Remove Line from File
Author |
Message |
randint
|
Posted: Sun Nov 27, 2011 5:22 pm Post subject: Remove Line from File |
|
|
Does anyone know how to remove a specific line from a file?
The problem I came accross is:
code: |
import java.io.*;
public class deleteline
{
public static void main (String args[]) throws Exception
{
try
{
BufferedReader stdin = new BufferedReader (new InputStreamReader (System.in));
System.out.print ("Enter file name: ");
String file = stdin.readLine ();
File f = new File (file);
BufferedReader br = new BufferedReader (new InputStreamReader (new FileInputStream (f), "UTF-8"));
System.out.print ("Enter the line you want to delete: ");
String delete = stdin.readLine();
if (br.readLine ().equals (delete))
{
}
br.close ();
stdin.close ();
}
catch (Exception e)
{
System.exit (0);
}
}
}
|
What am I supposed to put into the if statement in order to delete the line?[/code] |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
ProgrammingFun

|
Posted: Sun Nov 27, 2011 5:43 pm Post subject: RE:Remove Line from File |
|
|
You could completely overwrite the file with that line missing by using arrays.
But I'm sure that there are better solutions. |
|
|
|
|
 |
Zren

|
Posted: Sun Nov 27, 2011 5:57 pm Post subject: RE:Remove Line from File |
|
|
This (your) code will get a string, checks the first line of the file, and compares it to your input.
~
As you're eventual goal is to change the size of the file, and you're not appending (adding on the end), you're required to rewrite the entire file. There are other more complicated ways to modify only certain parts of data, but let's not focus on that, as you'd need to shift all data after your found sections.
Simplified:
Read entire file.
Remove specified line(s) (could be done while reading).
Write to new file.
After you get it working. Consider:
If you think the file you are editing could be massive, consider writing to a temporary file as you read (and omit lines). Then overwrite the old filename with your temporary file. |
|
|
|
|
 |
randint
|
Posted: Sun Nov 27, 2011 9:08 pm Post subject: RE:Remove Line from File |
|
|
WHAT CODE DO YOU USE TO REMOVE THESE LINES??? I CANNOT FIND ANYTHING UNDER THE FILE CLASS OR THE STRING CLASS TO ALLOW THE LINE TO BE REMOVED! |
|
|
|
|
 |
Tony
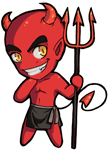
|
Posted: Sun Nov 27, 2011 9:33 pm Post subject: Re: RE:Remove Line from File |
|
|
http://bash.org/?835030 wrote:
<Khassaki> HI EVERYBODY!!!!!!!!!!
<Judge-Mental> try pressing the the Caps Lock key
<Khassaki> O THANKS!!! ITS SO MUCH EASIER TO WRITE NOW!!!!!!!
<Judge-Mental> fuck me
You've read the documentation -- there is no such function (nor would it make sense, for the reasons hinted at here in the discussion). Read the entire thread for suggested solutions. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Zren

|
Posted: Mon Nov 28, 2011 10:36 am Post subject: RE:Remove Line from File |
|
|
Some clearcut steps to learning to do this. Example code mentioned can easily be found with Google. From those examples, I leave you to learn how to combine them yourself, otherwise, you learn nothing.
Goal 1:
Print to console the entire file. This will require loops. An easy Googling of "java BufferedReader read from file" should come up with some example code. The right way to do it is with a while loop, as it will read until it fails to read (end of file). Common approach is:
String line;
while ((line = fin.readline()) != null) {}
Broken down:
(line = fin.readline()) will assign line to the next line. The operation will return the line variable after it's assignment. Then you're comparing it to make sure it's not null (eof).
Goal 2:
Compare and omit specific lines when printing to screen. This is easy as 'print line if it doesn't equal ____'.
Just in case you've forgotten, ! is the NOT operator.
Eg: !a.equals(b)
Goal 3:
Close your current project and focus on writing to a file. In a new project, learn how to create a new file and write something to it. Examples can easily be found with Googling 'java BufferedWriter write to file'. Don't forget to flush/close the stream, or nothing will write to file.
Goal 4:
Combine your knowledge so that a single program will load, omit, then write to file.
Conclusion:
You (with help) just broke down a larger task into smaller tasks. You also learned to search Google for how to do those menial tasks. |
|
|
|
|
 |
|
|