pointers and memory allocation (noob question)
Author |
Message |
HyperFlexed

|
Posted: Fri Jun 18, 2010 3:52 pm Post subject: pointers and memory allocation (noob question) |
|
|
I'm trying to create an array of pointers to integers. I know it's not very useful, but the fact that I'm getting segfaults tells me I'm doing something wrong.
As I understand it, this should create an array of 20 pointers to int. Where I'm running into trouble is when I try to start filling in the memory addresses those pointers reference. My suspicion is that memory isn't being allocated, so when I try something like this:
code: | int i;
for (i = 0; i < 20; ++i){
fprintf(stderr, "iteration %i", i);
*a[i] = rand();
}
|
I get a segfault. I had thought of creating a temp var to create my random value, and linking to &temp, but then all my pointers would point to the same value, even if it changed once for each assignment. How can I get memory allocated for these integers? Any help is greatly appreciated
EDIT: doing some more reading, since the values of a aren't initialized in any way, I'm trying to assign values to a null pointer (if null is the default state). This would definitely explain the segfault. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
TerranceN
|
Posted: Fri Jun 18, 2010 4:21 pm Post subject: RE:pointers and memory allocation (noob question) |
|
|
Your edit is correct. All a pointer is, is a number that points to something else. You created 20 of these pointers which don't point to anything, so trying to set the value of what they point to gives you the segmentation fault. |
|
|
|
|
 |
Tony
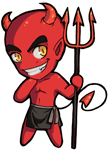
|
Posted: Fri Jun 18, 2010 4:25 pm Post subject: RE:pointers and memory allocation (noob question) |
|
|
I'm taking an educated guess that rand() returns a value on the stack -- that is not something that you want to point to, as that will disappear as soon as it's poped.
malloc some space on a heap, store the values there, and point to those locations. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
HyperFlexed

|
Posted: Fri Jun 18, 2010 4:30 pm Post subject: Re: pointers and memory allocation (noob question) |
|
|
My updated and working code is as follows:
code: | #include <stdlib.h>
#include <stdio.h>
int *a[20];
void swpptr(int *a, int *b);
void qsortptr(int *start, int *end, int *pivot);
int main(){
// debug: display RAND_MAX
printf("RAND_MAX=%u;\n", RAND_MAX);
// generate random integers and display values
int i;
for (i = 0; i < 20; ++i){
//fprintf(stderr, "iteration %i", i);
a[i] = (int*) malloc(sizeof(int));
*a[i] = rand();
printf("a[%i]=%i;\n", i, *a[i]);
}
return 0;
} |
Why would it be disadvantageous to point to something on the stack, and how do you know it's there? Under what cases might it "get popped"?
I'm not familiar with heaps. Any suggestions on learning resources? |
|
|
|
|
 |
DtY

|
Posted: Fri Jun 18, 2010 4:31 pm Post subject: Re: RE:pointers and memory allocation (noob question) |
|
|
Tony @ Fri Jun 18, 2010 4:25 pm wrote: I'm taking an educated guess that rand() returns a value on the stack -- that is not something that you want to point to, as that will disappear as soon as it's poped.
malloc some space on a heap, store the values there, and point to those locations. OP's not setting to point to rand(), they're setting the memory pointed to by the pointer to rand() (*a[ i]) |
|
|
|
|
 |
jcollins1991
|
Posted: Fri Jun 18, 2010 8:57 pm Post subject: Re: pointers and memory allocation (noob question) |
|
|
There's really nothing wrong to pointing to something on the stack... Just make sure that before you ever try to modify something referenced by a pointer make sure you've either malloc'ed / calloc'ed / realloc'ed the memory (and make sure you haven't freed it yet), or that you've set it to point to something on the stack (and that that value is still in the scope of the program you're using the pointer to access it from)...
DtY wrote:
Tony @ Fri Jun 18, 2010 4:25 pm wrote: I'm taking an educated guess that rand() returns a value on the stack -- that is not something that you want to point to, as that will disappear as soon as it's poped.
malloc some space on a heap, store the values there, and point to those locations. OP's not setting to point to rand(), they're setting the memory pointed to by the pointer to rand() ( *a[ i])
Which is why it's segfaulting, nothings been malloc'ed so the pointer isn't pointing to anything valid... |
|
|
|
|
 |
2goto1

|
Posted: Mon Jun 21, 2010 8:57 pm Post subject: Re: pointers and memory allocation (noob question) |
|
|
HyperFlexed @ Fri Jun 18, 2010 4:30 pm wrote:
Why would it be disadvantageous to point to something on the stack, and how do you know it's there? Under what cases might it "get popped"?
I'm not familiar with heaps. Any suggestions on learning resources?
Hi HyperFlexed, in general stack memory allocation is faster than heap memory allocation. That is a general rule of thumb but it can vary from compiler to compiler / platform to platform. You could always create a benchmarking test program that looped a few million times to verify the performance differences between both approaches with both small and larger memory footprint data items.
That being said the heap may be better for data consuming a larger memory footprint, and stack for data consuming a smaller footprint...not sure what the other guru's thoughts on this would be. Some research that might help you:
http://www.google.ca/search?hl=en&q=c+memory+allocation+stack+versus+heap+best+practices&aq=f&aqi=&aql=&oq=&gs_rfai=
http://www.google.ca/search?hl=en&q=c+memory+allocation+stack+versus+heap+performance&aq=f&aqi=&aql=&oq=&gs_rfai= |
|
|
|
|
 |
DemonWasp
|
Posted: Tue Jun 22, 2010 9:53 am Post subject: RE:pointers and memory allocation (noob question) |
|
|
In general, the simple rule is this:
1. If you allocate, then pass the pointer outside the function (or, more accurately, block) where it's created, it must be allocated on the heap. You will need to manually deallocate these objects.
2. If you allocate something and only use it locally, put it on the stack. It will be deallocated for you automatically when it goes out of scope. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
HyperFlexed

|
Posted: Tue Jun 22, 2010 3:47 pm Post subject: Re: pointers and memory allocation (noob question) |
|
|
so in summary, if I pass a pointer out of the block in which its created, I must deallocate manually? Seems simple enough.
EDIT: I found what seems like a pretty good description here http://www.learncpp.com/cpp-tutorial/79-the-stack-and-the-heap/
What you say makes sense now. As local variables are popped off the stack after a function call, they would go well on the stack. Large amounts of persistent data would definitely be better in the heap though. Just curious, does each program get its own stack? Or does the OS have a single stack for all programs? |
|
|
|
|
 |
Tony
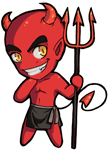
|
Posted: Tue Jun 22, 2010 4:31 pm Post subject: Re: pointers and memory allocation (noob question) |
|
|
HyperFlexed @ Tue Jun 22, 2010 3:47 pm wrote: Just curious, does each program get its own stack?
Each thread has its own stack. In most cases, a process (program) would have just a single thread, so the distinction is minimal. Things get more complicated in concurrent programming. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
DemonWasp
|
Posted: Tue Jun 22, 2010 5:08 pm Post subject: RE:pointers and memory allocation (noob question) |
|
|
It's worth noting that some less-common constructs like coroutines also have their own stacks. However, you won't see those in C or C++ or...really in any programming language in common use. |
|
|
|
|
 |
|
|