Author |
Message |
lufthansa747
|
Posted: Sun Sep 11, 2011 10:43 pm Post subject: Help with Java Semiphore |
|
|
ok what i am trying to do with the semiphore is. i want to init it and for every thread i make i want to increase the count by 1. when the thread is done i want to decrease the count by 1. while all this is happening i want the main thread to wait untill the semphore has reached and count of 0 and then continue with the code. how can i do this. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
lufthansa747
|
Posted: Mon Sep 12, 2011 7:39 am Post subject: Re: Help with Java Semiphore |
|
|
bump |
|
|
|
|
 |
S_Grimm

|
Posted: Mon Sep 12, 2011 8:29 am Post subject: RE:Help with Java Semiphore |
|
|
http://www.javaworld.com/javaworld/jw-04-1996/jw-04-threads.html?page=2
try using that code but with a counter instead of the boolean variable.
eg code: | if (counter > 1)
{
threadOne.suspend();
}
else
{
threadOne.resume();
}
|
and have your other threads increment the counter when they are called.
edit: you'd have to stick it in a block of code that isn't mouseDown() |
|
|
|
|
 |
Tony
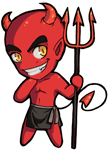
|
Posted: Mon Sep 12, 2011 8:35 am Post subject: RE:Help with Java Semiphore |
|
|
A semaphore is typically used to block until a resource becomes available (continue with code when a count is above 0), but I suppose you can inverse the condition.
The only tricky part to the implementation are atomic increment/decrement operations, but then there's java.util.concurrent.atomic |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
lufthansa747
|
Posted: Mon Sep 12, 2011 2:00 pm Post subject: Re: RE:Help with Java Semiphore |
|
|
Tony @ Mon Sep 12, 2011 8:35 am wrote: A semaphore is typically used to block until a resource becomes available (continue with code when a count is above 0), but I suppose you can inverse the condition.
The only tricky part to the implementation are atomic increment/decrement operations, but then there's java.util.concurrent.atomic
well this is why i want to do that. i have one main thread. that thread spawn off 9 other threads which all do there own math operations. when they are done they update the main thread with numbers and decrement a variable in the main thread which keeps track of alive threads. currently i have my main thread stuck in a while loop with a 1 millisecond sleep waiting for the other threads to finish. i was hopping that using a semphore could optimize the code a bit because it would be more efficient then calling thread.sleep? |
|
|
|
|
 |
Tony
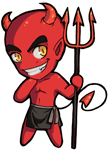
|
Posted: Mon Sep 12, 2011 2:06 pm Post subject: RE:Help with Java Semiphore |
|
|
With a semaphore, you would still be busy-waiting. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
lufthansa747
|
Posted: Mon Sep 12, 2011 2:42 pm Post subject: Re: RE:Help with Java Semiphore |
|
|
Tony @ Mon Sep 12, 2011 2:06 pm wrote: With a semaphore, you would still be busy-waiting.
but wouldn't it be faster and more efficient |
|
|
|
|
 |
Tony
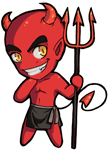
|
Posted: Mon Sep 12, 2011 2:59 pm Post subject: RE:Help with Java Semiphore |
|
|
Than what? How are you checking that other threads have finished now? How is that different from what you plan on doing? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Sponsor Sponsor

|
|
 |
lufthansa747
|
Posted: Mon Sep 12, 2011 5:58 pm Post subject: Re: RE:Help with Java Semiphore |
|
|
Tony @ Mon Sep 12, 2011 2:59 pm wrote: Than what? How are you checking that other threads have finished now? How is that different from what you plan on doing?
the code currently looks like this
while(threadCount != 0){
Thread.Sleep(1);
}
//do something her
but i thought that if i could use a semiphore block it would be better because i would not be doing a tight loop. lol idk i just though it would be better. |
|
|
|
|
 |
DemonWasp
|
Posted: Mon Sep 12, 2011 8:47 pm Post subject: RE:Help with Java Semiphore |
|
|
In Java, if you want to wait for another thread to complete, use .join() on the thread you want to wait for. See: http://download.oracle.com/javase/6/docs/api/java/lang/Thread.html
Your main then looks like:
Java: |
List<Thread> threads = ... // your code here...
// Start all threads...
for ( Thread thread : threads ) {
thread.start();
}
// Wait until all threads are done...
for ( Thread thread : threads ) {
thread.join();
}
|
Join puts the calling thread to sleep until the target thread is done. This is done without a busy-wait. |
|
|
|
|
 |
|