Author |
Message |
Tony
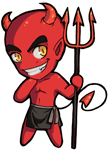
|
Posted: Wed Nov 27, 2002 1:11 am Post subject: [trig] so you're making a pool game? |
|
|
NOTE: thoughful has made a much better tutorial (well, mostly example) of a pool game found here I sujest checking it out.
Trigionometry and Physics are very important for games... expesially if there's some movement involved. In this tutorail I'll be talking about Physics on a pool table.
How To Make Realistic Pool Game
well Pool uses round balls so we need a oval collision detection to start us off... You may want to refer to tutorail about that... here's the code to remind us:
code: | distance = ((x2-x1)*(x2-x1) + (y2-y1)*(y2-y1))^0.5
if distance < radius1 + radius2 then
%We have a collision
end if |
lets also set up some variables:
code: | var x1, x2, y1, y2:int
var sp1x,sp1y, sp2x,sp2y:int
var distance:real |
x1,y1 are position of first ball and x2,y2 are position of second. They are used for drawing the images and calculating distances.
sp1x, sp1y are movement of the ball along X and Y axis. Same for sp2's.
Now for some declarations:
code: | x1:=100
x2:=500
y1:=100
y2:=105
sp1x:=5
sp1y:=0
sp2x:=0
sp2y:=0 |
here you actually put your start set up of the table. This is just for an example, with 2 balls, 1 set to hit the other.
and lets add the actual loop now...
code: | loop
%movement of balls
x1 += sp1x
x2 += sp2x
y1 += sp1y
y2 += sp2y
%calculating distance between
distance := ((x2-x1)*(x2-x1) + (y2-y1)*(y2-y1))** 0.5
if distance < 10 %if collision
then
sp2x:=sp1x %ball 2 gets 100% transfer of enery from ball 1
sp2y:=sp1y
sp1x:=0 %first ball instantly stops, though in fact it should hit back with
sp1y:=0 %atleast some energy... but we live in a perfect world :wink:
end if
drawoval(x1,y1,5,5,red) %draw balls
drawoval(x2,y2,5,5,blue)
delay(100)
end loop |
Put everything together and we'll have a little animation... But it doesn't look right... why? Because the second ball should have went @ 45degree angle, shouldn't it have? Yeah... we have a problem...
I'll continue this tutorial later on... I'm gonna go sleep now. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
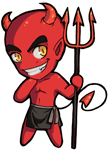
|
Posted: Fri Nov 29, 2002 9:49 pm Post subject: (No subject) |
|
|
Making a pool game - Part 2
Here's a little diagram for us:
code: |
/```\
\_`_/
/```\
\_`_/ |
Two balls colliding... If we draw the line connecting two centers, we'll get the direction of path for 2nd ball. Magnitude will be the speed of moving ball (@ perfect energy transfer). The X and Y components of this vector will make up relative velocity at which 2nd ball should start moving... Here's another diagram:
code: |
. <center of 2nd ball
centr/ |
b1 / |
\/ / | Y-component (y2-y1)
/ |
.______|
X-component
(x2-x1) |
Velocity of 1st ball is ball1V = (sp1x*sp1x + sp1y*sp1y)**0.5
It should be evenly destributed between X and Y components of ball2, so:
code: |
sp2x := round(sp1x*sp1x + sp1y*sp1y)**0.5 * ((x2-x1)/(x2-x1 + y2-y1))
sp2y := round(sp1x*sp1x + sp1y*sp1y)**0.5 * ((y2-y1)/(x2-x1 + y2-y1))
|
A bit of explanation here first part (sp1x*sp1x + sp1y*sp1y)**0.5 thats the total speed of first ball... we already covered that.
((x2-x1)/(x2-x1 + y2-y1)) finds the % makeup of X-axis movement of total speed.
same for Y-axis, just change letters.
Now put that sp2's = code inside the loop instead of previous sp2's =. Your program should work fine.
Try changing y2 to different values between 99 and 109 for different results.
NOTE: The program is buggy. Since I initially used INT variables instead of REAL as I should have, I was forced to use ROUND() which produces inacurate results if the angle of hit is <45 and not=0.
Also you'll run into problem if your 2nd ball is below 1st. You should use Absolute values |x| instead while keeping track of relative ball locations.
I hope this is enough to start you pool game off  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
JayLo
|
Posted: Sat Nov 30, 2002 2:39 am Post subject: thanks for the help. |
|
|
couldn't there be a code like this for collision??
var bounce : real
bounce := sqrt ((x2 - x) ** 2 + (y2 - y) ** 2)
if bounce <= 14 then
xChange := -xChange
xChange2 := xChange
yChange := -yChange
yChange2 := yChange
end if[/quote] |
|
|
|
|
 |
Tony
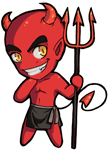
|
Posted: Sat Nov 30, 2002 3:41 pm Post subject: (No subject) |
|
|
no... because ball1 will fly at same speed in opposite direction and ball 2 will go in direction of ball1 at its speed... which is incorect both in direction and speed for both of balls.
Just read the tutorial, code works fine. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Cervantes

