53) = "Water" then
Draw.FillBox (3 * 50, 2 * 50, 3 * 50 + 50, 2 * 50 + 50, 100)
end if
if TileType (MapInt + 54) = "Sand" then
Draw.FillBox (4 * 50, 2 * 50, 4 * 50 + 50, 2 * 50 + 50, 14)
elsif TileType (MapInt + 54) = "Water" then
Draw.FillBox (4 * 50, 2 * 50, 4 * 50 + 50, 2 * 50 + 50, 100)
end if
if TileType (MapInt + 55) = "Sand" then
Draw.FillBox (5 * 50, 2 * 50, 5 * 50 + 50, 2 * 50 + 50, 14)
elsif TileType (MapInt + 55) = "Water" then
Draw.FillBox (5 * 50, 2 * 50, 5 * 50 + 50, 2 * 50 + 50, 100)
end if
if TileType (MapInt + 56) = "Sand" then
Draw.FillBox (6 * 50, 2 * 50, 6 * 50 + 50, 2 * 50 + 50, 14)
elsif TileType (MapInt + 56) = "Water" then
Draw.FillBox (6 * 50, 2 * 50, 6 * 50 + 50, 2 * 50 + 50, 100)
end if
if TileType (MapInt + 57) = "Sand" then
Draw.FillBox (7 * 50, 2 * 50, 7 * 50 + 50, 2 * 50 + 50, 14)
elsif TileType (MapInt + 57) = "Water" then
Draw.FillBox (7 * 50, 2 * 50, 7 * 50 + 50, 2 * 50 + 50, 100)
end if
if TileType (MapInt + 58) = "Sand" then
Draw.FillBox (8 * 50, 2 * 50, 8 * 50 + 50, 2 * 50 + 50, 14)
elsif TileType (MapInt + 58) = "Water" then
Draw.FillBox (8 * 50, 2 * 50, 8 * 50 + 50, 2 * 50 + 50, 100)
end if
if TileType (MapInt + 59) = "Sand" then
Draw.FillBox (9 * 50, 2 * 50, 9 * 50 + 50, 2 * 50 + 50, 14)
elsif TileType (MapInt + 59) = "Water" then
Draw.FillBox (9 * 50, 2 * 50, 9 * 50 + 50, 2 * 50 + 50, 100)
end if
end if
if MapIntY > - 3 then
if TileType (MapInt + 75) = "Sand" then
Draw.FillBox (0 * 50, 3 * 50, 0 * 50 + 50, 3 * 50 + 50, 14)
elsif TileType (MapInt + 74) = "Water" then
Draw.FillBox (0 * 50, 3 * 50, 0 * 50 + 50, 3 * 50 + 50, 100)
end if
if TileType (MapInt + 76) = "Sand" then
Draw.FillBox (1 * 50, 3 * 50, 1 * 50 + 50, 3 * 50 + 50, 14)
elsif TileType (MapInt + 76) = "Water" then
Draw.FillBox (1 * 50, 3 * 50, 1 * 50 + 50, 3 * 50 + 50, 100)
end if
if TileType (MapInt + 77) = "Sand" then
Draw.FillBox (2 * 50, 3 * 50, 2 * 50 + 50, 3 * 50 + 50, 14)
elsif TileType (MapInt + 77) = "Water" then
Draw.FillBox (2 * 50, 3 * 50, 2 * 50 + 50, 3 * 50 + 50, 100)
end if
if TileType (MapInt + 78) = "Sand" then
Draw.FillBox (3 * 50, 3 * 50, 3 * 50 + 50, 3 * 50 + 50, 14)
elsif TileType (MapInt + 78) = "Water" then
Draw.FillBox (3 * 50, 3 * 50, 3 * 50 + 50, 3 * 50 + 50, 100)
end if
if TileType (MapInt + 79) = "Sand" then
Draw.FillBox (4 * 50, 3 * 50, 4 * 50 + 50, 3 * 50 + 50, 14)
elsif TileType (MapInt + 79) = "Water" then
Draw.FillBox (4 * 50, 3 * 50, 4 * 50 + 50, 3 * 50 + 50, 100)
end if
if TileType (MapInt + 80) = "Sand" then
Draw.FillBox (5 * 50, 3 * 50, 5 * 50 + 50, 3 * 50 + 50, 14)
elsif TileType (MapInt + 80) = "Water" then
Draw.FillBox (5 * 50, 3 * 50, 5 * 50 + 50, 3 * 50 + 50, 100)
end if
if TileType (MapInt + 81) = "Sand" then
Draw.FillBox (6 * 50, 3 * 50, 6 * 50 + 50, 3 * 50 + 50, 14)
elsif TileType (MapInt + 81) = "Water" then
Draw.FillBox (6 * 50, 3 * 50, 6 * 50 + 50, 3 * 50 + 50, 100)
end if
if TileType (MapInt + 82) = "Sand" then
Draw.FillBox (7 * 50, 3 * 50, 7 * 50 + 50, 3 * 50 + 50, 14)
