length() and substr() Are Messed Up...
Author |
Message |
HRI
|
Posted: Wed May 05, 2010 7:22 pm Post subject: length() and substr() Are Messed Up... |
|
|
For class I have to make a program that given a number between 1 and 1000, outputs that many 'moo' numbers which satisfy 2 conditions:
1. It is even.
2. It is a palindrome.
Here's my code, although I've changed it quite a bit to try to make it work. I've also never converted ints to strings before so I'm guessing that's where the problem is. I tried both sstream and itoa. Also, please mention if that break statement won't exit the for loop, as that was my intention.
#include <iostream>
using std::cout; //cout is like printf
using std::cin; //cin is like scan or whatever stdio uses
using std::endl; //new line
#include <string>
using std::string;
#include <sstream>
#include <cmath>
//I would just put 'using namespace std;', but we're not allowed.
int main()
{
bool palindrome = false; //initialize the palindrome check to false.
int i = 1, n; //i is for the current number being checked and n is for how many numbers in total.
cout << "Enter number of moo numbers: ";
cin >> n;
for (i = 1; i <= n; i++)
{
if (i % 2 == 0) //check if it's even.
{
std::stringstream ss;
ss << i; //convert number to string...***
int length = i.length(); //should work, instead says length is not a type.
for (int count = 1; count <= ceil (length / 2); count++) //This loop is to compare the first/second etc. with the last/second last etc. and see if they're the same.
{
string first = i.substr (count - 1, 1);
string second = i.substr (length - count, 1);
if (first == second)
palindrome = true;
else
break;
}
}
if (palindrome == true)
cout << i << endl;
palindrome = false;
i++;
}
system ("pause"); //for use in devcpp to pause the window so it won't close before you can read the output.
return 0;
} |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
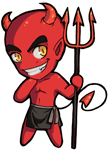
|
Posted: Wed May 05, 2010 7:33 pm Post subject: Re: length() and substr() Are Messed Up... |
|
|
HRI @ Wed May 05, 2010 7:22 pm wrote:
...
int i = 1, n; //i is for the current number being checked and n is for how many numbers in total.
...
int length = i.length(); //should work, instead says length is not a type.
"i" is not an object, and thus has no methods.
edit: what does length() of an integer even mean? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
HRI
|
Posted: Wed May 05, 2010 7:38 pm Post subject: Re: length() and substr() Are Messed Up... |
|
|
I tried to convert i to a string, so that it would be more like "22" rather than 22, for example.
Then you can call those functions using <stringname>.length/substr.
Also, I'm unaware of how to enclose my code in one of those fancy boxes. May someone enlighten me? |
|
|
|
|
 |
Tony
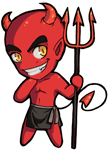
|
Posted: Wed May 05, 2010 7:46 pm Post subject: RE:length() and substr() Are Messed Up... |
|
|
C++ is a statically typed language -- you declare what type a variable "i" is (int), and it stays an int (strictly speaking, the program allocates enough memory for an int, and not enough for a larger String). "i" can't become a String.
Use [ syntax="cpp" ] [ /syntax ] tags (sans spaces) to format code blocks |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
HRI
|
Posted: Wed May 05, 2010 7:52 pm Post subject: Re: length() and substr() Are Messed Up... |
|
|
Thanks for the help, but is there no way to accomplish this then?
Thanks for the code block statements too. |
|
|
|
|
 |
OneOffDriveByPoster
|
Posted: Thu May 06, 2010 10:44 pm Post subject: Re: length() and substr() Are Messed Up... |
|
|
HRI @ Wed May 05, 2010 7:52 pm wrote: Thanks for the help, but is there no way to accomplish this then?
It just means that i is always going to be an int. It does not mean that you will not be able to produce a string with a representation of the value of i. Look at a reference for the basic_stringstream template class to see how you get a string out of a stringstream. |
|
|
|
|
 |
|
|