Logo
Author |
Message |
MysticAngel

|
Posted: Wed Mar 26, 2003 10:08 pm Post subject: Logo |
|
|
This is a mini verson of the Logo program. basically you move a turtle around the computer with a couple of commands. unfortuanely my turtle is a dot. neways its fun. enjoy
code: |
%Author:MysticAngel
%Date:March 2,2003
%Purpose:The program will move a "turtle" around on the screen in
%response to four commands.
%Input:The user will input a set of commands to the turtle
%Output:the movement of the turtle
var iWindow, iWindow1 : int
var sDirection : string
var iStep, x, y, x2, y2, iDir : int := 0
var sMove : string (1)
iWindow := Window.Open ("grahics:500;500")
colorback(black)
cls
color(brightgreen)
iWindow1 := Window.Open ("graphics:500;500")
colorback(black)
cls
color(brightgreen)
x := 150
y := 150
iDir := 90
Window.Select (iWindow)
Draw.Dot (x, y,brightgreen)
loop
Window.Select (iWindow1)
if iDir = - 90 then
iDir := 270
end if
if iDir = 360 then
iDir := 0
end if
cls
if iDir = 0 then
sDirection := "e"
put ""
put "The turtle is facing the East direction"
elsif iDir = 180 or iDir = - 180 then
sDirection := "w"
put ""
put "The turtle is facing the West direction"
elsif iDir = 90 then
sDirection := "n"
put ""
put "The turtle is facing the North direction"
else
sDirection := "s"
put ""
put "The turtle is facing the South direction"
end if
Window.Select (iWindow1)
put""
put "To move Left(L), move Right(R), move Forward(F), and to Quit(Q)"
put "What movement do you want the turtle to do?"
getch (sMove)
if sMove = "R" or sMove = "r" then
iDir := iDir - 90
elsif sMove = "l" or sMove = "L" then
iDir := iDir + 90
elsif sMove = "f" or sMove = "F" then
Window.Select (iWindow1)
put""
put "How many step do you want the turtle to move"
get iStep
Window.Select (iWindow)
if sDirection = "n" then
x2 := x
y2 := y + iStep
Draw.Line (x, y, x2, y2, brightgreen)
x := x2
y := y2
elsif sDirection = "s" then
x2 := x
y2 := y - iStep
Draw.Line (x, y, x2, y2, brightgreen)
x := x2
y := y2
elsif sDirection = "e" then
y2 := y
x2 := x + iStep
Draw.Line (x, y, x2, y2, brightgreen)
x := x2
y := y2
else
y2 := y
x2 := x - iStep
Draw.Line (x, y, x2, y2, brightgreen)
x := x2
y := y2
end if
end if
exit when sMove ="q" or sMove = "Q"
end loop
Window.Close(iWindow)
Window.Close(iWindow1)
|
MOD Edit: Thx for sharing your program with us. Giving you +7Bits - Tony |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Prince
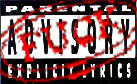
|
Posted: Thu Mar 27, 2003 10:54 am Post subject: more logo |
|
|
heres another version of the logo program... same idea but wit procedures and stuff
code: |
% Name: PrInCe_
% Date: Mar. 26, 2003
% Purpose: Draws a "turtle" and moves it around the
% screen based on the users commands
% Inputs: Direction (direction), Distance (distance), User Command
% (command)
% Output: Turtle's movements shown on the "turtle window"
% fuction getCommand
% purpose: Gets the command from the user
% parameters: key
% return value: key
function getCommand : string (1)
var key : string (1)
put "What would you like to do? " ..
getch (key)
result key
end getCommand
% procedure goLeft
% purpose: changes the direction of the turtle to make it go left
% input parameters: none
% output parameters: none
procedure goLeft (var direction : int)
delay (500)
direction := direction + 90
if direction >= 360 then
direction := 0
end if
end goLeft
% procedure goRight
% purpose: changes the direction of the turtle to make it go right
% input parameters: none
% output parameters: none
procedure goRight (var direction : int)
delay (500)
direction := direction - 90
if direction = - 90 then
direction := 270
end if
end goRight
% procedure goForward
% purpose: moves the turtle forward depending on the direction being faced
% and the distance determined by the user
% input parameters: distance
% output parameters: none
procedure goForward (var x, y, win1, direction : int)
var distance : int
loop
locate (7, 1)
put "How far would you like to go ? " ..
get distance
exit when distance >= 0
locate (7, 31)
put " "
end loop
Window.Select (win1)
if direction = 270 then
Draw.Line (x, y, x + distance, y, black)
x := x + distance
elsif direction = 90 then
Draw.Line (x, y, x - distance, y, black)
x := x - distance
elsif direction = 180 then
Draw.Line (x, y, x, y - distance, black)
y := y - distance
else
Draw.Line (x, y, x, y + distance, black)
y := y + distance
end if
end goForward
% procedure quitProgram
% purpose: closes the program when the user decides to quit
% input parameters: none
% output parameters: none
procedure quitProgram (var win1, win2 : int)
Window.Close (win1)
Window.Select (win2)
Window.Close (win2)
Window.Select (0)
end quitProgram
% ---------- Main ----------
var direction : int := 0
var x, y : int
var command : string (1)
var win1, win2 : int
% turtle window
win1 :=
Window.Open ("graphics:1013;550,position:0;0,,noecho,nocursor,title:Turtle Window")
% command window
win2 := Window.Open ("graphics:1013;155,position:0;590,title:Command Window")
colorback (53)
cls
Window.Select (win2)
x := 506
y := 275
Window.Select (win1)
colorback (brightgreen)
cls
loop
Window.Select (win2)
cls
put "This turtle obeys only 4 commands"
put "f - go forward"
put "r - turn right"
put "l - turn left"
put "q - quit"
command := getCommand
if command = "l" or command = "L" then
goLeft (direction)
elsif command = "r" or command = "R" then
goRight (direction)
elsif command = "f" or command = "F" then
goForward (x, y, win1, direction)
elsif command = "q" or command = "Q" then
quitProgram (win1, win2)
end if
exit when command = "q" or command = "Q"
end loop
|
MOD Edit: Thx for sharing your program I'm giving you +8Bits - Tony |
|
|
|
|
 |
|
|