elsif TileType (MapInt + 82) = "Water" then
Draw.FillBox (7 * 50, 3 * 50, 7 * 50 + 50, 3 * 50 + 50, 100)
end if
if TileType (MapInt + 83) = "Sand" then
Draw.FillBox (8 * 50, 3 * 50, 8 * 50 + 50, 3 * 50 + 50, 14)
elsif TileType (MapInt + 83) = "Water" then
Draw.FillBox (8 * 50, 3 * 50, 8 * 50 + 50, 3 * 50 + 50, 100)
end if
if TileType (MapInt + 84) = "Sand" then
Draw.FillBox (9 * 50, 3 * 50, 9 * 50 + 50, 3 * 50 + 50, 14)
elsif TileType (MapInt + 84) = "Water" then
Draw.FillBox (9 * 50, 3 * 50, 9 * 50 + 50, 3 * 50 + 50, 100)
end if
end if
if TileType (MapInt + 100) = "Sand" then
Draw.FillBox (0 * 50, 4 * 50, 0 * 50 + 50, 4 * 50 + 50, 14)
elsif TileType (MapInt + 100) = "Water" then
Draw.FillBox (0 * 50, 4 * 50, 0 * 50 + 50, 4 * 50 + 50, 100)
end if
if TileType (MapInt + 101) = "Sand" then
Draw.FillBox (1 * 50, 4 * 50, 1 * 50 + 50, 4 * 50 + 50, 14)
elsif TileType (MapInt + 101) = "Water" then
Draw.FillBox (1 * 50, 4 * 50, 1 * 50 + 50, 4 * 50 + 50, 100)
end if
if TileType (MapInt + 102) = "Sand" then
Draw.FillBox (2 * 50, 4 * 50, 2 * 50 + 50, 4 * 50 + 50, 14)
elsif TileType (MapInt + 102) = "Water" then
Draw.FillBox (2 * 50, 4 * 50, 2 * 50 + 50, 4 * 50 + 50, 100)
end if
if TileType (MapInt + 103) = "Sand" then
Draw.FillBox (3 * 50, 4 * 50, 3 * 50 + 50, 4 * 50 + 50, 14)
elsif TileType (MapInt + 103) = "Water" then
Draw.FillBox (3 * 50, 4 * 50, 3 * 50 + 50, 4 * 50 + 50, 100)
end if
if TileType (MapInt + 104) = "Sand" then
Draw.FillBox (4 * 50, 4 * 50, 4 * 50 + 50, 4 * 50 + 50, 14)
elsif TileType (MapInt + 104) = "Water" then
Draw.FillBox (4 * 50, 4 * 50, 4 * 50 + 50, 4 * 50 + 50, 100)
end if
if TileType (MapInt + 105) = "Sand" then
Draw.FillBox (5 * 50, 4 * 50, 5 * 50 + 50, 4 * 50 + 50, 14)
elsif TileType (MapInt + 105) = "Water" then
Draw.FillBox (5 * 50, 4 * 50, 5 * 50 + 50, 4 * 50 + 50, 100)
end if
if TileType (MapInt + 106) = "Sand" then
Draw.FillBox (6 * 50, 4 * 50, 6 * 50 + 50, 4 * 50 + 50, 14)
elsif TileType (MapInt + 106) = "Water" then
Draw.FillBox (6 * 50, 4 * 50, 6 * 50 + 50, 4 * 50 + 50, 100)
end if
if TileType (MapInt + 107) = "Sand" then
Draw.FillBox (7 * 50, 4 * 50, 7 * 50 + 50, 4 * 50 + 50, 14)
elsif TileType (MapInt + 107) = "Water" then
Draw.FillBox (7 * 50, 4 * 50, 7 * 50 + 50, 4 * 50 + 50, 100)
end if
if TileType (MapInt + 108) = "Sand" then
Draw.FillBox (8 * 50, 4 * 50, 8 * 50 + 50, 4 * 50 + 50, 14)
elsif TileType (MapInt + 108) = "Water" then
Draw.FillBox (8 * 50, 4 * 50, 8 * 50 + 50, 4 * 50 + 50, 100)
end if
if TileType (MapInt + 109) = "Sand" then
Draw.FillBox (9 * 50, 4 * 50, 9 * 50 + 50, 4 * 50 + 50, 14)
elsif TileType (MapInt + 109) = "Water" then
Draw.FillBox (9 * 50, 4 * 50, 9 * 50 + 50, 4 * 50 + 50, 100)
end if
if TileType (MapInt + 125) = "Sand" then
Draw.FillBox (0 * 50, 5 * 50, 0 * 50 + 50, 5 * 50 + 50, 14)
elsif TileType (MapInt + 125) = "Water" then
Draw.FillBox (0 * 50, 5 * 50, 0 * 50 + 50, 5 * 50 + 50, 100)
end if
if TileType (MapInt + 126) = "Sand" then
Draw.FillBox (1 * 50, 5 * 50, 1 * 50 + 50, 5 * 50 + 50, 14)