|
Posted: Sat Jan 17, 2004 4:38 pm Post subject: (No subject) |
|
|
works nicely.. I like your approach more that Homer's approach from slime volleyball, yours is easier to understand, though more buggy
the main problem i have with yours is if you set y2 to 109 then the angle that the first ball bounces off is right but the ball that was moving in the first place (ball2) really shouldn't stand still all of a sudden... is there anyway to fix that? other than doing Homer's way with angles and weights and velocitys and all.
the other problem is if you change the size of the ovals then it doesn't bounce right at all
is there any way to change that as well?
Cheers |
|
|
|
|
 |
Tony
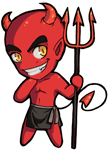
|
Posted: Sat Jan 17, 2004 4:51 pm Post subject: (No subject) |
|
|
I think so
the radious values are hardcoded, so that is probably the cause of the error...
ether way, you should keep in mind that I wrote this long ago and you should not rely on the code since it doesnt work  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Cervantes

|
Posted: Sat Jan 17, 2004 6:32 pm Post subject: (No subject) |
|
|
is there a way to make it deliver the correct amount of energy (not 100%) and then make the first ball bounce off correctly instead of just stopping? |
|
|
|
|
 |
Tony
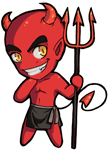
|
Posted: Sat Jan 17, 2004 7:05 pm Post subject: (No subject) |
|
|
yes...
code: |
sp2x:=sp1x %ball 2 gets 100% transfer of enery from ball 1
sp2y:=sp1y
sp1x:=0 %first ball instantly stops, though in fact it should hit back with
sp1y:=0 %atleast some energy... but we live in a perfect world :wink:
|
so instead you redestribute it based on the weight... Since both balls are the same, each should get 50%, so:
code: |
sp2x:=sp1x*0.5
sp2y:=sp1y*0.5
sp1x:=sp1x*-0.5
sp1y:=sp1y*-0.5
|
first ball is multiplied by -50% to reverce it's direction... I think  |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
Cervantes

|
Posted: Sat Jan 17, 2004 7:19 pm Post subject: (No subject) |
|
|
im sorry I don't quite follow..
where do you insert this part? (below)
code: | sp2x:=sp1x*0.5
sp2y:=sp1y*0.5
sp1x:=sp1x*-0.5
sp1y:=sp1y*-0.5 |
tried some things none work so far |
|
|
|
|
 |
Cervantes

|
Posted: Sat Jan 17, 2004 7:22 pm Post subject: (No subject) |
|
|
ooh I just tried something else... nothing to do with energy transfer or anything but I figured out how to make it work right even when the sizes are different..
just change the if distance < ## part to ball1 radius + ball2 radius
hurray!  |
|
|
|
|
 |
Tony
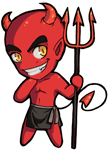
|
Posted: Sat Jan 17, 2004 8:29 pm Post subject: (No subject) |
|
|
you insert that part in place of other block mentioned in first half of the post |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
santabruzer

|
Posted: Thu Jan 22, 2004 3:11 pm Post subject: (No subject) |
|
|
where does the 0.5 come from.. i just don't get that part  |
|
|
|
|
 |
Cervantes

|
Posted: Thu Jan 22, 2004 5:55 pm Post subject: (No subject) |
|
|
the .5 is saying that each of them are getting .5 (aka 50%) of the energy from the collision. |
|
|
|
|
 |
santabruzer

|
Posted: Thu Jan 22, 2004 6:22 pm Post subject: (No subject) |
|
|
so in reality changing that or redeclaring a variable for that will alow to change the amount of energy it recieves.. excellent.. |
|
|
|
|
 |
|