[Tutorial] View.Set and View.Update
Author |
Message |
Clayton

|
Posted: Tue May 30, 2006 8:55 pm Post subject: [Tutorial] View.Set and View.Update |
|
|
Well here goes, I saw the tutorial in the Turing Walkthrough for this and well... it didnt really explain much, so I'm going to try to give you a tutorial on what I know about View.Set and View.Update.
The Basics
View.Set (alternately known as setscreen, but for the purposes of this tutorial we will be using View.Set) is a command that allows you to play around with your output screen. It allows you to change the size of the screen, the position of the screen, and other goodies that I will show you.
To Start
To start with we need to know how to use View.Set. View.Set is called on its own line and can accept many different things, here is an example:
Turing: |
View.Set("graphics:500;300,position:center;center,nobuttonbar,title:View.Set tut")
|
now some of you are probably going, "Whoa, what the heck happened there?", well let me explain. When giving View.Set a parameter, anything that's passed is in quotations. Now lets take apart my line piece by piece:
1) View.Set : calls the Set procedure in the View module
2) ("") : the parameter passed to the Set procedure which is in quotes
3) ("graphics" : this is a screen "mode" by default the screen is set to "text" mode, in which the screen is measured by rows and columns of characters, whereas in "graphics" mode, the screen is measured in pixels and use a regular cartesian plane.
4):500;300 : this sets the screen size to being 500 pixels wide(x-axis) and 300 pixels high (y-axis) in this way we can control the size of our screen to whatever we want
5)"position:center;center" : this accesses your monitor size and places your run window in the center of the screen, you can also have
Turing: |
View.Set("graphics:500;300,position:0,0,nobuttonbar")
|
where the position of the bottom left corner of your run window would be in the bottom left-hand corner of the monitor
6)"nobuttonbar" : this is just one of a few things you can do to change the cursor, run window, and output. the following other commands can go into there as well if you so choose, seperated by a comma (,) from other commands:
nobuttonbar : this eliminates the "button bar" at the top of the run window (the one with stop, pause, print etc on it)
noecho : causes typed text not to show up on the screen
nocursor : causes the annoying Turing cursor to disappear, poof!
7)"title: View.Set tut") : changes the name on your run window from your program name run window to that of whatever follows the colon after title (eg. if your program's name is foo, the run window name would be foo run window unless changed with View.Set)
Using View.Set to eliminate Flickering
Now I've noticed a lot of people lately have taken to asking how to reduce the flickering in their programs. So I am going to do my best to explain how to stop the flickering.
To stop the flickering in programs Turing has a wonderful command called View.Update (there is also View.UpdateArea which we will go over later). This command is used in conjunction with View.Set (oooooh) and one of its commands: offscreenonly
But what is so special about offscreenonly you say? What it does is create an off screen buffer where all changes made to the screen are made there, and is drawn to the screen when View.Update is called. This is called double buffering. This reduces the flickering because all of the changes that you make are being made to the screen at once, so instead of seeing a clear screen for a split second then animation, then a clear screen for a split second... etc, you see all changes at once, resulting in a "smooth" animation
Here I have an example of using offscreenonly without View.Update and with View.Update (its just a ball bouncing)
without View.Update
Turing: |
View.Set ("graphics,offscreenonly")
var x, y, xChange, yChange : int := 1
const RADIUS : int := 5
Draw.Box (0, 0, maxx, maxy, black)
x := maxx div 2
y := maxy div 2
loop
Draw.Cls
Draw.Box (0, 0, maxx, maxy, black)
Draw.FillOval (x, y, RADIUS, RADIUS, green)
Time.Delay (5)
x + = xChange
y + = yChange
if View.WhatDotColor (x, y + RADIUS ) = black then
yChange * = - 1
elsif View.WhatDotColor (x, y - RADIUS ) = black then
yChange * = - 1
end if
if View.WhatDotColor (x + RADIUS, y ) = black then
xChange * = - 1
elsif View.WhatDotColor (x - RADIUS, y ) = black then
xChange * = - 1
end if
end loop
|