elsif TileType (MapInt + 126) = "Water" then
Draw.FillBox (1 * 50, 5 * 50, 1 * 50 + 50, 5 * 50 + 50, 100)
end if
if TileType (MapInt + 127) = "Sand" then
Draw.FillBox (2 * 50, 5 * 50, 2 * 50 + 50, 5 * 50 + 50, 14)
elsif TileType (MapInt + 127) = "Water" then
Draw.FillBox (2 * 50, 5 * 50, 2 * 50 + 50, 5 * 50 + 50, 100)
end if
if TileType (MapInt + 128) = "Sand" then
Draw.FillBox (3 * 50, 5 * 50, 3 * 50 + 50, 5 * 50 + 50, 14)
elsif TileType (MapInt + 128) = "Water" then
Draw.FillBox (3 * 50, 5 * 50, 3 * 50 + 50, 5 * 50 + 50, 100)
end if
if TileType (MapInt + 129) = "Sand" then
Draw.FillBox (4 * 50, 5 * 50, 4 * 50 + 50, 5 * 50 + 50, 14)
elsif TileType (MapInt + 129) = "Water" then
Draw.FillBox (4 * 50, 5 * 50, 4 * 50 + 50, 5 * 50 + 50, 100)
end if
if TileType (MapInt + 130) = "Sand" then
Draw.FillBox (5 * 50, 5 * 50, 5 * 50 + 50, 5 * 50 + 50, 14)
elsif TileType (MapInt + 130) = "Water" then
Draw.FillBox (5 * 50, 5 * 50, 5 * 50 + 50, 5 * 50 + 50, 100)
end if
if TileType (MapInt + 131) = "Sand" then
Draw.FillBox (6 * 50, 5 * 50, 6 * 50 + 50, 5 * 50 + 50, 14)
elsif TileType (MapInt + 131) = "Water" then
Draw.FillBox (6 * 50, 5 * 50, 6 * 50 + 50, 5 * 50 + 50, 100)
end if
if TileType (MapInt + 132) = "Sand" then
Draw.FillBox (7 * 50, 5 * 50, 7 * 50 + 50, 5 * 50 + 50, 14)
elsif TileType (MapInt + 132) = "Water" then
Draw.FillBox (7 * 50, 5 * 50, 7 * 50 + 50, 5 * 50 + 50, 100)
end if
if TileType (MapInt + 133) = "Sand" then
Draw.FillBox (8 * 50, 5 * 50, 8 * 50 + 50, 5 * 50 + 50, 14)
elsif TileType (MapInt + 133) = "Water" then
Draw.FillBox (8 * 50, 5 * 50, 8 * 50 + 50, 5 * 50 + 50, 100)
end if
if TileType (MapInt + 134) = "Sand" then
Draw.FillBox (9 * 50, 5 * 50, 9 * 50 + 50, 5 * 50 + 50, 14)
elsif TileType (MapInt + 134) = "Water" then
Draw.FillBox (9 * 50, 5 * 50, 9 * 50 + 50, 5 * 50 + 50, 100)
end if
if TileType (MapInt + 150) = "Sand" then
Draw.FillBox (0 * 50, 6 * 50, 0 * 50 + 50, 6 * 50 + 50, 14)
elsif TileType (MapInt + 150) = "Water" then
Draw.FillBox (0 * 50, 6 * 50, 0 * 50 + 50, 6 * 50 + 50, 100)
end if
if TileType (MapInt + 151) = "Sand" then
Draw.FillBox (1 * 50, 6 * 50, 1 * 50 + 50, 6 * 50 + 50, 14)
elsif TileType (MapInt + 151) = "Water" then
Draw.FillBox (1 * 50, 6 * 50, 1 * 50 + 50, 6 * 50 + 50, 100)
end if
if TileType (MapInt + 152) = "Sand" then
Draw.FillBox (2 * 50, 6 * 50, 2 * 50 + 50, 6 * 50 + 50, 14)
elsif TileType (MapInt + 152) = "Water" then
Draw.FillBox (2 * 50, 6 * 50, 2 * 50 + 50, 6 * 50 + 50, 100)
end if
if TileType (MapInt + 153) = "Sand" then
Draw.FillBox (3 * 50, 6 * 50, 3 * 50 + 50, 6 * 50 + 50, 14)
elsif TileType (MapInt + 153) = "Water" then
Draw.FillBox (3 * 50, 6 * 50, 3 * 50 + 50, 6 * 50 + 50, 100)
end if
if TileType (MapInt + 154) = "Sand" then
Draw.FillBox (4 * 50, 6 * 50, 4 * 50 + 50, 6 * 50 + 50, 14)
elsif TileType (MapInt + 154) = "Water" then
Draw.FillBox (4 * 50, 6 * 50, 4 * 50 + 50, 6 * 50 + 50, 100)
end if
if TileType (MapInt + 155) = "Sand" then
Draw.FillBox (5 * 50, 6 * 50, 5 * 50 + 50, 6 * 50 + 50, 14)
