Author |
Message |
krishon
|
Posted: Sun Nov 09, 2003 12:13 pm Post subject: turning an integer to a string |
|
|
do u use String.parseString(theinteger) or something like that cuz it dusn't work in my program. I'm using it cuz i initialized i as an integer in a for loop, and since my program is used to check if a number turned 180 degrees will be the same, i need to find the length of a range of numbers. newayz, ne help will be appreciated. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
rizzix
|
Posted: Sun Nov 09, 2003 12:37 pm Post subject: (No subject) |
|
|
well now that you are using ver 1.4.2 (the latest) here's the recomended way to do it:
code: |
// instance method call
int i = 0;
String str = (new Integer(i)).toString();
|
or
code: |
// static method call
int i = 0;
String str = Integer.toString(i);
|
or
code: |
// static method call
int i = 0;
String str = String.valueOf(i);
|
or
code: |
// concatenation (same thing as instance method call)
int i = 0;
String str = i + "";
|
the upcomming version of java (1.5) will also let u you do this:
code: |
// autoboxing method call
int i = 0;
String str = i.toString();
|
|
|
|
|
|
 |
krishon
|
Posted: Mon Nov 10, 2003 7:46 pm Post subject: (No subject) |
|
|
icic...knew i had to use toString(), but i dun't like the api's.... |
|
|
|
|
 |
krishon
|
Posted: Mon Nov 10, 2003 7:53 pm Post subject: (No subject) |
|
|
sry..got 2 more questions
1. i have o = Integer.parseString(i), y won't it let me do int a = Integer.parseInt(o.charAt(1));
2. if u fix problem number 1, cuz i split the input of numbers into strings, convert them back into numer, how can i turn a number backwords e.g. 54321 to 12345.
thx |
|
|
|
|
 |
Tony
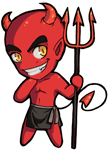
|
Posted: Mon Nov 10, 2003 8:13 pm Post subject: (No subject) |
|
|
well since you're converting a string to integer, shouldn't it be
a = String.parseInt(o.charAt(1)); ? |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
krishon
|
Posted: Mon Nov 10, 2003 10:24 pm Post subject: (No subject) |
|
|
probably...oooooo that's probably y they have the Integer, STring or wutever in front....i never knew that...k that makes sense thx |
|
|
|
|
 |
rizzix
|
Posted: Tue Nov 11, 2003 4:42 pm Post subject: (No subject) |
|
|
krishon wrote: sry..got 2 more questions
1. i have o = Integer.parseString(i), y won't it let me do int a = Integer.parseInt(o.charAt(1));
2. if u fix problem number 1, cuz i split the input of numbers into strings, convert them back into numer, how can i turn a number backwords e.g. 54321 to 12345.
thx
1) it won't let u do Integer.parseInt(o.charAt(1)); cuz ur not passing a string as it's arguments.. to fix it contatenate the char with an enpty string like this:
int x = Integer.parseInt(o.charAt(1)+"");
2) well make it simple here once u divide the string into 2 parts u get two different strings. so lets say str1 = "54321" and str2 = "6789", and now u want an interger from the reverse order of str1 (i.e 12345), u would do this:
code: |
StringBuffer sb = new StringBuffer(str1);
sb.reverse();
int x = Integer.parseInt(sb.toString());
System.out.println(x);
|
|
|
|
|
|
 |
krishon
|
Posted: Tue Nov 11, 2003 7:07 pm Post subject: (No subject) |
|
|
java has a method that can reverse digits woulda been helpful if that wuz in turing last year...coulda saved soo much time. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
krishon
|
Posted: Tue Nov 11, 2003 7:45 pm Post subject: (No subject) |
|
|
k, i've done most of the question...this part eludes me the most.....
how can u figure out if a number flipped 180 will be the same
i took the reverse with the help of rizzix, i tried to say that the different the number and its reverse must be positive, but that didn't work as 69 and 96 are reversed, but 69-96 is negative.....i thought that having a difference of 0 won't work, as the 69 and 96 pair again breaks it. I also tried doin that any number divided by 11 that gives a remainder of 0 cannot be included, but 88 and 11 break that....i'm so confused now...i have no clue how to fix it ne help will be great |
|
|
|
|
 |
Tony
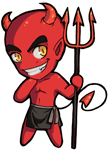
|
Posted: Tue Nov 11, 2003 8:05 pm Post subject: (No subject) |
|
|
well you have to make sure that the number only contains flippable digits -0, 1, 8. And replace 6s and 9s. Then see if number reads same backwards. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
krishon
|
Posted: Sat Nov 15, 2003 7:17 pm Post subject: (No subject) |
|
|
this is bout some other question now....didn't wanna waste space by making another thread......how do u convert a string to a character, thx |
|
|
|
|
 |
krishon
|
Posted: Sat Nov 15, 2003 7:41 pm Post subject: (No subject) |
|
|
scratch that..i found out how to do it, just use input.charAt(0)...can't believe i didn't think of it earlier |
|
|
|
|
 |
rizzix
|
Posted: Sat Nov 15, 2003 9:28 pm Post subject: (No subject) |
|
|
krishon wrote: java has a method that can reverse digits  woulda been helpful if that wuz in turing last year...coulda saved soo much time.
wierd eh? there's really nothing much to implement ur self.. now all u have to do is problem-sove  |
|
|
|
|
 |
krishon
|
Posted: Sat Nov 15, 2003 11:09 pm Post subject: (No subject) |
|
|
yah...plus it'll be ezier to do the CCC with java...how did u find out bout all these methods newayz? |
|
|
|
|
 |
rizzix
|
Posted: Sat Nov 15, 2003 11:22 pm Post subject: (No subject) |
|
|
the API documentation by sun: http://java.sun.com/j2se/1.4.2/docs/api/index.html
you need to keep in mind that most of the time ur either gonna use the java.util, java.io, java.net, (even java.sql if ur into databases), java.awt or the javax.swing package
and u'll always will be using the java.lang package (whether u like it or not)
so just bookmark those |
|
|
|
|
 |
|