Copy and paste that code into Turing and you'll see nothing appears to happen, that's because offscreenonly mode is in effect and you are not drawing what was put to the off screen buffer using View.Update, to solve that problem just stick in a View.Update and... Voila! It should also be noted that as few View.Updates should be used as possible to avoid unnecessary slowdowns and multiple View.Updates can cause a problem
Turing: |
View.Set ("graphics,offscreenonly")
var x, y, xChange, yChange : int := 1
const RADIUS : int := 5
Draw.Box (0, 0, maxx, maxy, black)
x := maxx div 2
y := maxy div 2
loop
Draw.Cls
Draw.Box (0, 0, maxx, maxy, black)
Draw.FillOval (x, y, RADIUS, RADIUS, green)
View.Update
Time.Delay (5)
x + = xChange
y + = yChange
if View.WhatDotColor (x, y + RADIUS ) = black then
yChange * = - 1
elsif View.WhatDotColor (x, y - RADIUS ) = black then
yChange * = - 1
end if
if View.WhatDotColor (x + RADIUS, y ) = black then
xChange * = - 1
elsif View.WhatDotColor (x - RADIUS, y ) = black then
xChange * = - 1
end if
end loop
|
pop that into your editor and you'll notice a ball moving around... without flickering!! yay we've solved the problem of flickering.
now a program similiar to that but WITHOUT View.Update (dont watch if you're epileptic lol)
Turing: |
View.Set ("graphics")
var x, y, xChange, yChange : int := 1
const RADIUS : int := 5
Draw.Box (0, 0, maxx, maxy, black)
x := maxx div 2
y := maxy div 2
loop
Draw.Cls
Draw.Box (0, 0, maxx, maxy, black)
Draw.FillOval (x, y, RADIUS, RADIUS, green)
Time.Delay (5)
x + = xChange
y + = yChange
if View.WhatDotColor (x, y + RADIUS ) = black then
yChange * = - 1
elsif View.WhatDotColor (x, y - RADIUS ) = black then
yChange * = - 1
end if
if View.WhatDotColor (x + RADIUS, y ) = black then
xChange * = - 1
elsif View.WhatDotColor (x - RADIUS, y ) = black then
xChange * = - 1
end if
end loop
|
notice how flashy/flickery the screen is? that is because all changes are being made to the screen as they happen instead of all happening at once from the buffer
However, using View.Update has its drawbacks. It slows down your program IMMENSELY due to the fact that it has to update the entire screen from the buffer. The easiest way to solve this is to use a neat little command called...
View.UpdateArea
The only main difference from View.Update is that it only updates a specific area (specified by you). An example of its use is as follows:
As you can see View.UpdateArea requires the screen to be in offscreenonly mode as well, but it also requires four(4) parameters (x1,y1,x2,y2), these parameters tell the computer to update the area within these boundaries and thus it can speed up your program (as its not updating the entire screen like View.Update)
An example of View.UpdateArea in action (our ball program)
Turing: |
View.Set ("graphics,offscreenonly")
var x, y, xChange, yChange : int := 1
const RADIUS : int := 5
Draw.Box (0, 0, maxx, maxy, black)
x := maxx div 2
y := maxy div 2
loop
Draw.Cls
Draw.Box (0, 0, maxx, maxy, black)
Draw.FillOval (x, y, RADIUS, RADIUS, green)
View.UpdateArea (x - 10, y - 10, x + 10, y + 10)
Time.Delay (5)
x + = xChange
y + = yChange
if View.WhatDotColor (x, y + RADIUS ) = black then
yChange * = - 1
elsif View.WhatDotColor (x, y - RADIUS ) = black then
yChange * = - 1
end if
if View.WhatDotColor (x + RADIUS, y ) = black then
xChange * = - 1
elsif View.WhatDotColor (x - RADIUS, y ) = black then
xChange * = - 1
end if
end loop
|
as you can see the program runs faster now that the buffer isnt entirely being transferred to the screen.
Also, if for any reason you want to turn offscreenonly off, simply create another call to View.Set with nooffscreenonly in it to deactivate offscreen only. Another note is that, by default, your programs begin in nooffscreenonly mode.
noofscreenonly in action:
Turing: |
View.Set("offscreenonly")
%oops i dont want it on anymore
View.Set ("nooffscreenonly")
|
And with that I will end my Tutorial on View.Set and View.Update(Area) and leave you to try out this newfound idea hope this helps you animators out there!! If you have any questions plz post or PM me [/b] |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Tony
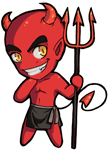
|
Posted: Tue May 30, 2006 9:18 pm Post subject: (No subject) |
|
|
This is a definite improvement
Perhaps it would have been better if you were to explain what double buffering is, how it works, and why does it eliminate the flickering. Also the examples of the same code - one flickering and one not, so that a clear improvement could be seen.
Though I still like it. +100Bits |
Tony's programming blog. DWITE - a programming contest. |
|
|
|
 |
Clayton