elsif TileType (MapInt + 155) = "Water" then
Draw.FillBox (5 * 50, 6 * 50, 5 * 50 + 50, 6 * 50 + 50, 100)
end if
if TileType (MapInt + 156) = "Sand" then
Draw.FillBox (6 * 50, 6 * 50, 6 * 50 + 50, 6 * 50 + 50, 14)
elsif TileType (MapInt + 151) = "Water" then
Draw.FillBox (6 * 50, 6 * 50, 6 * 50 + 50, 6 * 50 + 50, 100)
end if
if TileType (MapInt + 157) = "Sand" then
Draw.FillBox (7 * 50, 6 * 50, 7 * 50 + 50, 6 * 50 + 50, 14)
elsif TileType (MapInt + 151) = "Water" then
Draw.FillBox (7 * 50, 6 * 50, 7 * 50 + 50, 6 * 50 + 50, 100)
end if
if TileType (MapInt + 158) = "Sand" then
Draw.FillBox (8 * 50, 6 * 50, 8 * 50 + 50, 6 * 50 + 50, 14)
elsif TileType (MapInt + 151) = "Water" then
Draw.FillBox (8 * 50, 6 * 50, 8 * 50 + 50, 6 * 50 + 50, 100)
end if
if TileType (MapInt + 159) = "Sand" then
Draw.FillBox (9 * 50, 6 * 50, 9 * 50 + 50, 6 * 50 + 50, 14)
elsif TileType (MapInt + 151) = "Water" then
Draw.FillBox (9 * 50, 6 * 50, 9 * 50 + 50, 6 * 50 + 50, 100)
end if
if TileType (MapInt + 175) = "Sand" then
Draw.FillBox (0 * 50, 7 * 50, 0 * 50 + 50, 7 * 50 + 50, 14)
elsif TileType (MapInt + 175) = "Water" then
Draw.FillBox (0 * 50, 7 * 50, 0 * 50 + 50, 7 * 50 + 50, 100)
end if
if TileType (MapInt + 176) = "Sand" then
Draw.FillBox (1 * 50, 7 * 50, 1 * 50 + 50, 7 * 50 + 50, 14)
elsif TileType (MapInt + 176) = "Water" then
Draw.FillBox (1 * 50, 7 * 50, 1 * 50 + 50, 7 * 50 + 50, 100)
end if
if TileType (MapInt + 177) = "Sand" then
Draw.FillBox (2 * 50, 7 * 50, 2 * 50 + 50, 7 * 50 + 50, 14)
elsif TileType (MapInt + 177) = "Water" then
Draw.FillBox (2 * 50, 7 * 50, 2 * 50 + 50, 7 * 50 + 50, 100)
end if
if TileType (MapInt + 178) = "Sand" then
Draw.FillBox (3 * 50, 7 * 50, 3 * 50 + 50, 7 * 50 + 50, 14)
elsif TileType (MapInt + 178) = "Water" then
Draw.FillBox (3 * 50, 7 * 50, 3 * 50 + 50, 7 * 50 + 50, 100)
end if
if TileType (MapInt + 179) = "Sand" then
Draw.FillBox (4 * 50, 7 * 50, 4 * 50 + 50, 7 * 50 + 50, 14)
elsif TileType (MapInt + 179) = "Water" then
Draw.FillBox (4 * 50, 7 * 50, 4 * 50 + 50, 7 * 50 + 50, 100)
end if
if TileType (MapInt + 180) = "Sand" then
Draw.FillBox (5 * 50, 7 * 50, 5 * 50 + 50, 7 * 50 + 50, 14)
elsif TileType (MapInt + 180) = "Water" then
Draw.FillBox (5 * 50, 7 * 50, 5 * 50 + 50, 7 * 50 + 50, 100)
end if
if TileType (MapInt + 181) = "Sand" then
Draw.FillBox (6 * 50, 7 * 50, 6 * 50 + 50, 7 * 50 + 50, 14)
elsif TileType (MapInt + 181) = "Water" then
Draw.FillBox (6 * 50, 7 * 50, 6 * 50 + 50, 7 * 50 + 50, 100)
end if
if TileType (MapInt + 182) = "Sand" then
Draw.FillBox (7 * 50, 7 * 50, 7 * 50 + 50, 7 * 50 + 50, 14)
elsif TileType (MapInt + 182) = "Water" then
Draw.FillBox (7 * 50, 7 * 50, 7 * 50 + 50, 7 * 50 + 50, 100)
end if
if TileType (MapInt + 183) = "Sand" then
Draw.FillBox (8 * 50, 7 * 50, 8 * 50 + 50, 7 * 50 + 50, 14)
elsif TileType (MapInt + 183) = "Water" then
Draw.FillBox (8 * 50, 7 * 50, 8 * 50 + 50, 7 * 50 + 50, 100)
end if
if TileType (MapInt + 184) = "Sand" then
Draw.FillBox (9 * 50, 7 * 50, 9 * 50 + 50, 7 * 50 + 50, 14)
