strcmp Source
Author |
Message |
rar

|
Posted: Thu Mar 04, 2010 9:51 am Post subject: strcmp Source |
|
|
Does anyone have the source code for C? For fun, I tried creating it myself, which I would post here but I now cannot find it....
I know that strcmp(x,y):
returns 1 if x > y
returns 0 if x = y
returns -1 if x < y
(x and y are strings, of course).
I had code written that called a function called strcmp (leaving out the string.h library) which compared them simply with the > < = operators. However, it always returned 1.
so
code: | if(x>y)
return 1; //was the case that always seemed to be called
if(x<y)
return -1;
|
So I tried changing the > < signs for each case, and then it just always returned -1. So why is x always greater than y? Clearly, I can't use these comparison operators, so how do I compare without using strcmp?
So, repeating my initial question, does anyone have the source code for strcmp? |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
chrisbrown

|
Posted: Thu Mar 04, 2010 10:50 am Post subject: RE:strcmp Source |
|
|
Don't give up, you're probably closer than you think. As far as C is concerned, strings are just arrays of characters, and characters are just integers, treated differently. So yes, you can use the same operators (<, >=, ), but they only compare one character, not the whole string. You want to check the first character of each string. If equal, move on the the next pair; if higher or lower, you're done. Note that case matters, 'a' is not equal to 'A'. |
|
|
|
|
 |
Dan
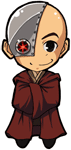
|
Posted: Thu Mar 04, 2010 10:54 am Post subject: RE:strcmp Source |
|
|
There is no singal "source code for C". There are source codes for C compliers (such as gcc) and source codes for C libbaires such as string.h (which i think you want). Even then there are diffrent versions of the same libbariy and diffrent implmentations of it on diffrent platforms.
For GNU GLIBC the strcmp function looks like this:
c: |
/* Copyright (C) 1991, 1996, 1997, 2003 Free Software Foundation, Inc.
This file is part of the GNU C Library.
The GNU C Library is free software; you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public
License as published by the Free Software Foundation; either
version 2.1 of the License, or (at your option) any later version.
The GNU C Library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with the GNU C Library; if not, write to the Free
Software Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA
02111-1307 USA. */
#include <string.h>
#include <memcopy.h>
#undef strcmp
/* Compare S1 and S2, returning less than, equal to or
greater than zero if S1 is lexicographically less than,
equal to or greater than S2. */
int
strcmp (p1, p2)
const char *p1;
const char *p2;
{
register const unsigned char *s1 = (const unsigned char *) p1;
register const unsigned char *s2 = (const unsigned char *) p2;
unsigned reg_char c1, c2;
do
{
c1 = (unsigned char) *s1++;
c2 = (unsigned char) *s2++;
if (c1 == '\0')
return c1 - c2;
}
while (c1 == c2);
return c1 - c2;
}
libc_hidden_builtin_def (strcmp)
|
|
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
Alexmula
|
Posted: Thu Mar 04, 2010 10:58 am Post subject: Re: strcmp Source |
|
|
rar @ Thu Mar 04, 2010 9:51 am wrote:
I know that strcmp(x,y):
returns 1 if x > y
returns 0 if x = y
returns -1 if x < y
the lab instructor was actually wrong. you must listen to what kobti says. |
|
|
|
|
 |
rar

|
Posted: Thu Mar 04, 2010 11:51 am Post subject: RE:strcmp Source |
|
|
How so? |
|
|
|
|
 |
Alexmula
|
Posted: Thu Mar 04, 2010 2:17 pm Post subject: RE:strcmp Source |
|
|
strcmp("a","A") returns 32 not 1 |
|
|
|
|
 |
McCulloch

|
Posted: Thu Mar 04, 2010 2:20 pm Post subject: Re: strcmp Source |
|
|
The lab instructor said that
strcmp(x,y):
returns 1 if x > y
returns 0 if x = y
returns -1 if x < y
but he messed up a really meant
strcmp(x,y):
returns a positive number if x > y
returns 0 if x = y
returns a negative number if x < y
you could have simply figured this out by testing out the function strcmp
printf("%d\n", strcmp(x,y)); |
|
|
|
|
 |
rar

|
Posted: Thu Mar 04, 2010 4:16 pm Post subject: RE:strcmp Source |
|
|
Yeah ok, but 1 is a positive number and -1 is a negative number, which also happen to be the numbers that print when I do that test. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
chrisbrown