|
Posted: Tue May 30, 2006 9:27 pm Post subject: (No subject) |
|
|
Changes you requested have been made and thanks for the bits   |
|
|
|
|
 |
Shyfire

|
Posted: Thu Jun 01, 2006 7:46 am Post subject: (No subject) |
|
|
dude thank you i was gonna write a tutorial on this but im to lazy so....thx |
|
|
|
|
 |
rownale
|
Posted: Mon Jun 05, 2006 3:26 pm Post subject: (No subject) |
|
|
Thank for writing this
before i didnt no how to end the offscreenonly part
i didnt realize that it was as simple as nooffscreenonly lol
thanks |
|
|
|
|
 |
upthescale
|
Posted: Mon Jun 12, 2006 4:20 pm Post subject: (No subject) |
|
|
very nice superfreak, u said a lot, 10 bits:) |
|
|
|
|
 |
Mr. T

|
Posted: Mon Jun 12, 2006 11:21 pm Post subject: Alex's Opinion |
|
|
upthescale wrote: very nice superfreak, u said a lot, 10 bits:)
I'll take quality over quantity any day of the week.  |
|
|
|
|
 |
Foundation

|
Posted: Wed Sep 13, 2006 8:10 pm Post subject: (No subject) |
|
|
Thanks, it's a lot of help, but I still don't understand one part. How does nooffscreen work, I think that double buffering would actually cause flickering because it's transferring the images from the offscreen to onscreen frequently. And how often does View.Update update? Only when it is encountered in the code?" Thanks. |
|
|
|
|
 |
Sponsor Sponsor

|
|
 |
Clayton

|
Posted: Wed Sep 13, 2006 8:15 pm Post subject: (No subject) |
|
|
please post questions like these in [Turing Help], however, its here so ill answer it.
basically when the screen is in "offscreenonly" mode, all changes that would normally be made to the screen are made to an offscreen buffer, you will never see any of these changes unless you call the procedure that draws that buffer to the screen. The reason that this doesnt cause flickering is because when you are drawing a moving ball or whatever in nooffscreenonly mode, you see everything that happens as it happens on the screen. so in other words, you see the screen clear itself (turn white) then get drawn on, clear itself (turn white) and then be drawn on, this is what causes the flickering. however, in offscreenonly mode, you should never see the screen be cleared, because it is happening off the screen, then when you call View.Update, the offscreen buffer is drawn to the screen, eliminating the flicker. the buffer is only drawn to the screen when turing encounters the View.Update command in your code. |
|
|
|
|
 |
War_Caymore

|
Posted: Fri Sep 22, 2006 11:14 am Post subject: (No subject) |
|
|
i would like to thank freakman for making such an informational tutorial. jsut to let you know i'm reading every one of them beucase i'm a complete noob at turing. Because of your tutorial, i've learned valuable info that will allow me to actualy help fatboi and zero_paladn in the games that they will hopefully present to compsci.ca
keep up the good work.
P.S. i'd give out bits but... i'm nearly out. |
|
|
|
|
 |
GrimGreg
|
Posted: Mon Feb 19, 2007 7:48 pm Post subject: Re: [Tutorial] View.Set and View.Update |
|
|
Thanks man! great job. The explanation at the end, of why it did what it did really helped.. although i dont know where to put these lines into my game. good job anyways |
|
|
|
|
 |
GrimGreg
|
Posted: Mon Feb 19, 2007 7:49 pm Post subject: Re: [Tutorial] View.Set and View.Update |
|
|
Thanks man! great job. The explanation at the end, of why it did what it did really helped.. although i dont know where to put these lines into my game. good job anyways |
|
|
|
|
 |
Clayton

|
|
|
|
 |
evildaddy911
|
Posted: Mon Jan 16, 2012 8:55 am Post subject: RE:[Tutorial] View.Set and View.Update |
|
|
you should put View.Update right before you need it, and View.Update right before the cls, and try not to double post |
|
|
|
|
 |
SNIPERDUDE

|
Posted: Mon Jan 16, 2012 11:31 am Post subject: Re: RE:[Tutorial] View.Set and View.Update |
|
|
evildaddy911 @ January 16th 2012, 8:55 am wrote: and try not to double post
Try not to necro-post. |
|
|
|
|
 |
|
|