elsif TileType (MapInt + 184) = "Water" then
Draw.FillBox (9 * 50, 7 * 50, 9 * 50 + 50, 7 * 50 + 50, 100)
end if
if TileType (MapInt + 200) = "Sand" then
Draw.FillBox (0 * 50, 8 * 50, 0 * 50 + 50, 8 * 50 + 50, 14)
elsif TileType (MapInt + 200) = "Water" then
Draw.FillBox (0 * 50, 8 * 50, 0 * 50 + 50, 8 * 50 + 50, 100)
end if
if TileType (MapInt + 201) = "Sand" then
Draw.FillBox (1 * 50, 8 * 50, 1 * 50 + 50, 8 * 50 + 50, 14)
elsif TileType (MapInt + 201) = "Water" then
Draw.FillBox (1 * 50, 8 * 50, 1 * 50 + 50, 8 * 50 + 50, 100)
end if
if TileType (MapInt + 202) = "Sand" then
Draw.FillBox (2 * 50, 8 * 50, 2 * 50 + 50, 8 * 50 + 50, 14)
elsif TileType (MapInt + 202) = "Water" then
Draw.FillBox (2 * 50, 8 * 50, 2 * 50 + 50, 8 * 50 + 50, 100)
end if
if TileType (MapInt + 203) = "Sand" then
Draw.FillBox (3 * 50, 8 * 50, 3 * 50 + 50, 8 * 50 + 50, 14)
elsif TileType (MapInt + 203) = "Water" then
Draw.FillBox (3 * 50, 8 * 50, 3 * 50 + 50, 8 * 50 + 50, 100)
end if
if TileType (MapInt + 204) = "Sand" then
Draw.FillBox (4 * 50, 8 * 50, 4 * 50 + 50, 8 * 50 + 50, 14)
elsif TileType (MapInt + 204) = "Water" then
Draw.FillBox (4 * 50, 8 * 50, 4 * 50 + 50, 8 * 50 + 50, 100)
end if
if TileType (MapInt + 205) = "Sand" then
Draw.FillBox (5 * 50, 8 * 50, 5 * 50 + 50, 8 * 50 + 50, 14)
elsif TileType (MapInt + 205) = "Water" then
Draw.FillBox (5 * 50, 8 * 50, 5 * 50 + 50, 8 * 50 + 50, 100)
end if
if TileType (MapInt + 206) = "Sand" then
Draw.FillBox (6 * 50, 8 * 50, 6 * 50 + 50, 8 * 50 + 50, 14)
elsif TileType (MapInt + 206) = "Water" then
Draw.FillBox (6 * 50, 8 * 50, 6 * 50 + 50, 8 * 50 + 50, 100)
end if
if TileType (MapInt + 207) = "Sand" then
Draw.FillBox (7 * 50, 8 * 50, 7 * 50 + 50, 8 * 50 + 50, 14)
elsif TileType (MapInt + 207) = "Water" then
Draw.FillBox (7 * 50, 8 * 50, 7 * 50 + 50, 8 * 50 + 50, 100)
end if
if TileType (MapInt + 208) = "Sand" then
Draw.FillBox (8 * 50, 8 * 50, 8 * 50 + 50, 8 * 50 + 50, 14)
elsif TileType (MapInt + 208) = "Water" then
Draw.FillBox (8 * 50, 8 * 50, 8 * 50 + 50, 8 * 50 + 50, 100)
end if
if TileType (MapInt + 209) = "Sand" then
Draw.FillBox (9 * 50, 8 * 50, 9 * 50 + 50, 8 * 50 + 50, 14)
elsif TileType (MapInt + 209) = "Water" then
Draw.FillBox (9 * 50, 8 * 50, 9 * 50 + 50, 8 * 50 + 50, 100)
end if
if TileType (MapInt + 225) = "Sand" then
Draw.FillBox (0 * 50, 9 * 50, 0 * 50 + 50, 9 * 50 + 50, 14)
elsif TileType (MapInt + 225) = "Water" then
Draw.FillBox (0 * 50, 9 * 50, 0 * 50 + 50, 9 * 50 + 50, 100)
end if
if TileType (MapInt + 226) = "Sand" then
Draw.FillBox (1 * 50, 9 * 50, 1 * 50 + 50, 9 * 50 + 50, 14)
elsif TileType (MapInt + 226) = "Water" then
Draw.FillBox (1 * 50, 9 * 50, 1 * 50 + 50, 9 * 50 + 50, 100)
end if
if TileType (MapInt + 227) = "Sand" then
Draw.FillBox (2 * 50, 9 * 50, 2 * 50 + 50, 9 * 50 + 50, 14)
elsif TileType (MapInt + 227) = "Water" then
Draw.FillBox (2 * 50, 9 * 50, 2 * 50 + 50, 9 * 50 + 50, 100)
end if
if TileType (MapInt + 228) = "Sand" then
Draw.FillBox (3 * 50, 9 * 50, 3 * 50 + 50, 9 * 50 + 50, 14)
