Author |
Message |
abcdefghijklmnopqrstuvwxy
|
Posted: Sun Mar 25, 2007 7:17 am Post subject: Are you as genius as me? |
|
|
Hi, I've been trying to prove to myself that I'm a genius. If more than one other person can figure this out by themselves then I guess I gotta go figure something else out and make a similar post.
Here's the "test".
We all know the familiar swap function. It's very easy to do when you are allowed to make an extra variable (temp), but what if you didn't have this variable?
Given two integers, x and y, swap x's value into y and y's value into x WITHOUT any additional variables.
code: |
void swap(unsigned int& x, unsigned int& y) {
//no variable declarations under no circumstances. However you may make as much use as you wish of x and y and operators and if statements.
//at the end of this function x's original value should be y's initial value and vice versa.
}
|
I won't give any hints just yet... I was able to come up with a formula in a little less than 10 minutes. And it took another 20 to test the formula. (and it passed all my tests).
Oh yeah, don't worry about negative numbers (although it can be done), just make it work with positive integers and that's good enough.
Allowed Equipment: paper, pen, text editor, c++ compiler(not needed though).
Definitely NOT Allowed: Internet.
I'm willing to bet less than 1% of the worlds population can figure this out in less than an hour if they followed my rules. So please don't cheat in order to prove me wrong. If you're not smarter than me than at least have some honor... |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
wtd
|
Posted: Sun Mar 25, 2007 8:37 am Post subject: RE:Are you as genius as me? |
|
|
code: | void swap(unsigned int& x, unsigned int& y)
{
x = x ^ y;
y = x ^ y;
x = y ^ x;
} |
|
|
|
|
|
 |
Mazer

|
Posted: Sun Mar 25, 2007 8:52 am Post subject: Re: Are you as genius as me? |
|
|
[quote="abcdefghijklmnopqrstuvwxy @ Sun Mar 25, 2007 7:17 am"]
stuff
[quote]
How does that make you a genius? Did you actually figure the xor trick out by yourself, or did you see it on the internet/in a book like pretty much everyone else? |
|
|
|
|
 |
CodeMonkey2000
|
Posted: Sun Mar 25, 2007 9:48 am Post subject: RE:Are you as genius as me? |
|
|
I don't know C++ but meh... |
|
|
|
|
 |
Cervantes

