Java
Author |
Message |
magic

|
Posted: Sat Dec 25, 2010 6:02 am Post subject: Java |
|
|
please help me with this problem..
It's my first time to learn about Java..
Write a program that reads in a whole bunch of words (each word is no more than 20 characters) and spit out the duplicate words. For our purposes, words are to be separated by spaces, commas (,), !, ?, and periods (.). No word goes past the end of a line. All comparisons should ignore case.
Output: Output the sorted words, one per line, in lower case. Display same words only once.
Sample Input:
How much wood would a woodchuck chuck if a woodchuck could chuck wood? Well? What
do you think? Are you feeling well?
Sample Console:
a
are
chuck
could
do
feeling
how
if
much
think
well
what
wood
woodchuck
would
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
ProgrammingFun

|
Posted: Sat Dec 25, 2010 9:31 am Post subject: RE:Java |
|
|
Which part do you need help with? We cannot give you the complete code but can help you with concepts, debugging etc... |
|
|
|
|
 |
magic

|
Posted: Tue Dec 28, 2010 9:27 am Post subject: RE:Java |
|
|
thank you very much..
I'm just confused on how can I spit out the words and display the same words only once? |
|
|
|
|
 |
ProgrammingFun

|
Posted: Tue Dec 28, 2010 11:33 am Post subject: Re: Java |
|
|
You can use the split method to split all of the words into an array:
Java: |
String test = "This is my string."; //This is the string you are using
String[] splitstring; //Declare an array to store each word
splitstring = test. split (" "); //Feed the split words into the array
/*This will split the words whenever a space " " is found...you can replace the " " with any character (such as ",").
To use this method, you will need to import java.util.* or java.io.*
This method is initialized by StringName.split ("character");
You can then access word in the array by using splitstring[arrayindex] */
|
After this, you can use a declare a new array which is the size of the array containing the words.
Then initialize a for loop which reads thru the current array, stores the word being processed, and then if it is being repeated and is not already been stored in the second array, you should store it in the second array.
I hope that makes some sense...there might be more efficient ways to do this but this is what came to mind first.  |
|
|
|
|
 |
jcollins1991
|
Posted: Tue Dec 28, 2010 12:44 pm Post subject: Re: Java |
|
|
edit: Actually, since it's your first time learning Java you should probably first read up on stuff like for loops and strings. After you understand those read up on a few sorting algorithms http://en.wikipedia.org/wiki/Sorting_algorithm.
Except there's a list of characters to split on, while the string split function only takes one. Iterating through the array would probably be easiest, creating a new word every time you see one of the characters that the OP mentioned. Then make the entire array of words lower case, sort the array of all the words, and then iterate over the sorted array and find all the unique words (you can figure that part out yourself ).
Depending on what sort you use this method can be faster than the one that ProgrammingFun gave (using merge sort you should end up with O(nlog(n)) instead of O(n^2) AKA faster as your input grows), but if you're going to do something like bubble sort just use the method ProgrammingFun gave (but don't forget about case-insensitivity and sorting it at the end).[/url] |
|
|
|
|
 |
magic

|
Posted: Wed Dec 29, 2010 6:37 am Post subject: RE:Java |
|
|
Thank you so much for the information..
I tried to make one but unfortunately this code is still lacking..
-All comparisons should ignore case and duplicate words..
-because I used for loop, the words to be printed are not enough..
import java.util.Scanner;
public class Main
{
public static void main(String[] args)
{
String[] Array;
Array=new String[10];
String temp;
Scanner input=new Scanner(System.in);
System.out.println("Enter Sentences:");
for(int i=0;i<10;i++){
Array[i]=input.next();
}
for(int x=1;x<10;x++)
{
for(int y=0;y<10-x;y++)
{
if(Array[y].compareTo(Array[y+1])>0)
{
temp=Array[y];
Array[y]=Array[y+1];
Array[y+1]=temp;
}
}
}
for(int i=0;i<10;i++){
System.out.println(Array[i]);
}
}
}
This is the Output:
How
a
a
chuck
if
much
wood
woodchuck
woodchuck
would |
|
|
|
|
 |
copthesaint

|
Posted: Wed Dec 29, 2010 11:17 am Post subject: RE:Java |
|
|
make a for loop that reads every charater, then when you reach a space// period record the last word in an array. After all values have been recorded, make a two dimensional array that checks if one word in the array is the same as the indexed word in the array. Of course the indexed array though should not check if it equals its self, and one a word equals another all words simular should be cleared, then the word simular to others printed .
If this helps technically I have only helped and not given you the answer. |
|
|
|
|
 |
magic

|
Posted: Thu Dec 30, 2010 1:21 am Post subject: RE:Java |
|
|
But how?
I don't know how..  |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
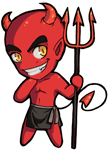
|
Posted: Thu Dec 30, 2010 2:26 am Post subject: RE:Java |
|
|
But how _what_ ? The above was a pretty detailed description with many parts to it. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
magic

|
Posted: Thu Dec 30, 2010 3:34 am Post subject: Re: Java |
|
|
SORRY |
|
|
|
|
 |
copthesaint

|
Posted: Thu Dec 30, 2010 12:04 pm Post subject: RE:Java |
|
|
An array is very simple.
To declair an array, is like declairing a normal variable.
for a single integer you would type
int varName = 0;
for an array of integers you would type
int [] varName = new int [/*Array Size*/];
and I believe that java arrays are dynamic, not completly sure I forget.
To make a two Dimentional array its as easy as adding another bound
int [][] varName = new int [/*Array Size*/][/*Array Size*/];
Now if you dont know what a two dimesional array is, think of a tree diagram or a matrix if that makes it easier, but for example purposes Ill use a tree diagram.
Ok so for example you make an array [3] by [2]
so for every one of the three branches, there are two twigs. *Or two values* anotherway of thinking of it is the parent child definition, for every parent there would be two childs.
An array basically is a variable with many variable of the same type within it.
I dont know how else to explain this and honestly I think you should know this if your teacher has given you this problem. |
|
|
|
|
 |
magic

|
Posted: Sat Jan 01, 2011 10:12 am Post subject: RE:Java |
|
|
Thank You very much.. |
|
|
|
|
 |
|
|