elsif TileType (MapInt + 228) = "Water" then
Draw.FillBox (3 * 50, 9 * 50, 3 * 50 + 50, 9 * 50 + 50, 100)
end if
if TileType (MapInt + 229) = "Sand" then
Draw.FillBox (4 * 50, 9 * 50, 4 * 50 + 50, 9 * 50 + 50, 14)
elsif TileType (MapInt + 229) = "Water" then
Draw.FillBox (4 * 50, 9 * 50, 4 * 50 + 50, 9 * 50 + 50, 100)
end if
if TileType (MapInt + 230) = "Sand" then
Draw.FillBox (5 * 50, 9 * 50, 5 * 50 + 50, 9 * 50 + 50, 14)
elsif TileType (MapInt + 230) = "Water" then
Draw.FillBox (5 * 50, 9 * 50, 5 * 50 + 50, 9 * 50 + 50, 100)
end if
if TileType (MapInt + 231) = "Sand" then
Draw.FillBox (6 * 50, 9 * 50, 6 * 50 + 50, 9 * 50 + 50, 14)
elsif TileType (MapInt + 231) = "Water" then
Draw.FillBox (6 * 50, 9 * 50, 6 * 50 + 50, 9 * 50 + 50, 100)
end if
if TileType (MapInt + 232) = "Sand" then
Draw.FillBox (7 * 50, 9 * 50, 7 * 50 + 50, 9 * 50 + 50, 14)
elsif TileType (MapInt + 232) = "Water" then
Draw.FillBox (7 * 50, 9 * 50, 7 * 50 + 50, 9 * 50 + 50, 100)
end if
if TileType (MapInt + 233) = "Sand" then
Draw.FillBox (8 * 50, 9 * 50, 8 * 50 + 50, 9 * 50 + 50, 14)
elsif TileType (MapInt + 233) = "Water" then
Draw.FillBox (8 * 50, 9 * 50, 8 * 50 + 50, 9 * 50 + 50, 100)
end if
if TileType (MapInt + 234) = "Sand" then
Draw.FillBox (9 * 50, 9 * 50, 9 * 50 + 50, 9 * 50 + 50, 14)
elsif TileType (MapInt + 234) = "Water" then
Draw.FillBox (9 * 50, 9 * 50, 9 * 50 + 50, 9 * 50 + 50, 100)
end if
Draw.FillBox (CharX, CharY, CharX + 50, CharY + 50, red)
if MapIntX > 16 then
Draw.FillBox (CharX + 100, 0, maxx, maxy, 100 )
end if
end DrawWorld
%===========================================================================%
% = == = == = == = == = == = PlayerSpawn = == = == = == = == = == = == = %
%===========================================================================%
proc PlayerSpawn
PHealth := 100
CharX := SpawnX
CharY := SpawnY
end PlayerSpawn
%===========================================================================%
% = == = == = == = == = == = PlayerSpawn = == = == = == = == = == = == = %
%===========================================================================%
proc KeyDown
Input.KeyDown (chars )
if chars ('w') or chars (KEY_UP_ARROW) then
MapInt := MapInt + 25
MapIntY := MapIntY + 1
end if
if chars ('a') or chars (KEY_LEFT_ARROW) then
MapInt := MapInt - 1
MapIntX := MapIntX - 1
end if
if chars ('s') and MapIntY > - 4 or chars (KEY_DOWN_ARROW) then
MapInt := MapInt - 25
MapIntY := MapIntY - 1
end if
if chars ('d') and MapIntX < 18 or chars (KEY_RIGHT_ARROW) then
MapInt := MapInt + 1
MapIntX := MapIntX + 1
end if
end KeyDown
%===========================================================================%
% = == = == = == = == = == = Game Loop = == = == = == = == = == = == = %
%===========================================================================%
GenerateWorld
loop
GUI.SetBackgroundColour (100)
if PHealth < 100 then
PlayerSpawn
end if
KeyDown
DrawWorld
View.Update
delay (100)
cls
end loop
|
What in the mother of god is this crap you suck!
