[Tutorial] XML-RPC Web Service (Windows)
Author |
Message |
rizzix
|
Posted: Sun Oct 05, 2003 6:52 pm Post subject: [Tutorial] XML-RPC Web Service (Windows) |
|
|
This is a quick tutorial on implementing an xml-rpc web service in c++.
I've attaced the library and tested it. Do read the readme, it has the settings necessary for getting your projects to work.
Here's how to implement it...
The Server:
code: |
#include "XmlRpc.h"
#include <stdlib.h>
using namespace XmlRpc;
XmlRpcServer s;
class TestMethod : public XmlRpcServerMethod
{
public:
TestMethod(XmlRpcServer* s1) : XmlRpcServerMethod("test", s1) {}
void execute(XmlRpcValue& params, XmlRpcValue& result)
{
result = "It Works! Yahooooooooooo!";
}
} test(&s);
int main(int argc, char* argv[])
{
int port = 8000;
//XmlRpc::setVerbosity(5);
s.bindAndListen(port);
s.enableIntrospection(true);
s.work(-1.0);
return 0;
}
|
as you can see it's all self explanatory.
We first include the "XmlRpc.h" header file. Next we start using the namespace XmlRpc, since all the necessary classes are defined in that namespace.
We first write a class defining a Remote Procedure. It's constructor takes an arguement of XmlRpcServer and we initialize the object by passing the name we of the method to register this method with (int this case "test") and the XmlRpcServer to which the method will be registered to, to it's super class's constructor. We then overload the execute method that has two arguments one is the parameters supplied the other the result to be returned.
Then in the main mehtod we bind the server to the port 8000. The next line states that we enable introspection, which basically means we want the library itself to figure out the parameter types, return types etc. The work method that is called is passed the time in milliseconds you want the server to run, a value of -1 means continously until interrupted (i.e interrupted by Ctrl+c).
The Client:
code: |
#include "XmlRpc.h"
#include <iostream>
using namespace XmlRpc;
int main(int argc, char* argv[])
{
int port = 8000;
//XmlRpc::setVerbosity(5);
XmlRpcClient c("127.0.0.1", port);
XmlRpcValue noArgs, result;
if (c.execute("test", noArgs, result))
std::cout << "\nResult:\n " << result << "\n\n";
else
std::cout << "Error calling 'listMethods'\n\n";
return 0;
}
|
This too is pretty simple as you can see. We again have to include the #include "XmlRpc.h" header file and we use the namespace XmlRpc. In the main method we create a new XmlRpcClient object which represents the connection to the server. We pass it the server ip address and port number.
Then define two XmlRpcValue objects that basically represent the "types" for our method(s). We pass those objects (by reference) to the execute method of the client object along with the name of the method we want to invoke(in this case "test"). the reurn value is stored in result (the 3rd argument).
NOTE: make sure you finally check the project settings, especially the linker library paths and preprocessor include paths
VC++: download library/header files here
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
rizzix
|
Posted: Sun Oct 05, 2003 7:10 pm Post subject: (No subject) |
|
|
ok i have a problem uploading the file(aprox 3mb).. can an admin get in contact with me, and upload the file?
|
|
|
|
|
 |
Tony
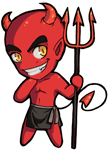
|
|
|
|
 |
rizzix
|
Posted: Wed Oct 08, 2003 3:53 pm Post subject: (No subject) |
|
|
i've compiled a Dev-C++ version of the file.. so for those folks.. here's it.
Description: |
|
 Download |
Filename: |
libxmlrpc.zip |
Filesize: |
91.53 KB |
Downloaded: |
597 Time(s) |
|
|
|
|
|
 |
rizzix
|
Posted: Wed Oct 08, 2003 4:47 pm Post subject: (No subject) |
|
|
by the way i tiried the devc++ version.. and woops heh.. yea ok.. i now uploaded a new static lib file.
to get ur project to succesfully compile and link you need to do this:
1) click "project"->"project options"
2) click on the "parameters" tab
3) in the "Linker" text area box type this in: "-lws2_32 -lxmlrpc -lwsock32" (without the " of course)
4) click the "directories" tab, then select the tab "Library directories" and add the location of the the directory where the libxmlrpc.a file is located
5) click the "Include directories" tab and add the location of the directory that contains the .h header files.
thats all.
|
|
|
|
|
 |
|
|