mandelbrot set in java
Author |
Message |
hamid14

|
Posted: Sat Jan 08, 2011 1:16 pm Post subject: mandelbrot set in java |
|
|
im trying to draw the mandelbrot set in netbeans using java, but i cant get it work properly. if i use g.drawOval (x,y,1,1); it says you cannot apply doubles to int, which i understand why. but then if i convert the x and y to int, then the whole screen just goes black. I dont understand what i should do at this point.
heres my code:
import java.awt.*;
import BreezyGUI.*;
public class BreezyStuff extends GBFrame {
public void paint(Graphics g) {
double imageHeight = 399;
double imageWidth = 639;
double minRe = -2;
double maxRe = 1;
double minIm = -1.2;
double maxIm = minIm + (maxRe - minRe) * imageHeight / imageWidth;
double Re_factor = (maxRe - minRe) / (imageWidth - 1);
double Im_factor = (maxIm - minIm) / (imageHeight - 1);
double unsignedMaxIterations = 30;
for (double y = 0; y < imageHeight; y++) {
double c_im = maxIm - y * Im_factor;
for (double x = 0; x < imageWidth; x++) {
double c_re = minRe + x * Re_factor;
double Z_re = c_re;
double Z_im = c_im;
boolean isInside = true;
for (double n = 0; n < unsignedMaxIterations; n++) {
double Z_re2 = Z_re * Z_re;
double Z_im2 = Z_im * Z_im;
if (Z_re2 + Z_im2 > 4) {
isInside = false;
}
Z_im = 2 * Z_re * Z_im + c_im;
Z_re = Z_re2 - Z_im2 + c_re;
}
if (isInside = true) {
int e, f;
e = (int) x;
f = (int) y;
g.setColor(Color.BLACK);
g.drawOval(e, f, 1, 1);
}
}
}
}
public static void main(String[] args) {
Frame frame = new BreezyStuff();
frame.setSize(639, 399);
frame.setVisible(true);
}
}
im using breezygui because my teacher wanted us to ""implement"" into our code somehow >.> any help is greatly appreciated |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
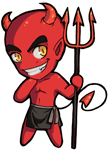
|
Posted: Sat Jan 08, 2011 1:26 pm Post subject: RE:mandelbrot set in java |
|
|
so presumably to color the entire screen black, "isInside" must always be true. Is this correct for the location you are trying to examine? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
hamid14

|
Posted: Sat Jan 08, 2011 2:06 pm Post subject: Re: mandelbrot set in java |
|
|
when isInside = true then its supposed to draw the black dots on the screen, assuming the background is white. i moved the defining of the variable isInside to the outside of the for loops but still doesnt work. the screen goes black from top to bottom 4-5 times and then stops. |
|
|
|
|
 |
2goto1

|
Posted: Sat Jan 08, 2011 4:58 pm Post subject: RE:mandelbrot set in java |
|
|
in your code if (isInside = true), did you intend to compare isInside with the boolean value true, rather than assign it? If so, you should use the == operator, so it becomes if (isInside == true). Otherwise the expression as-is assigns true to isInside, and evaluates as true. |
|
|
|
|
 |
Tony
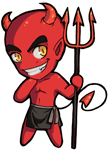
|
Posted: Sat Jan 08, 2011 5:25 pm Post subject: RE:mandelbrot set in java |
|
|
hah, I've missed that too D:
Really, the expression should have been
code: |
if (isInside) { ...
|
|
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
2goto1

|
Posted: Sat Jan 08, 2011 5:40 pm Post subject: Re: RE:mandelbrot set in java |
|
|
Tony @ Sat Jan 08, 2011 5:25 pm wrote: hah, I've missed that too D:
Really, the expression should have been
code: |
if (isInside) { ...
|
Yes that is much cleaner, and an allowed syntax in most common languages. Less is best! |
|
|
|
|
 |
hamid14

|
Posted: Sat Jan 08, 2011 6:42 pm Post subject: Re: mandelbrot set in java |
|
|
now it doesnt do anything at all i dont know what wrong with it :\
EDIT: i got it working thanks guys |
|
|
|
|
 |
hamid14

|
Posted: Sat Jan 08, 2011 7:28 pm Post subject: Re: mandelbrot set in java |
|
|
sorry for double posting, but how would i create different colors? couldnt find any tutorials on how to use color functions in java netbeans |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
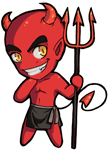
|
Posted: Sat Jan 08, 2011 7:39 pm Post subject: RE:mandelbrot set in java |
|
|
I would assume that Color is some kind of a class and has some methods and/or constants (such as #BLACK) that give you "colours". Read the docs. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
hamid14

|
Posted: Sat Jan 08, 2011 8:52 pm Post subject: Re: mandelbrot set in java |
|
|
no i meant coloring the different spaces, not the black space itself thanks anyways |
|
|
|
|
 |
|
|