Shut up troll.
Wow thats messy.
I know, As I said this was my first "big" project and I wrote most of it on the fly. Its very inefficient. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Aange10

|
Posted: Tue Jan 03, 2012 11:14 pm Post subject: RE:Realm Of The Mad God / MiniCraft Terrain System [Incomplete] [Source] |
|
|
Learning functions or procedures could help you tremendously here. |
|
|
|
|
 |
Tony
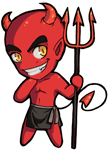
|
Posted: Tue Jan 03, 2012 11:29 pm Post subject: RE:Realm Of The Mad God / MiniCraft Terrain System [Incomplete] [Source] |
|
|
for-loops
code: |
if TileType (MapInt) = "Sand" then
Draw.FillBox (0 * 50, 0 * 50, 0 * 50 + 50, 0 * 50 + 50, 14)
elsif TileType (MapInt) = "Water" then
Draw.FillBox (0 * 50, 0 * 50, 0 * 50 + 50, 0 * 50 + 50, 100)
end if
if TileType (MapInt + 1) = "Sand" then
Draw.FillBox (1 * 50, 0 * 50, 1 * 50 + 50, 0 * 50 + 50, 14)
elsif TileType (MapInt + 1) = "Water" then
Draw.FillBox (1 * 50, 0 * 50, 1 * 50 + 50, 0 * 50 + 50, 100)
end if
... * forever
|
into
code: |
for counter : 0 .. whatever
if TileType (MapInt + counter) = "Sand" then
Draw.FillBox (counter * 50, 0 * 50, counter * 50 + 50, 0 * 50 + 50, 14)
elsif TileType (MapInt + counter) = "Water" then
Draw.FillBox (counter * 50, 0 * 50, counter * 50 + 50, 0 * 50 + 50, 100)
end if
end for
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Zren

|
Posted: Tue Jan 03, 2012 11:45 pm Post subject: RE:Realm Of The Mad God / MiniCraft Terrain System [Incomplete] [Source] |
|
|
Whenever you copy paste, it's a key indication you're doing something wrong (even if you haven't learned how to solve the problem, you should know the signs).
Basically this is a long winded explanation of what Tony just posted. Skip to the next point after the second tilde (~).
~
If you find two piece of code, back to back, consider it a sequence. How does one deal with sequences? Loops.
code: |
if blarg + fooey + 2 == meh then
doNargles()
end if
if blarg + fooey + 4 == meh then
doNargles()
end if
|
If I came upon the code above, I'd notice it's extremely similar. I'd look for differences. In it, there's just one thing different, the 2 and the 4. Essentially I'm running the same code twice, but with 2 different numbers. So:
code: |
for each item in the set [2, 4] as the variable x
if blarg + fooey + x == meh then
doNargles()
end if
|
There's no for each code structure in turing, so I'd have to make do with creating that sequence.
Turing: |
for x : 2 .. 4 by 2
put x
end for
|
Of course I'd use a more descriptive name than x (which brings me to my next point).
~
Magic Numbers
Also, do not, ever, hardcode in 'magic numbers'. You used the number 50 about a gazillion times, but I and anyone else who glances at your code have no idea for what reason you used it. No, don't explain it afterwards, that's not the point. The point is that your code should be readable. If you find you need to shift by a certain amount, you should do:
Draw.Dot(gameCanvasOffsetX + playerX, ...)
instead of:
Draw.Dot(30 + playerX, ...)
As you can see, in the former you can clearly understand what was happening because I used a variable with a descriptive name. Of course, I'd of set it's value earlier. If I didn't plan on changing that variable at runtime, I'd make it a constant. |
|
|
|
|
 |
pers2981
|
Posted: Wed Jan 04, 2012 1:01 am Post subject: RE:Realm Of The Mad God / MiniCraft Terrain System [Incomplete] [Source] |
|
|
Yeah, I already knew about the for loop / how to use it but for some reason ( I wasn't thinking straight ) I decided to use an endless number of if statements. If you see the code that decides what block is what it i used a forloop there, Also like I said its very inefficient and I wrote most of it at once. |
|
|
|
|
 |
pers2981
|
Posted: Wed Jan 04, 2012 1:16 am Post subject: RE:Realm Of The Mad God / MiniCraft Terrain System [Incomplete] [Source] |
|
|
Sorry for the double post.