|
Posted: Sun Mar 25, 2007 12:35 pm Post subject: RE:Are you as genius as me? |
|
|
I remembered it had something to do with xor, then it took me about 2 minutes to get it.
This is really silly. |
|
|
|
|
 |
wtd
|
Posted: Sun Mar 25, 2007 1:13 pm Post subject: RE:Are you as genius as me? |
|
|
Now, let's see if Mr. alphabet-man is as genius as me.
Write a function in point-free style in Haskell which expresses the product of a range of numbers from one to a given natural number using a fold. |
|
|
|
|
 |
Dan
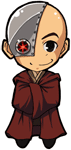
|
Posted: Sun Mar 25, 2007 1:57 pm Post subject: RE:Are you as genius as me? |
|
|
I might not be a "genius" but is doing 6 operations for a swap function when you can do 3 ( or 4 depending on the complier/cpu) at the cost of temperaly using 4 bytes in the ram worth it?
I am not shure of how much time it takes on most cpu/artechchers to alocate 4 bytes in ram vs do 3 math operations but i have a fealing that the noraml swap method is going to be faster.
This may not matter if you are only runing swap one time but if you run it many many times it could start adding up.
I tryed runing some tests on my laptop, however the code runs so fast it is hard to get a vaild result of the excuction time even when i made it run each swap function 1000000 times inbtween time readings.
my results where:
The standard swap funciton: 4 to 6 millisceonds
WTD's swap function: 6 to 9 milliseconds
CodeMonkey's swap function: 6 to 8 milliseconds
This was for runing the function 1000000 times on a P4 3.2ghz and taking a time reading befor and affter the 1000000 runs. I ran the test about 20 times for each function. |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
abcdefghijklmnopqrstuvwxy
|
Posted: Sun Mar 25, 2007 4:29 pm Post subject: RE:Are you as genius as me? |
|
|
Oh yeah I forgot about the xor way. I'll edit my original post to state that you can't use xor either.
The reason xor is not a valid way to prove you are as genius as me is because of what mazer said, it is so very unlikely that you figured it out by yourself wtd, but what you actually did was recall the way to do it after having seen it somewhere before.
So, its a shame you've all be side tracked. I still won't give any hints as I'm sure no one has been working on it because you thought wtd actually solved it.
Again, this "test" has not yet been passed!
EDIT: I can't edit my original post, so I'll add the rule here. You cannot use xor, and, or operators.
EDIT:
I just noticed codemonkey's post.
This meets the criteria, well done! It's not the way I did it, but it's actually a faster way requiring less steps. However since you posted this on the forum for everyone else to see I can't determine if more than 1 of you could figure it out unaided! Also forgot to mention to PM me, argh! |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
klopyrev
|
Posted: Sun Mar 25, 2007 4:45 pm Post subject: Re: Are you as genius as me? |
|
|
CodeMonkey's way is not valid then?
EDIT: Oh, so he's more of a genius than you then? |
|
|
|
|
 |
abcdefghijklmnopqrstuvwxy
|
Posted: Sun Mar 25, 2007 5:00 pm Post subject: RE:Are you as genius as me? |
|
|
code: |
CodeMonkey's way is not valid then?
|
That would be a foregone conclusion.
I'm sure codemonkey is honorable and he did figure this out all by himself and not from memory or from looking it up on the internet, but it is still very possible, judging by the succinctness, that his code was copied. Usually someones first solution isn't the BEST solution, and codemonkey's was surely the best so it is a little suspicious, but I will choose to believe that he figured it out on his own!
Before I post my code I'll add the second part to my test.
The Test (part 2)
Design a swap function that works for both positive and negative numbers and does not use codemonkey's method for the positive numbers. (make a new algorithm on your own!) or (wtd's obviously). Part 2 still follows all of the rules from Part 1. (obviously)
PM me your answers. Once more than one person has pm'd me an answer I'll post all the answers including my own.
Good luck! |
|
|
|
|
 |
abcdefghijklmnopqrstuvwxy
|
Posted: Sun Mar 25, 2007 5:12 pm Post subject: RE:Are you as genius as me? |
|
|
Quote:
Now, let's see if Mr. alphabet-man is as genius as me.
Write a function in point-free style in Haskell which expresses the product of a range of numbers from one to a given natural number using a fold.
This is not how you calculate someone's intelligence. I have never used Haskell, I don't know what a "fold" is in this context. And I don't know what a natural number is.
A problem which is meant to test someone's intelligence should require no more tools than the knowledge within their minds.
That's why my question would be unfair to those not knowing what a variable is in programming, and the ability to perform grade 6 mathematics.
I, being the shrewd fellow that I am, assumed that all of you meet those requirements thus yielding my test to be well-suited. |
|
|
|
|
 |
klopyrev
|
Posted: Sun Mar 25, 2007 5:19 pm Post subject: Re: Are you as genius as me? |
|
|
Codemonkey's way works for negative numbers too. Wouldn't it still be the best solution then?
KL |
|
|
|
|
 |
Dan
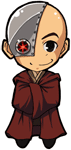
|
Posted: Sun Mar 25, 2007 5:26 pm Post subject: Re: Are you as genius as me? |
|
|
William Shakespeare wrote:
A fool thinks himself to be wise,
but a wise man knows himself to be a fool.
Topic Locked. |
Computer Science Canada
Help with programming in C, C++, Java, PHP, Ruby, Turing, VB and more! |
|
|
|
 |
Clayton

|
Posted: Sun Mar 25, 2007 5:26 pm Post subject: RE:Are you as genius as me? |
|
|
How 'bout you stop being a stuck up prick and ask a question without trying to allude to everyone that you're some kind of genius. I'm sorely tempted to lock this right now. |
|
|
|
|
 |
|