Attempt to use Null Pointer (java.lang.NullPointerException)
Author |
Message |
S_Grimm

|
Posted: Thu Oct 30, 2008 10:35 am Post subject: Attempt to use Null Pointer (java.lang.NullPointerException) |
|
|
When i try to run the code it tells me that there is a null pointer exception. what does that mean? and how do i fix it?
Java: |
//Chat Client Version 2.2 works with any chatServer
import java.net.*;
import java.io.*;
public class chatClientv2p2
{
//variable declarations
public static Socket mySocket;
public static String datavar;
public static String name;
public static InputStreamReader isr;
public static BufferedReader br;
public static InputStreamReader reader = new InputStreamReader (System. in);
public static BufferedReader bread = new BufferedReader (reader );
public static PrintWriter pw;
//thread declarations
/*
public static class Connect extends Thread
{
public void run ()
{
try
{
synchronized (mySocket)
{
}
//synchronized (pw)
//{
//
//}
}
catch (Exception e)
{
System.out.println ("Error connecting to host");
}
}
}
*/
public static class Write extends Thread
{
public void run ()
{
try
{
synchronized (datavar )
{
datavar = br. readLine ();
System. out. println (datavar );
}
}
catch (Exception e )
{
System. out. println ("Unable to recieve message");
}
}
}
public static class Read extends Thread
{
public void run ()
{
try
{
pw. println (name + bread. readLine ());
}
catch (Exception e )
{
}
}
}
//main method
public static void main (String [] args ) throws IOException
{
synchronized (mySocket ) //null pointer exception here.
{
try
{
mySocket = new Socket ("10.217.105.40", 5050); //input your server or host ip address
}
catch (Exception e )
{
}
}
//new Connect ().start();
InetAddress lclAdr = InetAddress. getLocalHost ();
System. out. println ("Your IP address is " + lclAdr. getHostAddress ());
// = new InputStreamReader (mySocket.getInputStream ());
//= new BufferedReader (isr);
synchronized (pw )
{
pw = new PrintWriter (mySocket. getOutputStream (), true);
}
System. out. print ("Input Screen Name : ");
synchronized (name )
{
name = bread. readLine ();
name = (name + " says : ");
}
new Write (). setPriority (1);
new Read (). setPriority (2);
for (;; )
{
new Write (). run ();
new Read (). run ();
}
}
}
|
|
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
btiffin

|
Posted: Thu Oct 30, 2008 12:06 pm Post subject: RE:Attempt to use Null Pointer (java.lang.NullPointerException) |
|
|
Well, I can tell you what it means, but not how to fix it other than this just doesn't look right.
A NullPointerException is usually caused by access to uninitialized data. In this case you are asking Java to check the object monitor for mySocket before mySocket is initialized.
I'm not a Java head by any means, but you usually surround sychronized around critical code sections that may use/modify an object, but I don't think that can include the instantiation.
Cheers |
|
|
|
|
 |
Tony
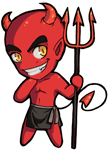
|
Posted: Thu Oct 30, 2008 12:23 pm Post subject: RE:Attempt to use Null Pointer (java.lang.NullPointerException) |
|
|
In Java's case, it's likely that you are calling a method on an object that is actually NULL (as btiffin says -- has not been initialized).
Look through the stack trace to see what line the problem originates at. |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
S_Grimm

|
Posted: Thu Oct 30, 2008 3:03 pm Post subject: Re: Attempt to use Null Pointer (java.lang.NullPointerException) |
|
|
Quote: Java: | public static void main (String [] args ) throws IOException
{
synchronized (mySocket ) //null pointer exception here.
{
try
{
mySocket = new Socket ("10.217.105.40", 5050); //input your server or host ip address
}
catch (Exception e )
{
}
} |
thanks biffin. i removed the snyc and it worked. |
|
|
|
|
 |
btiffin

|
Posted: Thu Oct 30, 2008 5:36 pm Post subject: RE:Attempt to use Null Pointer (java.lang.NullPointerException) |
|
|
No problem A\V
Instead of synchronized and wrapping all the snippets, you may want to look into java.util.concurrent (Assuming Java > 1.5) and use a Semaphore. Higher level abstraction and a little less finicky.
synchronized enclosures have quite a few "holes" that need care and attention. Semaphores are external to the Objects and methods needing special protection measures and will usually be easier to manage/debug.
Multi-threading gotchas can be a real bitch to catch, when they may only happen every 8,003 runs or on full moon Tuesdays when someone in Norway sneezes.
Cheers |
|
|
|
|
 |
Dion van Overdijk
|
Posted: Sun Dec 06, 2009 7:37 am Post subject: Re: Attempt to use Null Pointer (java.lang.NullPointerException) |
|
|
Hi guys I've a similar problem where I get a NullPointerException
The exception points to a line of code in a XMLRPCClient file where it asks for the execution of a server procedure
the line in the client: object result = client.execute("serverHandler.getTelNo", argument);
The server has a server object with a handler as follows: server.addHandler("serverHandler", new TelNos());
TelNos is a separate java class on the server for which the code is below:
What am I doing wrong?
package Server;
import java.util.*;
public class TelNos {
//database to hold names and telephone numbers
private Hashtable hashTab;
public TelNos() {
Hashtable<String, Integer> hashTab = new Hashtable<String, Integer>();
hashTab.put("Thomas", 9088);
hashTab.put("Wilkinson",7856);
hashTab.put("Davies", 2234);
hashTab.put("Mabbut", 2267);
hashTab.put("Tomkins", 4456);
hashTab.put("Price", 3377);
hashTab.put("Wilson", 2300);
}
/*I did try this as well with a main method but the result is the same*/
/*public static void main(String [] args){
Hashtable<String, Integer> hashTab = new Hashtable<String, Integer>();
try{
hashTab.put("Thomas", 9088);
hashTab.put("Wilkinson",7856);
hashTab.put("Davies", 2234);
hashTab.put("Mabbut", 2267);
hashTab.put("Tomkins", 4456);
hashTab.put("Price", 3377);
hashTab.put("Wilson", 2300);
}
catch(Exception e){
e.printStackTrace();
}
}*/
public int noStaff(){
//Return the number of staff in the database
return hashTab.size();
}
public int getTelNo(String staffName){
//Return the tel no of the argument
Integer n = (Integer)hashTab.get(staffName);
return n;
}
public Vector getNames(){
//Returns with a vector containing the names of all the staff
Vector names = (Vector)hashTab.keys();
return names;
}
} |
|
|
|
|
 |
|
|