Also noted that adding more "blocks" using my current method would be impossible and I attempted to accomplish the task of drawing only the bricks seen by the player by using a for loop but with the way the map is generated I found it impossible (for me at least)
This is how the map is. The black representing the area of map seen by the user/displayed. The red is the area generated but not being drawn.
Whilst generating each block is set with a variable. The map is 25 x 25
TileType (index)
So the first row of 25 blocks is water.
The next row (index 25- 50) will be randomly generated. There fore when i'm drawing the map the index has to jump x amount of numbers to make up for the rest of the row thats not being displayed.
Now I'm not asking for help as to fixing it just explaining my logic. |
|
|
|
|
 |
mirhagk
|
Posted: Wed Jan 04, 2012 12:35 pm Post subject: RE:Realm Of The Mad God / MiniCraft Terrain System [Incomplete] [Source] |
|
|
With a 2D version of minecraft you won't need to dynamically generate new parts of the map, because you only need to store a fraction of the information. 3D requires l*w*h whereas 2D only requires l*w. My own 2D version of minecraft stores tiles in a terribly inefficient way and it can still store 8000 x 8000 with no issues (which if you go 2 tiles per second, would take over an hour to cross the map) |
|
|
|
|
 |
pers2981
|
Posted: Wed Jan 04, 2012 1:30 pm Post subject: Re: RE:Realm Of The Mad God / MiniCraft Terrain System [Incomplete] [Source] |
|
|
mirhagk @ Wed Jan 04, 2012 12:35 pm wrote: With a 2D version of minecraft you won't need to dynamically generate new parts of the map, because you only need to store a fraction of the information. 3D requires l*w*h whereas 2D only requires l*w. My own 2D version of minecraft stores tiles in a terribly inefficient way and it can still store 8000 x 8000 with no issues (which if you go 2 tiles per second, would take over an hour to cross the map)
Okay? I'm aware the differences between 2d and 3d. I never said the engine couldn't store more then a 25x25 map? I just use such a small map due to the time consuming method I used to draw the map. Also I would love to see your engine/game in action. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Aange10

|
Posted: Wed Jan 04, 2012 5:31 pm Post subject: RE:Realm Of The Mad God / MiniCraft Terrain System [Incomplete] [Source] |
|
|
pers2981 wrote:
Okay? I'm aware the differences between 2d and 3d. I never said the engine couldn't store more then a 25x25 map?
Why are you so disrespectful to somebody trying to help you?
Read what he said:
Mirhagk wrote:
With a 2D version of minecraft you won't need to dynamically generate new parts of the map, because you only need to store a fraction of the information.
|
|
|
|
|
 |
pers2981
|
Posted: Wed Jan 04, 2012 8:56 pm Post subject: Re: RE:Realm Of The Mad God / MiniCraft Terrain System [Incomplete] [Source] |
|
|
Aange10 @ Wed Jan 04, 2012 5:31 pm wrote: pers2981 wrote:
Okay? I'm aware the differences between 2d and 3d. I never said the engine couldn't store more then a 25x25 map?
Why are you so disrespectful to somebody trying to help you?
Read what he said:
Mirhagk wrote:
With a 2D version of minecraft you won't need to dynamically generate new parts of the map, because you only need to store a fraction of the information.
I'm not being disrespectful and what he said helped me in no way.
Please stop posting in my threads, You aren't helping your just being annoying. |
|
|
|
|
 |
Tony
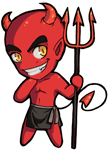
|
Posted: Wed Jan 04, 2012 9:30 pm Post subject: RE:Realm Of The Mad God / MiniCraft Terrain System [Incomplete] [Source] |
|
|
Right. mirhagk took things in a tangental direction. The bound is not in memory space, but in the design of implementation -- that was already acknowledged and discussed in this thread. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Aange10

|
Posted: Thu Jan 05, 2012 8:00 am Post subject: RE:Realm Of The Mad God / MiniCraft Terrain System [Incomplete] [Source] |
|
|
pers2981 wrote:
I'm not being disrespectful and what he said helped me in no way.
Emphassis:
mirhagk wrote:
you won't need to dynamically generate new parts of the map
And the rest of his post was his reasoning. Which you ironically followed up with
pers2981 wrote:
I just use such a small map due to the time consuming method I used
He was trying to help you. Weather it worked or not.
pers2981 wrote:
Please stop posting in my threads, You aren't helping your just being annoying.
It's a public thread. I'm sorry if you don't like my viewpoint on things, but I'm not going away. It's not like I'm trolling you; I'm trying to get you to see what is being said. |
|
|
|
|
 |
mirhagk
|
Posted: Thu Jan 05, 2012 12:09 pm Post subject: RE:Realm Of The Mad God / MiniCraft Terrain System [Incomplete] [Source] |
|
|
I was commenting on your saying generating new blocks would be impossible, saying you don't have to. |
|
|
|
|
 |
|
|