A tutorial: the beginning
Author |
Message |
wtd
|
Posted: Tue Nov 01, 2005 1:26 pm Post subject: A tutorial: the beginning |
|
|
If there is any merit to continuing this, please let me know.
So you want to learn C++?
I'll tell you something up front. C++ is a big, complex language with lots of very subtle ways to screw up, and the tools aren't going to provide much of a safety net.
You may want to learn C++ because that's what all of the games you play are written in, and because C++ programs can be really fast. Yes, these things are true. Guess what, though? It doesn't matter. If you're a novice programmer, still learning basic programming concepts, C++ makes learning them much harder than it has to be. It'll be hard enough without using tools that go out of their way to confuse you.
You should be looking elsewhere.
What do you need?
Ideally, you should be using a *nix-based OS, like Linux, *BSD, or Mac OS X, but since most of you are likely using Windows, these directions will be tailored to you.
Gandalf's excellent thread on this subject is where you should look.
Aside from this, you need a basic understanding of the Windows command-line. Understand directories, and how to navigate between them. Also understand how to invoke programs and pass arguments and options to them.
Hello, world!
The classic beginning.
c++: | #include <iostream>
int main()
{
std::cout << "Hello, world!" << std::endl;
return 0;
} |
Organization
All executable code in C++ is organized into functions. The "main" function is just such a function. It serves as the "entry point" for the program. When the program is run, this is where execution starts.
We can of course create our own functions, and I'll get to that eventually.
It should be noted that even things which do not look like functions can be. In the "Hello, world!" example, the << operator is a function.
The simplest explanation of functions is that they translate one or more values to some new value. In the case of main, the parentheses following the name "main" are empty, so the function essentially translates nothing to some "int" value which represents the success or failure of the program. Zero is success, and anything ese is some kind of failure.
Of course, in the process of doing that translation, side-effects may occur which change the environment. Printing a message to the screen would count as a side-effect.
Simple output
The "cout" in "std::cout" is short for "common output". This is almost always represented by the console. The << operator is a nice visual cue that the information on the right hand side of the operator is being sent to the output channel on the left hand side.
We can see this in the Hello world program.
c++: | std::cout << "Hello, world!" |
Of course, that doesn't explain How two of those operators got in there.
c++: | "Hello, world!" << std::endl |
Doesn't make sense.
It makes sense, however, if we consider that << is a function which takes an output channel and a string (or many other kinds of things) and translates them to the same output channel.
c++: | std::cout << "Hello, world!" << std::endl; |
Becomes a convenient shorthand for:
c++: | std::cout << "Hello, world!";
std::cout << std::endl; |
Declaring variables
Variables provide us a convenient place to store data, and allow us to give meaningful names to that data.
To create a variable, it must first be declared. This declaration provides us with information on the name of the variable, and the type of data which can be stored in it. Once we associate a particular type with that variable, we cannot store any other type in it.
The above declares a simple "int" variable called "myNumber". All other declarations follow this simple pattern.
Variables should be declared within functions such as "main". They can be declared outside of functions, but these are called "global variables", and are very difficult to keep track of in larger programs as well as making small programs harder to expand.
Good:
c++: | int main()
{
int myInteger;
return 0;
} |
Bad:
c++: | int myInteger;
int main()
{
return 0;
} |
Initializing variables
When we declare a variable, we specify its name, and the type of data it can hold. For many data types, though, including the simple ones we're using now, simply declaring the variable does not give it an initial value that we can work with.
Declaring a variable without initializing it will often, in fact, result in a random value being stored in that variable.
So, how do we initialize a variable?
There are two answers, and the differences between them only arise when dealing with more complex data.
c++: | #include <iostream>
int main()
{
int myInteger = 42;
std::cout << myInteger << std::endl;
return 0;
} |
c++: | #include <iostream>
int main()
{
int myInteger(42);
std::cout << myInteger << std::endl;
return 0;
} |
Why are they called "variable"?
Because their values can change. This change is affected through the use of assignment. We "assign" a new value to the variable.
c++: | #include <iostream>
int main()
{
int myInteger = 42;
std::cout << myInteger << std::endl;
myInteger = 27;
std::cout << myInteger << std::endl;
return 0;
} |
You'll notice that assignment looks an awful lot like one of the forms of assignment. There's a good reason for this.
That form of initialization is assignment. It's just rolled into one tidy package with declaration. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
[Gandalf]

|
Posted: Tue Nov 01, 2005 4:36 pm Post subject: (No subject) |
|
|
Thanks for the compliment and the nice beginners tutorial .
It looks like a good idea, I see this going somewhere like the Java thread? This sure could come in handy in the future, but I guess it depends on the amount of people who actually want it and care. |
|
|
|
|
 |
varun_dave
|
Posted: Wed Feb 07, 2007 8:22 pm Post subject: RE:A tutorial: the beginning |
|
|
Thank you i liked this.. |
|
|
|
|
 |
parul1234
|
Posted: Mon Jul 27, 2009 2:01 pm Post subject: RE:A tutorial: the beginning |
|
|
Nice example thanks for that . |
|
|
|
|
 |
LearningC++
|
Posted: Mon Nov 23, 2009 12:14 am Post subject: Re: A tutorial: the beginning |
|
|
So I just started learning C++ today and the tutorial I'm using starts every program with:
#include <iostream>
using namespace std;
I noticed you put the std part in another place. Why is it different? |
|
|
|
|
 |
DemonWasp
|
Posted: Mon Nov 23, 2009 1:57 am Post subject: RE:A tutorial: the beginning |
|
|
It can be specified in either place because std is a namespace. Namespaces are used so you can have two different functions use the same name and the compiler can tell the difference. You can either specify them on each use of a symbol that (might) appear to have multiple definitions, or you can specify that you're using that namespace and the compiler should assume that when you refer to "foo", you mean the one in that namespace. |
|
|
|
|
 |
Tony
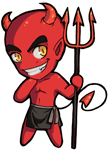
|
Posted: Mon Nov 23, 2009 2:10 am Post subject: RE:A tutorial: the beginning |
|
|
Because this tutorial is an introduction, and you don't yet need to worry about namespace. The line
code: |
using namespace std;
|
Is avoided, so that you wouldn't have to ask what it does. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
LearningC++
|
Posted: Mon Nov 23, 2009 6:12 pm Post subject: Re: A tutorial: the beginning |
|
|
So it's better to do it this way?
#include <iostream>
using namespace std; |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Mon Nov 23, 2009 6:33 pm Post subject: RE:A tutorial: the beginning |
|
|
It is different, and potentially worse if you don't know what it means. |
|
|
|
|
 |
Hoshi
|
Posted: Fri Dec 16, 2011 11:01 am Post subject: RE:A tutorial: the beginning |
|
|
How do I code C++? With what software(s)? What is used to write in the language? |
|
|
|
|
 |
|
|