Help with do .... while loop Conditon error
Author |
Message |
brl1995
|
Posted: Fri Oct 07, 2011 1:12 pm Post subject: Help with do .... while loop Conditon error |
|
|
alright, so im trying to create a validation loop that will exit when the user enters either "y" or "n" so to code im using is
do
{
System.out.print ("Are you a member?(y/n): ");
member = stdin.readLine ();
}
while (member != ("y") || ("n"));
ind im getting the error
the tytpe of this expression, "Java.lang.String", is not "boolean"
can someone explain to be how to fix it thanks!
** Edit ** by the way this is using ready to program btw
i will post the rest of the code below if that helps (bare in mind it is unfinished and will most likely have other errors)
import java.io.*;
import java.util.*;
import java.text.*;
public class Review4a
{
public static void main (String str[]) throws IOException
{
BufferedReader stdin = new BufferedReader (new InputStreamReader (System.in));
String member, response;
int numdisks, price, cost;
price = 9001;
do
{
System.out.println ();
System.out.print ("Enter the number of disks you wish to purchase: ");
numdisks = Integer.parseInt (stdin.readLine ());
do
{
System.out.print ("Are you a member?(y/n): ");
member = stdin.readLine ();
}
while (member != ("y")|| ("n"));
if (member.equalsIgnoreCase ("y"))
{
price = 4;
}
else if (member.equalsIgnoreCase ("n"))
{
price = 6;
}
else
{
System.out.println ("Error Invalid Input");
}
cost = numdisks * price;
System.out.println ();
System.out.println ("The cost of each disk is $" + price);
System.out.println ("Your total is $" + cost);
System.out.println ();
System.out.println ("Would you like to try again?(y/n): ");
response = stdin.readLine ();
}
while (response.equalsIgnoreCase ("y"))
;
}
} |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
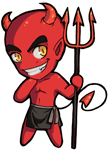
|
Posted: Fri Oct 07, 2011 1:38 pm Post subject: RE:Help with do .... while loop Conditon error |
|
|
in cases where you have
code: |
while ( condition )
|
the "condition" part needs to evaluate to a boolean value (true/false).
you can put together more complex expressions such as
code: |
while ( condition && condition )
while ( condition || condition )
|
and the boolean requirements recursively applies to every part.
In your case, the condition of
code: |
while ( ... || ("n") )
|
evaluates to a string, not a boolean value. So you get an error that says exactly this
Quote:
the type of this expression, "Java.lang.String", is not "boolean"
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
brl1995
|
Posted: Fri Oct 07, 2011 1:50 pm Post subject: Re: Help with do .... while loop Conditon error |
|
|
Thank you so much, i was being stupid lol i forgot that what i was saying in:
while (member != ("y") || ("n"));
was saying: while member does not equal "y" and = "n"
what i wanted it to say is:
while (member != ("y") || member != ("n"))
silly bad habits i learned last year with turing lol
anyway, thanks for the help
i just had one more question is it possible to make the condition ignore case? ex in the statment if (member.equalsIgnoreCase ("y"))
i know i could just further expand the conditions using
while (member != ("y") || member != ("n") || member != ("Y") || member != ("N"))
but was hoping there would be a more condensed way of saying this
i want to do this in the statment we just fixed if possible
thanks again |
|
|
|
|
 |
Tony
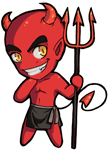
|
Posted: Fri Oct 07, 2011 2:30 pm Post subject: RE:Help with do .... while loop Conditon error |
|
|
you could change the case once before the evaluation.
Also, there are still two more problems with your while statement
1 - what must be the value of "member" for the loop to actually exit?
2 - explain the difference between == and .equals |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
brl1995
|
Posted: Sat Oct 08, 2011 12:14 pm Post subject: Re: Help with do .... while loop Conditon error |
|
|
while (member != ("y") || member != ("n"))
what that basicly says is while member does not equal to y or n then it loops if member = y or n then it will exit
the only differece is that with .equals i can add the ignore case function where as with = i have to add more conditions |
|
|
|
|
 |
Nick

|
Posted: Sat Oct 08, 2011 2:10 pm Post subject: RE:Help with do .... while loop Conditon error |
|
|
Java is stupid with strings, they're not a value type, they're a reference type. So we have say foo = "Foo" and bar = "Foo", if we try to evaluate this we'd get bar == foo which would return a false.. why? Because bar does not equal 'Foo", it equals a pointer to a memory location in which "foo" is stored. However, bar.Equals (foo) would return true as it takes this into account and checks against the string rather than the memory location. |
|
|
|
|
 |
Tony
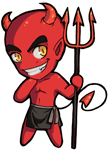
|
Posted: Sat Oct 08, 2011 2:26 pm Post subject: RE:Help with do .... while loop Conditon error |
|
|
Both answers are incorrect.
1 - consider member == "n". Will the loop continue? I.e. will member != ("y") equate to true?
2 - no.
http://download.oracle.com/javase/6/docs/api/java/lang/String.html#equals(java.lang.Object) wrote:
Compares this string to the specified object. The result is true if and only if the argument is not null and is a String object that represents the same sequence of characters as this object.
http://psc.informatik.uni-jena.de/languages/Java/javaspec-3.pdf (JLS v3) wrote:
At run time, the result of == is true if the operand values are both null or
both refer to the same object or array; otherwise, the result is false.
...
While == may be to compare references of type String, such an equality test determines whether or not the two operands refer to the same String
object. The result is false if the operands are distinct String objects, even if
they contain the same sequence of characters
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Tony
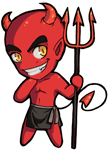
|
Posted: Sat Oct 08, 2011 2:29 pm Post subject: Re: RE:Help with do .... while loop Conditon error |
|
|
Nick @ Sat Oct 08, 2011 2:10 pm wrote: So we have say foo = "Foo" and bar = "Foo", if we try to evaluate this we'd get bar == foo which would return a false.. why?
Even more hilarious, if the compiler figures out enough to optimize "Foo" and "Foo" into the same location, then == will return true. Other times it will return false. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
|
|