|
Posted: Thu Mar 04, 2010 4:57 pm Post subject: RE:strcmp Source |
|
|
What strings are you using to test? "x" and "y" will give you +- 1; so will "aaaa" and "aaab". |
|
|
|
|
 |
DtY

|
Posted: Thu Mar 04, 2010 8:34 pm Post subject: RE:strcmp Source |
|
|
The C standard says that strcmp will return a positive, negative or zero depending on the input. Those numbers may or may not be (negative) one, or they may be any other number.
Mostly reiterating what has been said, but hopefully in a more concise way;
c: | int strcmp(const char *a, const char *b) {
if (a > b) return 1; //or any other positive number
else if (a == b) return 0;
else return -1;
} |
Will check value of `a` and `b`, which are pointers, so it will actually check a's address to b's, not what you want.
To actually compare the strings, you need to check character by character. If every character in the strings is identical, it returns zero. If they aren't, the comparison is the same as comparing the characters at the position where `a` and `b` have different values.
See the code Dan posted for a specific way of doing this. As you can see:
Is how the actual value is determined, if c1>c2, that will turn out positive, but if c2>c1, that will be positive, not just one or negative one.
Since the C standard does not have a specific return value specified, they can save using two more ifs and just return the difference. |
|
|
|
|
 |
rar

|
Posted: Tue Mar 09, 2010 7:18 pm Post subject: Re: RE:strcmp Source |
|
|
Dan @ Thu Mar 04, 2010 10:54 am wrote: There is no singal "source code for C". There are source codes for C compliers (such as gcc) and source codes for C libbaires such as string.h (which i think you want). Even then there are diffrent versions of the same libbariy and diffrent implmentations of it on diffrent platforms.
For GNU GLIBC the strcmp function looks like this:
c: |
/* Copyright (C) 1991, 1996, 1997, 2003 Free Software Foundation, Inc.
This file is part of the GNU C Library.
The GNU C Library is free software; you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public
License as published by the Free Software Foundation; either
version 2.1 of the License, or (at your option) any later version.
The GNU C Library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with the GNU C Library; if not, write to the Free
Software Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA
02111-1307 USA. */
#include <string.h>
#include <memcopy.h>
#undef strcmp
/* Compare S1 and S2, returning less than, equal to or
greater than zero if S1 is lexicographically less than,
equal to or greater than S2. */
int
strcmp (p1, p2)
const char *p1;
const char *p2;
{
register const unsigned char *s1 = (const unsigned char *) p1;
register const unsigned char *s2 = (const unsigned char *) p2;
unsigned reg_char c1, c2;
do
{
c1 = (unsigned char) *s1++;
c2 = (unsigned char) *s2++;
if (c1 == '\0')
return c1 - c2;
}
while (c1 == c2);
return c1 - c2;
}
libc_hidden_builtin_def (strcmp)
|
Hmm...we haven't learned anything about unsigned char yet, so I don't think that's what he's looking for...besides that, the code looks correct from what I understand.. |
|
|
|
|
 |
DtY

|
Posted: Tue Mar 09, 2010 7:20 pm Post subject: RE:strcmp Source |
|
|
Do you know what unsigned ints are? Unsigned char is the same thing, except that it is shorter. |
|
|
|
|
 |
Euphoracle

|
Posted: Tue Mar 09, 2010 7:39 pm Post subject: RE:strcmp Source |
|
|
An unsigned char lacks a sign bit (meaning that there is no negative representation)
Basically, it's -127 to 127, vs 0 to 255.
In binary, negative numbers are represented using the sign bit and two's compliment: http://en.wikipedia.org/wiki/Signed_number_representations |
|
|
|
|
 |
Dan
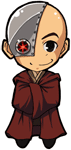
|
Posted: Tue Mar 09, 2010 8:06 pm Post subject: Re: RE:strcmp Source |
|
|
rar @ 9th March 2010, 7:18 pm wrote:
Hmm...we haven't learned anything about unsigned char yet, so I don't think that's what he's looking for...besides that, the code looks correct from what I understand..
Well that's the source code for strcmp (at least glibs version, i did not make that code it is from the acuatal source code of the library). I think they want you to make your own version tho. |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
rar

|
Posted: Tue Mar 09, 2010 8:44 pm Post subject: RE:strcmp Source |
|
|
Yeah that's what I'm thinking. It wasn't specifically asked of us, it was just suggested as practice. And then hinted towards with reference to a midterm. So I was hoping to get some insight.
The code was helpful, perhaps I'll give the code another shot (I'd post my current attempt, but I have no idea what happened to it...) |
|
|
|
|
 |
|
|