Author |
Message |
morningpost
|
Posted: Tue Jan 25, 2011 9:05 pm Post subject: Java File Input (Character by Character) |
|
|
Hi there,
I just need to know how to read in from a text file character by character in Java.
I've tried using Scanner, InputStreamReader, DataInputStream, FileInputStream ... but I'm obviously going wrong somewhere. I just can't seem to be able to read in from the file character by character. The file is supposed to be a maze, with the first two numbers representing # of columns and # of rows. I want to read in character by character so I can place the characters into a 2D array in my program.
The textfile looks something like this (legend: '#' - wall, '.' - open space, 'o' - start, '*' - finish):
7 4
#######
# . . . #o#
# *#. . . #
#######
^ So, these are the types of characters I want to read in one by one
If someone could help with this, that'd be great! Thanks!  |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
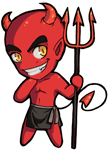
|
|
|
|
 |
morningpost
|
Posted: Tue Jan 25, 2011 9:27 pm Post subject: Re: RE:Java File Input (Character by Character) |
|
|
Tony @ Tue Jan 25, 2011 9:19 pm wrote:
I tried using the Scanner this way, but it won't read in character by character ...
File file = new File("maze1.txt");
try {
s = new Scanner(file);
int columns = s.nextInt();
int rows = s.nextInt();
String grid = new String[rows][columns];
while (s.hasNext()) {
int r = 0;
int col = 0;
String square = s.next();
grid[r][col] = square;
r++;
col++;
}
}catch (FileNotFoundException e) {
System.out.println("File cannot be found! " + e);
} |
|
|
|
|
 |
Tony
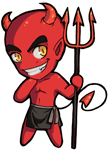
|
|
|
|
 |
RandomLetters
|
Posted: Tue Jan 25, 2011 9:43 pm Post subject: RE:Java File Input (Character by Character) |
|
|
You can use toCharArray() method to convert string to an array of characters. |
|
|
|
|
 |
morningpost
|
Posted: Tue Jan 25, 2011 9:49 pm Post subject: Re: RE:Java File Input (Character by Character) |
|
|
Tony @ Tue Jan 25, 2011 9:40 pm wrote: What does #next() return..?
http://download.oracle.com/javase/6/docs/api/java/util/Scanner.html#next()
code: |
String grid = new String[rows][columns];
|
Wait, what does this mean?
next () returns the entire first row and then nothing else :\
oh, sorry. It's suppose to be String [][] grid = new String[rows][columns]. My mistake. |
|
|
|
|
 |
Tony
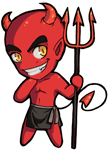
|
Posted: Tue Jan 25, 2011 9:53 pm Post subject: Re: RE:Java File Input (Character by Character) |
|
|
morningpost @ Tue Jan 25, 2011 9:49 pm wrote: next () returns the entire first row and then nothing else :\
Yup, sounds like that's not the method that you are looking for. Find one that returns the type that you want. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
ProgrammingFun

|
Posted: Tue Jan 25, 2011 10:21 pm Post subject: RE:Java File Input (Character by Character) |
|
|
Why don't you use BufferedReader to read one line at a time. This line can be saved in a String from which you can use .charAt() to extract characters? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
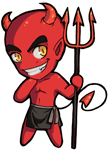
|
Posted: Tue Jan 25, 2011 10:38 pm Post subject: RE:Java File Input (Character by Character) |
|
|
Or use a Scanner to scan for the next character token and use that directly. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
RandomLetters
|
Posted: Tue Jan 25, 2011 11:24 pm Post subject: Re: RE:Java File Input (Character by Character) |
|
|
Tony @ Tue Jan 25, 2011 10:38 pm wrote: Or use a Scanner to scan for the next character token and use that directly.
How do you do that? next() Set delimiter to ""? I could not find a nextChar() method or similar, which is interesting (so there must be an obvious way) |
|
|
|
|
 |
|